USB CDC library for MBED on STM32
Embed:
(wiki syntax)
Show/hide line numbers
usbd_cdc.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file usbd_cdc.c 00004 * @author MCD Application Team 00005 * @version V2.4.2 00006 * @date 11-December-2015 00007 * @brief This file provides the high layer firmware functions to manage the 00008 * following functionalities of the USB CDC Class: 00009 * - Initialization and Configuration of high and low layer 00010 * - Enumeration as CDC Device (and enumeration for each implemented memory interface) 00011 * - OUT/IN data transfer 00012 * - Command IN transfer (class requests management) 00013 * - Error management 00014 * 00015 * @verbatim 00016 * 00017 * =================================================================== 00018 * CDC Class Driver Description 00019 * =================================================================== 00020 * This driver manages the "Universal Serial Bus Class Definitions for Communications Devices 00021 * Revision 1.2 November 16, 2007" and the sub-protocol specification of "Universal Serial Bus 00022 * Communications Class Subclass Specification for PSTN Devices Revision 1.2 February 9, 2007" 00023 * This driver implements the following aspects of the specification: 00024 * - Device descriptor management 00025 * - Configuration descriptor management 00026 * - Enumeration as CDC device with 2 data endpoints (IN and OUT) and 1 command endpoint (IN) 00027 * - Requests management (as described in section 6.2 in specification) 00028 * - Abstract Control Model compliant 00029 * - Union Functional collection (using 1 IN endpoint for control) 00030 * - Data interface class 00031 * 00032 * These aspects may be enriched or modified for a specific user application. 00033 * 00034 * This driver doesn't implement the following aspects of the specification 00035 * (but it is possible to manage these features with some modifications on this driver): 00036 * - Any class-specific aspect relative to communication classes should be managed by user application. 00037 * - All communication classes other than PSTN are not managed 00038 * 00039 * @endverbatim 00040 * 00041 ****************************************************************************** 00042 * @attention 00043 * 00044 * <h2><center>© COPYRIGHT 2015 STMicroelectronics</center></h2> 00045 * 00046 * Licensed under MCD-ST Liberty SW License Agreement V2, (the "License"); 00047 * You may not use this file except in compliance with the License. 00048 * You may obtain a copy of the License at: 00049 * 00050 * http://www.st.com/software_license_agreement_liberty_v2 00051 * 00052 * Unless required by applicable law or agreed to in writing, software 00053 * distributed under the License is distributed on an "AS IS" BASIS, 00054 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00055 * See the License for the specific language governing permissions and 00056 * limitations under the License. 