
robot
Embed:
(wiki syntax)
Show/hide line numbers
DQMapper.h
00001 #ifndef __DQ_MAPPER_H 00002 #define __DQ_MAPPER_H 00003 00004 #include "tables.h" 00005 00006 class DQMapper { 00007 public: 00008 virtual void map(float torque_percent, float w, float *d, float *q) = 0; 00009 }; 00010 00011 class QOnlyMapper : public DQMapper { 00012 public: 00013 QOnlyMapper(float kt, float tmax) {_kt = kt; _tmax = tmax;} 00014 virtual void map(float torque_percent, float w, float *d, float *q) {*d = 0; *q = torque_percent * _tmax / _kt;} 00015 private: 00016 float _kt; 00017 float _tmax; 00018 }; 00019 00020 class LinearNoFWMapper : public DQMapper { 00021 public: 00022 LinearNoFWMapper(float kt, float tmax, float lambda) {_kt = kt; _tmax = tmax; _lambda = lambda;} 00023 virtual void map(float torque_percent, float w, float *d, float *q); 00024 private: 00025 float _kt; 00026 float _tmax; 00027 float _lambda; 00028 }; 00029 00030 class LutMapper : public DQMapper { 00031 public: 00032 virtual void map(float torque_percent, float w, float *d, float *q); 00033 }; 00034 00035 class InterpolatingLutMapper : public DQMapper { 00036 public: 00037 virtual void map(float torque_percent, float w, float *d, float *q); 00038 private: 00039 static float lookup(short table[][COLUMNS], int row, int col); 00040 static float lookup(short *table, int index); 00041 static float interp(float a, float b, float eps); 00042 static float interp(float a, float b, float c, float eps_row, float eps_col); 00043 }; 00044 00045 class AngleMapper : public DQMapper { 00046 public: 00047 AngleMapper(float theta, float is) {_theta = theta; _is = is;} 00048 virtual void map(float torque_percent, float w, float *d, float *q); 00049 private: 00050 float _is; 00051 float _theta; 00052 }; 00053 00054 class DirectMapper : public DQMapper { 00055 public: 00056 DirectMapper(float id, float iq) {_id = id; _iq = iq;} 00057 virtual void map(float torque_percent, float w, float *d, float *q); 00058 private: 00059 float _id, _iq; 00060 }; 00061 00062 class SwapMapper : public DQMapper { 00063 public: 00064 SwapMapper(float id, float iq) {_id = id; _iq = iq;} 00065 virtual void map(float torque_percent, float w, float *d, float *q); 00066 private: 00067 float _id, _iq; 00068 }; 00069 00070 class AutoMapper : public DQMapper { 00071 public: 00072 AutoMapper(float phase_low, float phase_high, float steps, float is) 00073 {_phase_low = phase_low; _phase_high = phase_high; _steps = steps; _is = is; _theta = _phase_low;} 00074 virtual void map(float torque_percent, float w, float *d, float *q); 00075 private: 00076 float _phase_low, _phase_high, _steps, _is; 00077 float _theta; 00078 }; 00079 00080 #endif
Generated on Tue Jul 12 2022 17:58:39 by
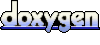