Simple RPG Library
Dependents: Audio_Player Wav_player_RPG_menu lcd_menu Lab3 ... more
RPG.cpp
00001 /** 00002 * ============================================================================= 00003 * Rotary Pulse Generator class (Version 0.0.1) 00004 * ============================================================================= 00005 * Copyright (c) 2012 Christopher Anderson 00006 * 00007 * Permission is hereby granted, free of charge, to any person obtaining a copy 00008 * of this software and associated documentation files (the "Software"), to deal 00009 * in the Software without restriction, including without limitation the rights 00010 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00011 * copies of the Software, and to permit persons to whom the Software is 00012 * furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included in 00015 * all copies or substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00020 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00022 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00023 * THE SOFTWARE. 00024 * ============================================================================= 00025 */ 00026 00027 #include "RPG.h" 00028 #include "mbed.h" 00029 00030 /** 00031 * Constructor(Channel A input pin, Channel B input pin, Pushbutton input pin) 00032 */ 00033 RPG::RPG(PinName pA, PinName pB, PinName pPB) 00034 : chRPG(pA, pB), PB(pPB) 00035 { 00036 chRPG.input(); 00037 chRPG.mode(PullUp); 00038 PB.mode(PullUp); 00039 wait_us(10); 00040 RPGO = chRPG; 00041 } 00042 00043 /** 00044 *Destructor 00045 */ 00046 RPG::~RPG() 00047 {} 00048 00049 /** 00050 *reads and debounces push button returns bool result 00051 */ 00052 bool RPG::pb() 00053 { 00054 int check = PB; 00055 wait_us(5); 00056 if((!check) && !PB) 00057 { 00058 return true; 00059 } 00060 else return false; 00061 } 00062 00063 /** 00064 *Determines direction of rotation returns: 00065 *1 for clockwise 00066 *-1 for counter-clockwise 00067 *0 for no rotation 00068 */ 00069 int RPG::dir() 00070 { 00071 int dir = 0; 00072 RPGO = chRPG; 00073 wait(.005); 00074 RPGN = chRPG; 00075 if(RPGN != RPGO) 00076 { 00077 if((RPGN & 1) != (RPGO >> 1)) dir = 1; 00078 else dir = -1; 00079 } 00080 else dir = 0; 00081 RPGO = RPGN; 00082 return dir; 00083 }
Generated on Tue Jul 12 2022 17:54:54 by
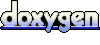