00057 * 00058 ****************************************************************************** 00059 */ 00060 00061 /* Includes ------------------------------------------------------------------*/ 00062 #include "usbd_cdc.h" 00063 #include "usbd_ctlreq.h" 00064 00065 /** @addtogroup STM32_USB_DEVICE_LIBRARY 00066 * @{ 00067 */ 00068 00069 /** @defgroup USBD_CDC 00070 * @brief usbd core module 00071 * @{ 00072 */ 00073 00074 /** @defgroup USBD_CDC_Private_TypesDefinitions 00075 * @{ 00076 */ 00077 /** 00078 * @} 00079 */ 00080 00081 /** @defgroup USBD_CDC_Private_Defines 00082 * @{ 00083 */ 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup USBD_CDC_Private_Macros 00089 * @{ 00090 */ 00091 00092 /** 00093 * @} 00094 */ 00095 00096 /** @defgroup USBD_CDC_Private_FunctionPrototypes 00097 * @{ 00098 */ 00099 00100 static uint8_t USBD_CDC_Init(USBD_HandleTypeDef *pdev, uint8_t cfgidx); 00101 00102 static uint8_t USBD_CDC_DeInit(USBD_HandleTypeDef *pdev, uint8_t cfgidx); 00103 00104 static uint8_t USBD_CDC_Setup(USBD_HandleTypeDef *pdev, 00105 USBD_SetupReqTypedef *req); 00106 00107 static uint8_t USBD_CDC_DataIn(USBD_HandleTypeDef *pdev, uint8_t epnum); 00108 00109 static uint8_t USBD_CDC_DataOut(USBD_HandleTypeDef *pdev, uint8_t epnum); 00110 00111 static uint8_t USBD_CDC_EP0_RxReady(USBD_HandleTypeDef *pdev); 00112 00113 static uint8_t *USBD_CDC_GetFSCfgDesc(uint16_t *length); 00114 00115 static uint8_t *USBD_CDC_GetHSCfgDesc(uint16_t *length); 00116 00117 static uint8_t *USBD_CDC_GetOtherSpeedCfgDesc(uint16_t *length); 00118 00119 static uint8_t *USBD_CDC_GetOtherSpeedCfgDesc(uint16_t *length); 00120 00121 uint8_t *USBD_CDC_GetDeviceQualifierDescriptor(uint16_t *length); 00122 00123 /* USB Standard Device Descriptor */ 00124 __ALIGN_BEGIN static uint8_t USBD_CDC_DeviceQualifierDesc[USB_LEN_DEV_QUALIFIER_DESC] __ALIGN_END 00125 = 00126 { 00127 USB_LEN_DEV_QUALIFIER_DESC, 00128 USB_DESC_TYPE_DEVICE_QUALIFIER, 0x00, 0x02, 0x00, 0x00, 0x00, 0x40, 00129 0x01, 0x00, }; 00130 00131 /** 00132 * @} 00133 */ 00134 00135 /** @defgroup USBD_CDC_Private_Variables 00136 * @{ 00137 */ 00138 00139 /* CDC interface class callbacks structure */ 00140 USBD_ClassTypeDef USBD_CDC = 00141 { USBD_CDC_Init, USBD_CDC_DeInit, USBD_CDC_Setup, 00142 NULL, /* EP0_TxSent, */ 00143 USBD_CDC_EP0_RxReady, USBD_CDC_DataIn, USBD_CDC_DataOut, 00144 NULL, 00145 NULL, 00146 NULL, USBD_CDC_GetHSCfgDesc, USBD_CDC_GetFSCfgDesc, 00147 USBD_CDC_GetOtherSpeedCfgDesc, USBD_CDC_GetDeviceQualifierDescriptor, }; 00148 00149 /* USB CDC device Configuration Descriptor */ 00150 __ALIGN_BEGIN uint8_t USBD_CDC_CfgDesc[USB_CDC_CONFIG_DESC_SIZ] __ALIGN_END = 00151 { 00152 00153 // 00154 /*Configuration Descriptor*/ 00155 0x09, /* bLength: Configuration Descriptor size */ 00156 USB_DESC_TYPE_CONFIGURATION, /* bDescriptorType: Configuration */ 00157 USB_CDC_CONFIG_DESC_SIZ, /* wTotalLength:no of returned bytes */ 00158 0x00, 0x02, /* bNumInterfaces: 2 interface */ 00159 0x01, /* bConfigurationValue: Configuration value */ 00160 0x00, /* iConfiguration: Index of string descriptor describing the configuration */ 00161 0xC0, /* bmAttributes: self powered */ 00162 0x32, /* MaxPower 0 mA */ 00163 00164 /*---------------------------------------------------------------------------*/ 00165 // IAD to associate the two CDC interfaces 00166 0x08,// bLength 00167 0x0b, // bDescriptorType 00168 0x00, // bFirstInterface 00169 0x02, // bInterfaceCount 00170 0x02, // bFunctionClass 00171 0x02, // bFunctionSubClass 00172 0, // bFunctionProtocol 00173 0, // iFunction 00174 00175 /*Interface Descriptor */ 00176 0x09, /* bLength: Interface Descriptor size */ 00177 USB_DESC_TYPE_INTERFACE, /* bDescriptorType: Interface */ 00178 /* Interface descriptor type */ 00179 0x00, /* bInterfaceNumber: Number of Interface */ 00180 0x00, /* bAlternateSetting: Alternate setting */ 00181 0x01, /* bNumEndpoints: One endpoints used */ 00182 0x02, /* bInterfaceClass: Communication Interface Class */ 00183 0x02, /* bInterfaceSubClass: Abstract Control Model */ 00184 0x01, /* bInterfaceProtocol: Common AT commands */ 00185 0x00, /* iInterface: */ 00186 00187 /*Header Functional Descriptor*/ 00188 0x05, /* bLength: Endpoint Descriptor size */ 00189 0x24, /* bDescriptorType: CS_INTERFACE */ 00190 0x00, /* bDescriptorSubtype: Header Func Desc */ 00191 0x10, /* bcdCDC: spec release number */ 00192 0x01, 00193 00194 /*Call Management Functional Descriptor*/ 00195 0x05, /* bFunctionLength */ 00196 0x24, /* bDescriptorType: CS_INTERFACE */ 00197 0x01, /* bDescriptorSubtype: Call Management Func Desc */ 00198 0x03, /* bmCapabilities: D0+D1 */ 00199 0x01, /* bDataInterface: 1 */ 00200 00201 /*ACM Functional Descriptor*/ 00202 0x04, /* bFunctionLength */ 00203 0x24, /* bDescriptorType: CS_INTERFACE */ 00204 0x02, /* bDescriptorSubtype: Abstract Control Management desc */ 00205 0x02, /* bmCapabilities */ 00206 00207 /*Union Functional Descriptor*/ 00208 0x05, /* bFunctionLength */ 00209 0x24, /* bDescriptorType: CS_INTERFACE */ 00210 0x06, /* bDescriptorSubtype: Union func desc */ 00211 0x00, /* bMasterInterface: Communication class interface */ 00212 0x01, /* bSlaveInterface0: Data Class Interface */ 00213 00214 /*Endpoint 2 Descriptor*/ 00215 0x07, /* bLength: Endpoint Descriptor size */ 00216 USB_DESC_TYPE_ENDPOINT, /* bDescriptorType: Endpoint */ 00217 CDC_CMD_EP, /* bEndpointAddress */ 00218 0x03, /* bmAttributes: Interrupt */ 00219 LOBYTE(CDC_CMD_PACKET_SIZE), /* wMaxPacketSize: */ 00220 HIBYTE(CDC_CMD_PACKET_SIZE), 0x10, /* bInterval: */ 00221 /*---------------------------------------------------------------------------*/ 00222 00223 /*Data class interface descriptor*/ 00224 0x09, /* bLength: Endpoint Descriptor size */ 00225 USB_DESC_TYPE_INTERFACE, /* bDescriptorType: */ 00226 0x01, /* bInterfaceNumber: Number of Interface */ 00227 0x00, /* bAlternateSetting: Alternate setting */ 00228 0x02, /* bNumEndpoints: Two endpoints used */ 00229 0x0A, /* bInterfaceClass: CDC */ 00230 0x00, /* bInterfaceSubClass: */ 00231 0x00, /* bInterfaceProtocol: */ 00232 0x00, /* iInterface: */ 00233 00234 /*Endpoint OUT Descriptor*/ 