
Thread Sync Example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // see: [[RTOS: Demonstration Setup]] 00002 // Trace ab 15:00 00003 #include "mbed.h" 00004 #include "C12832.h" 00005 00006 C12832 lcd(p5, p7, p6, p8, p11); 00007 00008 class Rgb 00009 { 00010 private: 00011 DigitalOut _led; 00012 00013 public: 00014 Rgb(PinName ld) : _led(ld) { _led = 1;}; // Constructor 00015 00016 void LedOn() { 00017 _led = 0; 00018 } 00019 void LedOff(){ 00020 _led.write(1); 00021 } 00022 }; 00023 00024 class HasA 00025 { 00026 private: 00027 DigitalOut _led; 00028 00029 public: 00030 HasA(PinName ld) : _led(ld) {}; // Constructor 00031 00032 void LedOn() { 00033 _led = 1; 00034 } 00035 void LedOff(){ 00036 _led.write(0); 00037 } 00038 }; 00039 00040 void Delay_Nonsense(uint32_t * DelayCounter, uint32_t const * TargetCount) 00041 { 00042 while(*DelayCounter <= *TargetCount) 00043 { 00044 *DelayCounter = *DelayCounter + 1; 00045 } 00046 00047 *DelayCounter = 0; 00048 } 00049 00050 //M3 r (p23); g (p24); b (p25); 00051 /* 00052 HasA Led1(LED1); 00053 HasA Led2(LED2); 00054 HasA Led3(LED3); 00055 */ 00056 Rgb Led1(p23); 00057 Rgb Led2(p24); 00058 Rgb Led3(p25); 00059 00060 //void Led1_Blink(void *pvParameters) 00061 void Led1_Blink() // red long 00062 { 00063 const int xDelay = 500; 00064 uint32_t BlueDelay = 0; 00065 const uint32_t TargetCount = 16000; 00066 00067 for(;;) { 00068 for(int i = 0; i < 10; i++) { // randomnes 00069 wait_ms(xDelay); 00070 } 00071 00072 { 00073 lcd.cls(); 00074 lcd.locate(0,3); 00075 lcd.printf("Thread 1 RED LED blinks \r\nRRRRRRRRRR!"); 00076 wait(1); 00077 Led1.LedOn(); 00078 Delay_Nonsense(&BlueDelay, &TargetCount); 00079 wait_ms(xDelay); 00080 Led1.LedOff(); 00081 Delay_Nonsense(&BlueDelay, &TargetCount); 00082 wait_ms(xDelay); 00083 } 00084 } 00085 } 00086 00087 void Led2_Blink() // green 00088 { 00089 const int xDelay = 250; 00090 uint32_t BlueDelay = 0; 00091 const uint32_t TargetCount = 16000; 00092 00093 for(;;) { 00094 for(int i = 0; i < 10; i++) { // randomnes 00095 wait_ms(xDelay); 00096 } 00097 { 00098 lcd.cls(); 00099 lcd.locate(0,3); 00100 lcd.printf("Thread 2 GREEN LED blinks \r\nGGGGGGGGGGG!"); 00101 wait(1); 00102 00103 Led2.LedOn(); 00104 Delay_Nonsense(&BlueDelay, &TargetCount); 00105 wait_ms(xDelay); 00106 Led2.LedOff(); 00107 Delay_Nonsense(&BlueDelay, &TargetCount); 00108 wait_ms(xDelay); 00109 } 00110 } 00111 } 00112 00113 void Led3_Blink() // blue very short 00114 { 00115 const int xDelay = 100; 00116 uint32_t BlueDelay = 0; 00117 const uint32_t TargetCount = 16000; 00118 00119 for(;;) { 00120 for(int i = 0; i < 10; i++) { // randomnes 00121 wait_ms(xDelay); 00122 } 00123 { 00124 lcd.cls(); 00125 lcd.locate(0,3); 00126 lcd.printf("Thread 3 BLUE LED blinks \r\nxxxxxxxxxx!"); 00127 wait(1); 00128 00129 Led3.LedOn(); 00130 Delay_Nonsense(&BlueDelay, &TargetCount); 00131 wait_ms(xDelay); 00132 Led3.LedOff(); 00133 Delay_Nonsense(&BlueDelay, &TargetCount); 00134 wait_ms(xDelay); 00135 } 00136 } 00137 } 00138 00139 DigitalOut Led4(LED4); 00140 volatile bool running = true; 00141 00142 // Callback function to pass arguments to params 00143 void blink(DigitalOut *led) { 00144 osThreadSetPriority(osThreadGetId(), osPriorityIdle); 00145 while (running) { 00146 *led = !*led; 00147 wait(1); 00148 } 00149 } 00150 00151 // https://docs.mbed.com/docs/mbed-os-api-reference/en/latest/APIs/tasks/rtos/#thread 00152 00153 //Thread thread1(osPriorityRealtime, DEFAULT_STACK_SIZE, NULL); 00154 Thread thread1; 00155 Thread thread2; 00156 Thread thread3; 00157 // parametrisierter Thread 00158 Thread pthread (osPriorityNormal, DEFAULT_STACK_SIZE, NULL); 00159 00160 int main() { 00161 thread1.start(Led1_Blink); 00162 thread2.start(Led2_Blink); 00163 thread3.start(Led3_Blink); 00164 // Start parametrisierter Thread mit Callback Funktion 00165 pthread.start(callback(blink, &Led4)); 00166 00167 osThreadSetPriority(osThreadGetId(), osPriorityIdle); // osPriorityHigh, 00168 // osPriorityIdle 00169 Thread::yield(); 00170 printf("Priority is %i\r\n", thread1.get_priority()); 00171 Thread::wait(1); 00172 00173 thread1.join(); 00174 thread2.join(); 00175 thread3.join(); 00176 00177 while(1) { 00178 } 00179 thread1.terminate(); 00180 }
Generated on Sun Jul 31 2022 15:35:24 by
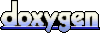