
Slow SD Logger
Dependencies: Adafruit_RTCLib BNO055 SDFileSystem_SlowLogger analoghalls mbed
Fork of analoghalls by
main.cpp
00001 #include "mbed.h" 00002 #include "SDFileSystem.h" 00003 #include "DS1307.h" 00004 #include "BNO055.h" 00005 #include "string.h" 00006 00007 #define M_PI 3.1415927f 00008 00009 #define DI D11 00010 #define DO D12 00011 #define SCK D13 00012 #define CS D10 00013 00014 #define I2C1_SDA PB_9 00015 #define I2C1_SCL PB_8 00016 00017 #define I2C2_SDA D5 00018 #define I2C2_SCL D7 00019 00020 #define PAGE_SIZE 4096 00021 #define clip(a) if ((a > 254)) a = 254 00022 00023 PwmOut pwm_r(D4); 00024 PwmOut pwm_g(D6); 00025 PwmOut pwm_b(D3); 00026 00027 char fname[255]; 00028 char current_time[64]; 00029 00030 unsigned char buf[PAGE_SIZE]; 00031 int buf_idx = 0; 00032 00033 void printDT(char *pre, DateTime &dt) 00034 { 00035 printf("%s %u/%u/%02u %2u:%02u:%02u\r\n" 00036 ,pre 00037 ,dt.month(),dt.day(),dt.year() 00038 ,dt.hour(),dt.minute(),dt.second() 00039 ); 00040 } 00041 00042 bool rtcUpdate(RtcDs1307 &rtc, int32_t bias) // this must be signed 00043 { bool bUpdated = false; 00044 00045 // Use the compiled date/time as a basis for setting the clock. 00046 // We assign it to a signed integer so that negative biases work correctly 00047 int64_t compiledTime = DateTime(__DATE__,__TIME__).unixtime(); 00048 00049 // This assumes that the program is run VERY soon after the initial compile. 00050 time_t localt = DateTime(compiledTime + bias).unixtime(); // offset by bias 00051 00052 // If the stored static time stamp does not equal the compiled time stamp, 00053 // then we need to update the RTC clock and the stored time stamp 00054 if(*((time_t *)&rtc[0]) != localt) 00055 { 00056 // Update the RTC time as local time, not GMT/UTC 00057 rtc.adjust(localt); 00058 // Store the new compiled time statically in the object ram image 00059 *((time_t *)&rtc[0]) = localt; 00060 // Push the object ram image to the RTC ram image 00061 bUpdated = rtc.commit(); 00062 } 00063 00064 return bUpdated; 00065 } 00066 00067 int main() { 00068 printf("%s\n", "Manworm's High-Speed Logger"); 00069 00070 /*init RGB lights*/ 00071 pwm_r.period(0.01); 00072 pwm_g.period(0.01); 00073 pwm_b.period(0.01); 00074 00075 pwm_r = 0.0f; 00076 pwm_g = 0.0f; 00077 pwm_b = 0.0f; 00078 00079 /*wait for SD card to be inserted*/ 00080 SDFileSystem sd(DI, DO, SCK, CS, "sd"); 00081 while (sd.disk_status()) { 00082 sd.disk_initialize(); 00083 wait(0.5); 00084 } 00085 00086 /*init RTC, get time and date for filename*/ 00087 I2C i2c1(I2C1_SDA, I2C1_SCL); 00088 i2c1.frequency(100000); 00089 RtcDs1307 rtc(i2c1); 00090 00091 rtcUpdate(rtc, -(5*60*60)); 00092 DateTime dt = rtc.now(); 00093 printDT("It is now", dt); 00094 00095 strcpy(fname, "/sd/log_"); 00096 sprintf(current_time, "%u.%u.%02u_%02u.%02u.%02u" 00097 ,dt.month(),dt.day(),dt.year() 00098 ,dt.hour(),dt.minute(),dt.second() 00099 ); 00100 strcat(fname, current_time); 00101 strcat(fname, ".csv"); 00102 printf("Logging to %s\n", fname); 00103 00104 FILE *fp = fopen(fname, "w"); 00105 00106 /*init IMU*/ 00107 BNO055 imu(I2C2_SDA, I2C2_SCL); 00108 imu.reset(); 00109 if (!imu.check()) { 00110 printf("%s\n", "IMU not ready"); 00111 } else { 00112 printf("BNO055 found\r\n\r\n"); 00113 printf("Chip ID: %03d\r\n",imu.ID.id); 00114 printf("Accelerometer ID: %03d\r\n",imu.ID.accel); 00115 printf("Gyroscope ID: %03d\r\n",imu.ID.gyro); 00116 printf("Magnetometer ID: %03d\r\n\r\n",imu.ID.mag); 00117 printf("Firmware version v%d.%0d\r\n",imu.ID.sw[0],imu.ID.sw[1]); 00118 printf("Bootloader version v%d\r\n\r\n",imu.ID.bootload); 00119 } 00120 imu.setmode(OPERATION_MODE_NDOF); 00121 imu.set_accel_units(MPERSPERS); 00122 00123 float theta = 0.0f; 00124 int tics = 0; 00125 for (;;) { 00126 float r, g, b; 00127 r = sinf(theta); 00128 g = sinf(theta + 2 * M_PI / 3); 00129 b = sinf(theta - 2 * M_PI / 3); 00130 00131 pwm_r = 0.5f + 0.5f * r; 00132 pwm_g = 0.5f + 0.5f * g; 00133 pwm_b = 0.5f + 0.5f * b; 00134 00135 theta += 0.001f; 00136 if (theta > 2 * M_PI) theta -= 2 * M_PI; 00137 00138 tics++; 00139 if (tics % 100 == 0) { 00140 tics = 0; 00141 00142 imu.get_accel(); 00143 fprintf(fp, "%d,%d,%d\n",(int) (100*imu.accel.x),(int)(100*imu.accel.y),(int)(100*imu.accel.z)); 00144 fflush(fp); 00145 } 00146 wait(0.001); 00147 } 00148 }
Generated on Thu Jul 14 2022 00:29:05 by
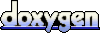