00235 0x07, /* bLength: Endpoint Descriptor size */ 00236 USB_DESC_TYPE_ENDPOINT, /* bDescriptorType: Endpoint */ 00237 CDC_OUT_EP, /* bEndpointAddress */ 00238 0x02, /* bmAttributes: Bulk */ 00239 LOBYTE(CDC_DATA_FS_MAX_PACKET_SIZE), /* wMaxPacketSize: */ 00240 HIBYTE(CDC_DATA_FS_MAX_PACKET_SIZE), 0x00, /* bInterval: ignore for Bulk transfer */ 00241 00242 /*Endpoint IN Descriptor*/ 00243 0x07, /* bLength: Endpoint Descriptor size */ 00244 USB_DESC_TYPE_ENDPOINT, /* bDescriptorType: Endpoint */ 00245 CDC_IN_EP, /* bEndpointAddress */ 00246 0x02, /* bmAttributes: Bulk */ 00247 LOBYTE(CDC_DATA_FS_MAX_PACKET_SIZE), /* wMaxPacketSize: */ 00248 HIBYTE(CDC_DATA_FS_MAX_PACKET_SIZE), 0x00 /* bInterval: ignore for Bulk transfer */ 00249 }; 00250 00251 /** 00252 * @} 00253 */ 00254 00255 /** @defgroup USBD_CDC_Private_Functions 00256 * @{ 00257 */ 00258 00259 static USBD_CDC_HandleTypeDef usbd_cdc; 00260 00261 /** 00262 * @brief USBD_CDC_Init 00263 * Initialize the CDC interface 00264 * @param pdev: device instance 00265 * @param cfgidx: Configuration index 00266 * @retval status 00267 */ 00268 static uint8_t USBD_CDC_Init(USBD_HandleTypeDef *pdev, uint8_t cfgidx) 00269 { 00270 uint8_t ret = 0; 00271 USBD_CDC_HandleTypeDef *hcdc; 00272 00273 if (pdev->dev_speed == USBD_SPEED_HIGH) 00274 { 00275 /* Open EP IN */ 00276 USBD_LL_OpenEP(pdev, 00277 CDC_IN_EP, 00278 USBD_EP_TYPE_BULK, 00279 CDC_DATA_HS_IN_PACKET_SIZE); 00280 00281 /* Open EP OUT */ 00282 USBD_LL_OpenEP(pdev, 00283 CDC_OUT_EP, 00284 USBD_EP_TYPE_BULK, 00285 CDC_DATA_HS_OUT_PACKET_SIZE); 00286 00287 } 00288 else 00289 { 00290 /* Open EP IN */ 00291 USBD_LL_OpenEP(pdev, 00292 CDC_IN_EP, 00293 USBD_EP_TYPE_BULK, 00294 CDC_DATA_FS_IN_PACKET_SIZE); 00295 00296 /* Open EP OUT */ 00297 USBD_LL_OpenEP(pdev, 00298 CDC_OUT_EP, 00299 USBD_EP_TYPE_BULK, 00300 CDC_DATA_FS_OUT_PACKET_SIZE); 00301 } 00302 /* Open Command IN EP */ 00303 USBD_LL_OpenEP(pdev, 00304 CDC_CMD_EP, 00305 USBD_EP_TYPE_INTR, 00306 CDC_CMD_PACKET_SIZE); 00307 00308 // pdev->pClassData = USBD_malloc(sizeof (USBD_CDC_HandleTypeDef)); 00309 pdev->pClassData = &usbd_cdc; 00310 00311 if (pdev->pClassData == NULL) 00312 { 00313 ret = 1; 00314 } 00315 else 00316 { 00317 hcdc = (USBD_CDC_HandleTypeDef*) pdev->pClassData; 00318 00319 /* Init physical Interface components */ 00320 ((USBD_CDC_ItfTypeDef *) pdev->pUserData)->Init(); 00321 00322 /* Init Xfer states */ 00323 hcdc->TxState = 0; 00324 hcdc->RxState = 0; 00325 00326 if (pdev->dev_speed == USBD_SPEED_HIGH) 00327 { 00328 /* Prepare Out endpoint to receive next packet */ 00329 USBD_LL_PrepareReceive(pdev, 00330 CDC_OUT_EP, hcdc->RxBuffer, 00331 CDC_DATA_HS_OUT_PACKET_SIZE); 00332 } 00333 else 00334 { 00335 /* Prepare Out endpoint to receive next packet */ 00336 USBD_LL_PrepareReceive(pdev, 00337 CDC_OUT_EP, hcdc->RxBuffer, 00338 CDC_DATA_FS_OUT_PACKET_SIZE); 00339 } 00340 00341 } 00342 return ret; 00343 } 00344 00345 /** 00346 * @brief USBD_CDC_Init 00347 * DeInitialize the CDC layer 00348 * @param pdev: device