
robot
Embed:
(wiki syntax)
Show/hide line numbers
CommandProcessor.h
00001 #ifndef __COMMAND_PROCESSOR_H 00002 #define __COMMAND_PROCESSOR_H 00003 00004 #include "mbed.h" 00005 #include "PreferenceWriter.h" 00006 00007 void processCmd(Serial *pc, PreferenceWriter *pref, char *buf); 00008 void processCmdFast(Serial *pc, PreferenceWriter *pref, char *buf); 00009 00010 /*---variable loading, setting, and flashing---*/ 00011 void cmd_ls(Serial *pc); 00012 void cmd_ls2(Serial *pc, char *buf); 00013 void cmd_set(Serial *pc, char *buf, char *val); 00014 void cmd_defaults(Serial *pc); 00015 void cmd_reload(Serial *pc, PreferenceWriter *pref); 00016 void cmd_flush(Serial *pc, PreferenceWriter *pref); 00017 void cmd_query(Serial *pc, char *buf); 00018 00019 /*---mode switching---*/ 00020 void cmd_setp(Serial *pc, char *buf); 00021 void cmd_mode(Serial *pc, char *buf); 00022 void cmd_src(Serial *pc, char *buf); 00023 void cmd_op(Serial *pc, char *buf); 00024 00025 /*---variable commands---*/ 00026 void cmd_exit(Serial *pc); 00027 00028 /*---system commands---*/ 00029 void cmd_clear(Serial *pc); 00030 00031 /*---fast path commands---*/ 00032 void cmdf_w(Serial *pc); 00033 void cmdf_data(Serial *pc); 00034 void cmdf_setp(Serial *pc, char c); 00035 00036 /*---internal functions---*/ 00037 int tokenize(char *buf, char **out, int max); 00038 float *checkf(char *s); 00039 int *checkn(char *s); 00040 char *mode_to_str(int n); 00041 int str_to_mode(char *buf); 00042 char *src_to_str(int n); 00043 int str_to_src(char *buf); 00044 char *op_to_str(int n); 00045 int str_to_op(char *buf); 00046 00047 #endif
Generated on Tue Jul 12 2022 17:58:39 by
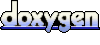