microbit sender
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 //including library 00002 #include "MicroBit.h" 00003 00004 00005 MicroBit uBit; 00006 00007 bool BP = false; 00008 00009 //defining times for button pressing 00010 uint64_t readtime, timepressed; 00011 00012 00013 //state of when code is running and not 00014 bool mcode = true; 00015 00016 //button function for seeing whether dot or dash 00017 void button() 00018 { 00019 while(BP == true) 00020 { 00021 P0.setDigitalValue(1); 00022 }P0.setDigtalValue(0); 00023 00024 00025 //if button was pressed once 00026 if (timepressed > 10 && timepressed < 500) 00027 { 00028 //shows a dot on the screen 00029 uBit.display.scroll("."); 00030 //sets digital value as 0 00031 uBit.io.P0.setDigitalValue(1); 00032 00033 } 00034 //if button is held down for a brief amount of time 00035 else if (timepressed > 500 && timepressed < 1000) 00036 { 00037 //shows a dash on screen 00038 uBit.display.scroll("-"); 00039 //sets digital value at 1 00040 uBit.io.P0.setDigitalValue(1); 00041 00042 } 00043 //if button held down for a longer time 00044 else if (timepressed > 1500) 00045 { 00046 //displays stop along the screen 00047 uBit.display.scroll("STOP"); 00048 //sets mcode as false so buttons stop running 00049 mcode = false; 00050 00051 } 00052 00053 } 00054 00055 00056 //button event from message bus for as button is being pressed 00057 void onButton(MicroBitEvent e) 00058 { 00059 //if mcode is true signals program needs to run 00060 if (mcode == true) 00061 { 00062 //if button A is pressed 00063 if (e.source == MICROBIT_ID_BUTTON_A) 00064 { 00065 //calculating reading on button being pressed 00066 BP = true; 00067 readtime = system_timer_current_time(); 00068 } 00069 00070 } 00071 } 00072 00073 //button event from message bus for when button being let go of 00074 void offButton(MicroBitEvent e) 00075 { 00076 //if mcode is true signals program needs to run 00077 if (mcode == true) 00078 { 00079 //if button A is pressed 00080 if (e.source == MICROBIT_ID_BUTTON_A) 00081 { 00082 //sees how long button pressed for 00083 timepressed = system_timer_current_time() - readtime; 00084 //calls button function 00085 button(); 00086 00087 00088 } 00089 00090 } 00091 } 00092 00093 //main function 00094 int main() 00095 { 00096 // Initialise the micro:bit runtime. 00097 uBit.init(); 00098 00099 //if mcode is true signals program needs to run 00100 if (mcode == true) 00101 { 00102 00103 //message buses for function onButton and offButton for when button clicked 00104 uBit.messageBus.listen(MICROBIT_ID_BUTTON_A, MICROBIT_BUTTON_EVT_DOWN, onButton); 00105 00106 uBit.messageBus.listen(MICROBIT_ID_BUTTON_A, MICROBIT_BUTTON_EVT_UP, offButton); 00107 00108 00109 00110 00111 00112 00113 00114 } 00115 00116 // If main exits, there may still be other fibers running or 00117 // registered event handlers etc. 00118 // Simply release this fiber, which will mean we enter the 00119 // scheduler. Worse case, we then 00120 // sit in the idle task forever, in a power efficient sleep. 00121 release_fiber(); 00122 }
Generated on Wed Aug 24 2022 19:52:39 by
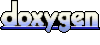