instance 00349 * @param cfgidx: Configuration index 00350 * @retval status 00351 */ 00352 static uint8_t USBD_CDC_DeInit(USBD_HandleTypeDef *pdev, uint8_t cfgidx) 00353 { 00354 uint8_t ret = 0; 00355 00356 /* Open EP IN */ 00357 USBD_LL_CloseEP(pdev, 00358 CDC_IN_EP); 00359 00360 /* Open EP OUT */ 00361 USBD_LL_CloseEP(pdev, 00362 CDC_OUT_EP); 00363 00364 /* Open Command IN EP */ 00365 USBD_LL_CloseEP(pdev, 00366 CDC_CMD_EP); 00367 00368 /* DeInit physical Interface components */ 00369 if (pdev->pClassData != NULL) 00370 { 00371 ((USBD_CDC_ItfTypeDef *) pdev->pUserData)->DeInit(); 00372 //USBD_free(pdev->pClassData); 00373 pdev->pClassData = NULL; 00374 } 00375 00376 return ret; 00377 } 00378 00379 /** 00380 * @brief USBD_CDC_Setup 00381 * Handle the CDC specific requests 00382 * @param pdev: instance 00383 * @param req: usb requests 00384 * @retval status 00385 */ 00386 static uint8_t USBD_CDC_Setup(USBD_HandleTypeDef *pdev, 00387 USBD_SetupReqTypedef *req) 00388 { 00389 USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef*) pdev->pClassData; 00390 static uint8_t ifalt = 0; 00391 00392 switch (req->bmRequest & USB_REQ_TYPE_MASK) 00393 { 00394 case USB_REQ_TYPE_CLASS: 00395 if (req->wLength) 00396 { 00397 if (req->bmRequest & 0x80) 00398 { 00399 ((USBD_CDC_ItfTypeDef *) pdev->pUserData)->Control( 00400 req->bRequest, (uint8_t *) hcdc->data, req->wLength); 00401 USBD_CtlSendData(pdev, (uint8_t *) hcdc->data, req->wLength); 00402 } 00403 else 00404 { 00405 hcdc->CmdOpCode = req->bRequest; 00406 hcdc->CmdLength = req->wLength; 00407 00408 USBD_CtlPrepareRx(pdev, (uint8_t *) hcdc->data, req->wLength); 00409 } 00410 00411 } 00412 else 00413 { 00414 ((USBD_CDC_ItfTypeDef *) pdev->pUserData)->Control(req->bRequest, 00415 (uint8_t*) req, 0); 00416 } 00417 break; 00418 00419 case USB_REQ_TYPE_STANDARD: 00420 switch (req->bRequest) 00421 { 00422 case USB_REQ_GET_INTERFACE: 00423 USBD_CtlSendData(pdev, &ifalt, 1); 00424 break; 00425 00426 case USB_REQ_SET_INTERFACE: 00427 break; 00428 } 00429 break; 00430 00431 default: 00432 break; 00433 } 00434 return USBD_OK; 00435 } 00436 00437 /** 00438 * @brief USBD_CDC_DataIn 00439 * Data sent on non-control IN endpoint 00440 * @param pdev: device instance 00441 * @param epnum: endpoint number 00442 * @retval status 00443 */ 00444 static uint8_t USBD_CDC_DataIn(USBD_HandleTypeDef *pdev, uint8_t epnum) 00445 { 00446 USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef*) pdev->pClassData; 00447 00448 if (pdev->pClassData != NULL) 00449 { 00450 00451 hcdc->TxState = 0; 00452 00453 ((USBD_CDC_ItfTypeDef *) pdev->pUserData)->Transmitted(hcdc->TxBuffer, 00454 hcdc->TxLength); 00455 00456 return USBD_OK; 00457 } 00458 else 00459 { 00460 return USBD_FAIL; 00461 } 00462 } 00463 00464 /** 00465 * @brief USBD_CDC_DataOut 00466 * Data received on non-control Out endpoint 00467 * @param pdev: device instance 00468 * @param epnum: endpoint number 00469 * @retval status 00470 */ 00471 static uint8_t USBD_CDC_DataOut(USBD_HandleTypeDef *pdev, uint8_t