DSHOT150 algorithm using digital IO pins on the LPC-1768
Embed:
(wiki syntax)
Show/hide line numbers
DSHOT150.h
00001 /* 00002 Copyright (c) 2019 Blake West, Kevin Tseng, Tyler Brown, Mason Totri 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 #ifndef MBED_DSHOT15_H 00023 #define MBED_DSHOT15_H 00024 #include "mbed.h" 00025 /** DSHOT150 converts a digital IO pin to act like a DSHOT pulse 00026 * This is done through writing one's and zero's a specific amount 00027 * to acheive the correct pulse width in the given duty cycle. 00028 * 00029 * 00030 * Example 00031 * @code 00032 * #include "mbed.h" 00033 * #include "DSHOT150.h" 00034 * 00035 * DSHOT150 motor( p21 ); 00036 * 00037 * int main() { 00038 * 00039 * motor.arm(); 00040 * motor.get_tel( true ); 00041 * for( float i = 0; i < 1; i+=0.1){ 00042 * motor.throttle( i ); 00043 * } 00044 * 00045 * 00046 * } 00047 * @endcode 00048 * 00049 * This example will step the motor from 0 to 100% in 10% intervals 00050 * 00051 */ 00052 class DSHOT150 00053 { 00054 public: 00055 DSHOT150(PinName pin); //Constructor that takes in 00056 void throttle(float speed); //Throttle value as a percentage [0,1] 00057 void get_tel(bool v); //Telemetry request function. Set to true if wanting to receive telemetry from the ESC 00058 void arm(); //Arming comand for the motor 00059 00060 private: 00061 void send_packet(); //Sends the packet 00062 void check_sum(unsigned int v); //Calculates the check sum, and inserts it into the packet 00063 void write_zero(); 00064 void write_one(); 00065 bool packet[16]; //Packet of data that is being sent 00066 DigitalOut _pin; //Pin that is being used. 00067 unsigned int tel; 00068 }; 00069 #endif
Generated on Mon Aug 8 2022 12:24:01 by
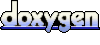