
Streams USB audio with sound effects applied. Sound effect selected by joystick and intensity altered by tilting the mbed. Output to the mbed-application-board phono jack.
Dependencies: C12832_lcd MMA7660 USBDevice mbed
user_interface.cpp
00001 /******************************************************************************* 00002 * Mangages LCD display, joystick and accelerometer for user interface. 00003 * Bryan Wade 00004 * 27 MAR 2014 00005 ******************************************************************************/ 00006 #include "mbed.h" 00007 #include "C12832_lcd.h" 00008 #include "MMA7660.h" 00009 #include "user_interface.h" 00010 #include "effects.h" 00011 00012 C12832_LCD lcd; 00013 MMA7660 accel(p28, p27, true); 00014 BusIn joystick(p15, p12, p13, p16); 00015 AnalogIn pot(p19); 00016 00017 static const int STICK_IDLE = 0; 00018 static const int STICK_UP = 1; 00019 static const int STICK_DOWN = 2; 00020 static const int STICK_LEFT = 4; 00021 static const int STICK_RIGHT = 8; 00022 00023 static uint8_t stickPrevious; 00024 static effect_mode_t selectedMode; 00025 static char *selectedModeName; 00026 static uint16_t effectGain; 00027 00028 /* 00029 * Update the display with mode, gain and buffer level. 00030 */ 00031 void updateDisplay(int32_t level) 00032 { 00033 lcd.locate(0,0); 00034 lcd.printf("Effect: %s ", selectedModeName); 00035 00036 lcd.locate(0,10); 00037 lcd.printf("Intensity: %d ", effectGain / (MAX_EFFECT_GAIN / 100)); 00038 00039 lcd.locate(0,20); 00040 lcd.printf("Buffer: %d ", level); 00041 } 00042 00043 /* 00044 * Get effects mode selected by joystick. 00045 */ 00046 void serviceJoystick(void) 00047 { 00048 uint8_t stick = joystick; 00049 00050 if (stick != stickPrevious) { 00051 stickPrevious = stick; 00052 00053 switch (stick) 00054 { 00055 case STICK_UP: 00056 selectedMode = EFFECT_ECHO; 00057 selectedModeName = "Echo"; 00058 break; 00059 00060 case STICK_DOWN: 00061 selectedMode = EFFECT_REVERB; 00062 selectedModeName = "Reverb"; 00063 break; 00064 00065 case STICK_LEFT: 00066 case STICK_RIGHT: 00067 selectedMode = EFFECT_STRAIGHT; 00068 selectedModeName = "Straight"; 00069 break; 00070 } 00071 } 00072 } 00073 00074 /* 00075 * Get effects gain from accelerometer tilt. 00076 */ 00077 void serviceAccel(void) 00078 { 00079 // Tilt sensitivity selected to produce max effect gain 00080 // at about 80 deg tilt. 00081 static const int32_t TILT_SENSITIVITY = 2750; 00082 int a[3]; 00083 accel.readData(a); 00084 //Convert x-axis raw accelerometer data to the effect gain. 00085 int32_t x = a[0] * TILT_SENSITIVITY; 00086 if (x < 0) x = -x; 00087 if (x > MAX_EFFECT_GAIN) x = MAX_EFFECT_GAIN; 00088 effectGain = (uint16_t)x; 00089 } 00090 00091 /* 00092 * Initialize the joystick and display. 00093 */ 00094 void UI_Initialize(void) 00095 { 00096 lcd.cls(); 00097 stickPrevious = STICK_IDLE; 00098 selectedMode = EFFECT_STRAIGHT; 00099 selectedModeName = "Straight"; 00100 effectGain = 0; 00101 // Set the accelerometer to sample slightly 00102 // faster than we poll the user interface. 00103 accel.setSampleRate(32); 00104 } 00105 00106 /* 00107 * Updates display, joystick and accelerometer 00108 */ 00109 void UI_Update(int32_t bufferLevel) 00110 { 00111 serviceJoystick(); 00112 serviceAccel(); 00113 updateDisplay(bufferLevel); 00114 } 00115 00116 00117 effect_mode_t UI_GetEffectMode(void) { return selectedMode; } 00118 00119 uint16_t UI_GetEffectGain(void) { return effectGain; } 00120 00121 00122
Generated on Thu Jul 14 2022 01:44:22 by
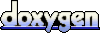