epnum) 00472 { 00473 USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef*) pdev->pClassData; 00474 00475 /* Get the received data length */ 00476 hcdc->RxLength = USBD_LL_GetRxDataSize(pdev, epnum); 00477 hcdc->RxState = 0; 00478 00479 /* USB data will be immediately processed, this allow next USB traffic being 00480 NAKed till the end of the application Xfer */ 00481 if (pdev->pClassData != NULL) 00482 { 00483 ((USBD_CDC_ItfTypeDef *) pdev->pUserData)->Received(hcdc->RxBuffer, 00484 &hcdc->RxLength); 00485 00486 return USBD_OK; 00487 } 00488 else 00489 { 00490 return USBD_FAIL; 00491 } 00492 } 00493 00494 /** 00495 * @brief USBD_CDC_DataOut 00496 * Data received on non-control Out endpoint 00497 * @param pdev: device instance 00498 * @param epnum: endpoint number 00499 * @retval status 00500 */ 00501 static uint8_t USBD_CDC_EP0_RxReady(USBD_HandleTypeDef *pdev) 00502 { 00503 USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef*) pdev->pClassData; 00504 00505 if ((pdev->pUserData != NULL) && (hcdc->CmdOpCode != 0xFF)) 00506 { 00507 ((USBD_CDC_ItfTypeDef *) pdev->pUserData)->Control(hcdc->CmdOpCode, 00508 (uint8_t *) hcdc->data, hcdc->CmdLength); 00509 hcdc->CmdOpCode = 0xFF; 00510 00511 } 00512 return USBD_OK; 00513 } 00514 00515 /** 00516 * @brief USBD_CDC_GetFSCfgDesc 00517 * Return configuration descriptor 00518 * @param speed : current device speed 00519 * @param length : pointer data length 00520 * @retval pointer to descriptor buffer 00521 */ 00522 static uint8_t *USBD_CDC_GetFSCfgDesc(uint16_t *length) 00523 { 00524 *length = USB_CDC_CONFIG_DESC_SIZ; 00525 return USBD_CDC_CfgDesc; 00526 } 00527 00528 /** 00529 * @brief USBD_CDC_GetHSCfgDesc 00530 * Return configuration descriptor 00531 * @param speed : current device speed 00532 * @param length : pointer data length 00533 * @retval pointer to descriptor buffer 00534 */ 00535 static uint8_t *USBD_CDC_GetHSCfgDesc(uint16_t *length) 00536 { 00537 *length = USB_CDC_CONFIG_DESC_SIZ; 00538 return USBD_CDC_CfgDesc; 00539 } 00540 00541 /** 00542 * @brief USBD_CDC_GetCfgDesc 00543 * Return configuration descriptor 00544 * @param speed : current device speed 00545 * @param length : pointer data length 00546 * @retval pointer to descriptor buffer 00547 */ 00548 static uint8_t *USBD_CDC_GetOtherSpeedCfgDesc(uint16_t *length) 00549 { 00550 *length = USB_CDC_CONFIG_DESC_SIZ; 00551 return USBD_CDC_CfgDesc; 00552 } 00553 00554 /** 00555 * @brief DeviceQualifierDescriptor 00556 * return Device Qualifier descriptor 00557 * @param length : pointer data length 00558 * @retval pointer to descriptor buffer 00559 */ 00560 uint8_t *USBD_CDC_GetDeviceQualifierDescriptor(uint16_t *length) 00561 { 00562 *length = sizeof(USBD_CDC_DeviceQualifierDesc); 00563 return USBD_CDC_DeviceQualifierDesc; 00564 } 00565 00566 /** 00567 * @brief USBD_CDC_RegisterInterface 00568 * @param pdev: device instance 00569 * @param fops: CD Interface callback 00570 * @retval status 00571 */ 00572 uint8_t USBD_CDC_RegisterInterface(USBD_HandleTypeDef *pdev, 00573 USBD_CDC_ItfTypeDef *fops) 00574 { 00575 uint8_t ret = USBD_FAIL; 00576 00577 if (fops != NULL) 00578 { 00579 pdev->pUserData = fops; 00580 ret = USBD_OK; 00581 } 00582 00583 return ret; 00584 } 00585 00586 /** 00587 * @brief USBD_CDC_SetTxBuffer 00588 * @param pdev: device instance 00589 * @param pbuff: Tx Buffer 00590 * @retval status 00591 */ 00592 uint8_t USBD_CDC_SetTxBuffer(USBD_HandleTypeDef *pdev, uint8_t *pbuff, 00593 uint16_t length) 00594 { 00595 USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef*) pdev->pClassData; 00596 00597 hcdc->TxBuffer = pbuff; 00598 hcdc->TxLength = length; 00599 00600 return USBD_OK; 00601 } 00602 00603 /** 00604 * @brief USBD_CDC_SetRxBuffer 00605 * @param pdev: device instance 00606 * @param pbuff: Rx Buffer 00607 * @retval status 00608 */ 00609 uint8_t USBD_CDC_SetRxBuffer(USBD_HandleTypeDef *pdev, uint8_t *pbuff) 00610 { 00611 USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef*) pdev->pClassData; 00612 00613 hcdc->RxBuffer = pbuff; 00614 00615 return USBD_OK; 00616 } 00617 00618 /** 00619 * @brief USBD_CDC_DataOut 00620 * Data received on non-control Out endpoint 00621 * @param pdev: device instance 00622 * @param epnum: endpoint number 00623 * @retval status 00624 */ 00625 uint8_t USBD_CDC_TransmitPacket(USBD_HandleTypeDef *pdev) 00626 { 00627 USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef*) pdev->pClassData; 00628 00629 if (pdev->pClassData != NULL) 00630 { 00631 if (hcdc->TxState == 0) 00632 { 00633 /* Tx Transfer in progress */ 00634 hcdc->TxState = 1; 00635 00636 /* Transmit next packet */ 00637 USBD_LL_Transmit(pdev, 00638 CDC_IN_EP, hcdc->TxBuffer, hcdc->TxLength); 00639 00640 return USBD_OK; 00641 } 00642 else 00643 { 00644 return USBD_BUSY; 00645 } 00646 } 00647 else 00648 { 00649 return USBD_FAIL; 00650 } 00651 } 00652 00653 /** 00654 * @brief USBD_CDC_ReceivePacket 00655 * prepare OUT Endpoint for reception 00656 * @param pdev: device instance 00657 * @retval status 00658 */ 00659 uint8_t USBD_CDC_ReceivePacket(USBD_HandleTypeDef *pdev) 00660 { 00661 USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef*) pdev->pClassData; 00662 00663 /* Suspend or Resume USB Out process */ 00664 if (pdev->pClassData != NULL) 00665 { 00666 hcdc->RxState = 1; 00667 if (pdev->dev_speed == USBD_SPEED_HIGH) 00668 { 00669 /* Prepare Out endpoint to receive next packet */ 00670 USBD_LL_PrepareReceive(pdev, 00671 CDC_OUT_EP, hcdc->RxBuffer, 00672 CDC_DATA_HS_OUT_PACKET_SIZE); 00673 } 00674 else 00675 { 00676 /* Prepare Out endpoint to receive next packet */ 00677 USBD_LL_PrepareReceive(pdev, 00678 CDC_OUT_EP, hcdc->RxBuffer, 00679 CDC_DATA_FS_OUT_PACKET_SIZE); 00680 } 00681 return USBD_OK; 00682 } 00683 else 00684 { 00685 return USBD_FAIL; 00686 } 00687 } 00688 /** 00689 * @} 00690 */ 00691 00692 /** 00693 * @} 00694 */ 00695 00696 /** 00697 * @} 00698 */ 00699 00700 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00701
Generated on Thu Jul 14 2022 15:47:45 by
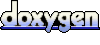