
Streams USB audio with sound effects applied. Sound effect selected by joystick and intensity altered by tilting the mbed. Output to the mbed-application-board phono jack.
Dependencies: C12832_lcd MMA7660 USBDevice mbed
main.cpp
00001 /******************************************************************************* 00002 * Project: USB Sound Effects. 00003 * 00004 * Uses the mbed LPC1768 and mbed-application-board to create a USB audio device 00005 * that streams audio from a host computer to headphones or powered speakers. 00006 * A couple different sound effects can be applied to the stream in real-time, 00007 * tilting the mbed alters the effects. 00008 * 00009 * ECHO 00010 * The joystick selects ) | 00011 * one of three effect ) STRAIGHT - o - STRAIGHT 00012 * modes. ) | 00013 * REVERB 00014 * 00015 * 00016 * 00017 * \\ || 00018 * Tilting the mbed ) ====== \\ || 00019 * determines intensity ) \\ || 00020 * of the effect. ) 00021 * 0% 50% 100% 00022 * 00023 * The LCD display shows the current effect mode, intesity and buffer level. 00024 * 00025 * For better audio quality, packets are buffered before playbeck. Playback is 00026 * halted when the buffer drops below two packets and resumes when it is greater 00027 * than four packets. This creates a lag of a few milliseconds, which is not 00028 * detectable by a listener using straight playback mode. 00029 * 00030 * The echo effect produces echos with a delay of about 250 milliseconds. Tilting 00031 * the mbed alters the persistence of echo. 00032 * 00033 * The reverb effect utilizes multiple delay lines of length varying from 00034 * about 25 to 200 milliseconds. Again, tilting the mbed alters the 00035 * persistence. 00036 * 00037 * Implemenation Notes: 00038 * Audio playback is implement with an mbed ticker, and it is critical that this interrupt 00039 * is not delayed to acheive decent audio quality. USB audio packet handling also uses 00040 * interrupts, but the priority is set lower to ensure proper playback. The USBAudio class 00041 * was modified to use a callback to transfer the received packet from the packet buffer to 00042 * the larger playback buffer. The user interface is handled entirely in the user-context 00043 * since it has only soft timing requirements. 00044 * 00045 * Future work: 00046 * 1. Clocks within the host computer and mbed will differ slightly, so the 00047 * average sample rate from the computer will not exactly match the average mbed 00048 * playback rate. Over time the playback buffer level with creep until it becomes 00049 * totaly empty or full. The current implementation addresses this by simply 00050 * increasing the buffer size and selecting a sample rate that the mbed 00051 * can replicate most accurately (mulitple of 1 us to use mbed Ticker). A better 00052 * solution may be to slightly alter the playback rate in response to the buffer level. 00053 * For example use 20us rate when the buffer level is high and 19us when the buffer 00054 * level is low. Of course this would distort the playback frequecy by 5%, so it would 00055 * be even better to make a custom timer interrupt with better resolution than the 00056 * mbed ticker. 00057 * 00058 * 2. It would be interesting to add stereo audio, but the mbed-application-board 00059 * audio jack is mono so this would require hardware customization. 00060 * 00061 * Bryan Wade 00062 * 27 MAR 2014 00063 ******************************************************************************/ 00064 00065 #include "mbed.h" 00066 #include "audio.h" 00067 #include "effects.h" 00068 #include "user_interface.h" 00069 00070 int main() 00071 { 00072 UI_Initialize(); // Init the user interface. 00073 Audio_Initialize(); // Starts audio playback. 00074 00075 while (true) 00076 { 00077 // Check the playback buffer level. 00078 int32_t level = Audio_CheckPlaybackBufferLevel(); 00079 00080 // Update the user interface, passing in the current buffer level 00081 // for display 00082 UI_Update(level); 00083 00084 // Set the effect mode and gain according to the user input. 00085 Effects_SetMode(UI_GetEffectMode()); 00086 Effects_SetGain(UI_GetEffectGain()); 00087 00088 // 50 milliseconds provides good UI responsivness. 00089 wait(.05); 00090 } 00091 } 00092
Generated on Thu Jul 14 2022 01:44:22 by
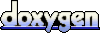