
it works!
Dependencies: SDFileSystem2 mbed
Fork of manworm_tv_raster by
main.cpp
00001 #include "mbed.h" 00002 #include <math.h> 00003 #include "main.h" 00004 #include "SDFileSystem.h" 00005 00006 #define TEXT_LEVEL 5 00007 00008 #define TX 24 // number of characters in X 00009 #define TY 10 // number of characters in Y 00010 00011 #define CHECK_BIT(var,pos) ((var) & (1<<(pos))) 00012 #define MIN(a,b) (((a)<(b))?(a):(b)) 00013 #define MAX(a,b) (((a)>(b))?(a):(b)) 00014 00015 00016 // DAC stuff 00017 #define DAC_SYNC 2 00018 00019 uint8_t char_col = 0; // current column counter 00020 uint8_t char_row = 0; // current row counter 00021 00022 Serial pc(USBTX, USBRX); 00023 00024 #include "vincent_data.h" 00025 00026 //DigitalOut sout(D8); //sync PA_9 00027 //DigitalOut vout(D7); //video PA_8 00028 00029 DigitalOut dac0(PA_4); 00030 DigitalOut dac1(PA_5); 00031 DigitalOut dac2(PA_6); 00032 DigitalOut dac3(PA_7); 00033 00034 DigitalOut user_led(PB_14); 00035 int led_state = 1; 00036 00037 // trigger horizontal line draw 00038 Ticker t; 00039 00040 uint8_t bl_line_s[H_RES]; //blank line sync buffer 00041 uint8_t bl_line_v[H_RES]; //blank line video buffer 00042 uint8_t vb_line_s[H_RES]; //vertical sync, sync buffer 00043 uint8_t vb_line_v[H_RES]; //vertical sync, video buffer 00044 uint8_t im_line_s[H_RES]; //image sync buffer 00045 00046 //buffers 00047 uint8_t current_frame[YL][XL / 2]; 00048 uint8_t im_line_va_1[H_RES*V_RES]; 00049 uint8_t im_line_va_2[H_RES*V_RES]; 00050 00051 //double buffered drawing 00052 uint8_t* im_line_va; 00053 uint8_t* im_back; 00054 00055 uint16_t l=0; //current line of scan 00056 uint32_t tics = 0; //timer 00057 00058 // positive or negative? 00059 int16_t sign(int16_t a) 00060 { 00061 if(a > 0) return 1; 00062 if(a < 0) return -1; 00063 return 0; 00064 } 00065 00066 // clear the screen and the text buffer 00067 void clr() 00068 { 00069 for(int i = 0; i < H_RES; i++) 00070 for(int j = 0; j < V_RES; j++) 00071 im_line_va[i+j*H_RES] = 0; 00072 } 00073 00074 // initialize video buffers 00075 void init_buffers() 00076 { 00077 clr(); // zero buffers 00078 for(int i = 0; i < H_RES; i++) 00079 { 00080 im_line_s[i] = DAC_SYNC; 00081 bl_line_s[i] = DAC_SYNC; 00082 bl_line_v[i] = 0; 00083 vb_line_s[i] = 0; 00084 vb_line_v[i] = 0; 00085 } 00086 im_line_s[0] = 0; 00087 im_line_s[1] = 0; 00088 im_line_s[2] = 0; 00089 im_line_s[3] = 0; 00090 00091 for(int i = 0; i < 15; i++) im_line_s[i] = 0; 00092 00093 bl_line_s[0] = 0; 00094 vb_line_s[0] = DAC_SYNC; 00095 bl_line_s[1] = 0; 00096 vb_line_s[1] = DAC_SYNC; 00097 00098 bl_line_s[3] = 0; 00099 vb_line_s[3] = DAC_SYNC; 00100 bl_line_s[2] = 0; 00101 vb_line_s[2] = DAC_SYNC; 00102 } 00103 00104 // video interrupt 00105 void isr() 00106 { 00107 uint8_t nop = 0, img = 0; //use nops or use wait_us 00108 uint8_t* sptr; //pointer to sync buffer for line 00109 uint8_t* vptr; //pointer to video buffer for line 00110 uint8_t* pptr; //porch 00111 00112 if (l < V_PORCH_SIZE) { 00113 vptr = bl_line_v; 00114 sptr = im_line_s; 00115 nop = 1; 00116 } 00117 else if (l < YL + V_PORCH_SIZE) { 00118 vptr = im_line_va + (l - 30) * H_RES; 00119 sptr = im_line_s; 00120 nop = 1; 00121 img = 1; 00122 } 00123 else if (l < 254) { 00124 vptr = bl_line_v; 00125 sptr = bl_line_s; 00126 nop = 0; 00127 } 00128 else { 00129 vptr = vb_line_v; 00130 sptr = vb_line_s; 00131 nop = 1; 00132 } 00133 00134 pptr = img ? bl_line_v : vptr; 00135 00136 if (nop) { 00137 for (uint16_t i = 0; i < H_PORCH_SIZE; i++) { 00138 GPIOA->ODR = (pptr[i] + sptr[i]) << 4; 00139 00140 asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop"); 00141 asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop"); 00142 } 00143 00144 for (uint16_t i = 0; i < H_RES; i++) //loop over each column 00145 { 00146 GPIOA->ODR = (vptr[i] + sptr[i]) << 4; 00147 00148 asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop"); 00149 asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop"); 00150 } 00151 } else { 00152 for(uint16_t i = 0; i < 12; i++) //loop over each column 00153 { 00154 GPIOA->ODR = sptr[i] << 4; 00155 00156 asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop"); 00157 asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop");asm("nop"); 00158 asm("nop");asm("nop");asm("nop");asm("nop"); 00159 } 00160 } 00161 00162 //move to next line 00163 l++; 00164 tics++; 00165 if(l > 255) l = 0; 00166 } 00167 00168 // draw letter 00169 void draw_vincent(uint16_t x0, uint16_t y0, uint8_t c) 00170 { 00171 if(c == 40) c = 41; 00172 else if(c == 41) c = 40; 00173 char* letter = vincent_data[c]; 00174 for(uint16_t xp = 0; xp < 8; xp++) 00175 { 00176 for(uint16_t yp = 0; yp < 8; yp++) 00177 { 00178 im_line_va[H_RES*(yp+y0) + xp + x0] = CHECK_BIT(letter[yp],8-xp)?TEXT_LEVEL:0; 00179 } 00180 } 00181 } 00182 00183 // draw string 00184 void draw_vincent_string(char* str) 00185 { 00186 for(int i = 0; i < strlen(str); i++) 00187 { 00188 if(str[i] == 0) return; 00189 char_col++; 00190 if(char_col >= TX) 00191 { 00192 char_col = 0; 00193 char_row++; 00194 } 00195 if(char_row >= TY) 00196 { 00197 char_row = 0; 00198 } 00199 00200 draw_vincent(X0 +2 + 8*char_col, Y0 + 14 + 8*char_row, 00201 str[i]); 00202 } 00203 } 00204 00205 void set_status_string(char* str) 00206 { 00207 uint8_t char_col_backup = char_col; 00208 uint8_t char_row_backup = char_row; 00209 char_col = 0; 00210 char_row = TY - 1; 00211 00212 draw_vincent_string(str); 00213 00214 char_col = char_col_backup; 00215 char_row = char_row_backup; 00216 } 00217 00218 int main() 00219 { 00220 uint8_t *buf_swap_tmp; 00221 im_line_va = im_line_va_1; 00222 im_back = im_line_va_2; 00223 00224 //init serial 00225 pc.baud(115200); 00226 pc.printf("derp!\r\n"); 00227 00228 //init buffers 00229 init_buffers(); 00230 //init timer 00231 t.attach_us(&isr,64); 00232 00233 /*init SD card, wait for card insertion*/ 00234 SDFileSystem sd(DI, DO, SCK, CS, "sd"); 00235 while (sd.disk_status()) { 00236 sd.disk_initialize(); 00237 wait(0.5); 00238 } 00239 00240 FILE *fp; 00241 00242 fp = fopen("/sd/man.worm.starwars", "rb"); 00243 00244 uint32_t old_tics = tics; 00245 for(;;) 00246 { 00247 if (feof(fp)) rewind(fp); 00248 fread(current_frame, 1, VIDEO_FRAME_SIZE, fp); 00249 for(int x = 0; x < XL; x += 2) 00250 { 00251 for(int y = 0; y < YL; y++) 00252 { 00253 im_back[H_RES*(y+Y0) + x + X0] = current_frame[YL - y][x >> 1] >> 4; 00254 im_back[H_RES*(y+Y0) + x + 1 + X0] = current_frame[YL - y][x >> 1]; 00255 } 00256 } 00257 00258 buf_swap_tmp = im_line_va; 00259 im_line_va = im_back; 00260 im_back = buf_swap_tmp; 00261 00262 led_state = !led_state; 00263 user_led = led_state; 00264 00265 char status_string[20]; 00266 sprintf(status_string, "%.1f", 15625.f / (float)(tics - old_tics)); 00267 old_tics = tics; 00268 set_status_string(status_string); 00269 } 00270 } 00271 00272
Generated on Sat Jul 16 2022 04:21:08 by
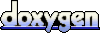