
Streams USB audio with sound effects applied. Sound effect selected by joystick and intensity altered by tilting the mbed. Output to the mbed-application-board phono jack.
Dependencies: C12832_lcd MMA7660 USBDevice mbed
delay.cpp
00001 /******************************************************************************* 00002 * Implements delay lines for audio samples. 00003 * Bryan Wade 00004 * 27 MAR 2014 00005 ******************************************************************************/ 00006 #include "delay.h" 00007 #include "string.h" 00008 00009 struct delay_t { // Implementation of opaque type. 00010 int16_t *base; 00011 int16_t *head; 00012 size_t len; 00013 }; 00014 00015 /* 00016 * Create a delay object given a ptr and size of available RAM. 00017 * This is only allocating memory for the metadata. The location of 00018 * the buffer memory is passed in. 00019 * @return Ptr to the new delay. 00020 */ 00021 delay_t *Delay_Create(void) 00022 { 00023 return (delay_t *)malloc(sizeof(delay_t)); 00024 } 00025 00026 /* 00027 * Configure delay buffer location and size. 00028 * @return True if successful. 00029 */ 00030 bool Delay_Configure(delay_t *delay, void *ram, size_t size) 00031 { 00032 if (delay) { 00033 delay->base = delay->head = (int16_t *)ram; 00034 delay->len = size / sizeof(int16_t); 00035 memset(ram, 0, size); 00036 return true; 00037 } 00038 00039 return false; 00040 } 00041 00042 /* 00043 * Push a new sample with feedback into the delay while simulateously retieving the output sample. 00044 * @return Output data. 00045 */ 00046 int16_t Delay_WriteWithFeedback(delay_t *delay, int16_t dataIn, uint16_t gain) 00047 { 00048 int16_t dataOut = *delay->head; 00049 00050 // Feedback gain is fixed-point Q16 format. 00051 *delay->head = dataIn + ((gain * dataOut ) >> 16); 00052 00053 if (++delay->head >= delay->base + delay->len) 00054 delay->head = delay->base; 00055 00056 return dataOut; 00057 } 00058
Generated on Thu Jul 14 2022 01:44:22 by
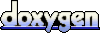