This library is an attempt to encapsulate the Pololu motor board MC33926 Motor Driver Carrier.
Embed:
(wiki syntax)
Show/hide line numbers
Motor.cpp
00001 #include "Motor.h" 00002 #include "mbed.h" 00003 00004 00005 Motor::Motor(PinName pwm, PinName dir1, PinName dir2): _pwm(pwm), _dir1(dir1), _dir2(dir2) 00006 { 00007 _direction=1; //default fwd 00008 _pwm.period(0.00005); //20Khz 00009 _pwm = 0; //default pwm off 00010 _dir1 = 0; 00011 _dir2 = 0; 00012 00013 } 00014 00015 float Motor::speed(float speed, bool direction) 00016 { 00017 if(direction == true) { 00018 _dir1=1; 00019 _dir2=0; 00020 } else { 00021 _dir1=0; 00022 _dir2=1; 00023 } 00024 if(speed > 1) { 00025 speed = 1; 00026 } 00027 if(speed < -1) { 00028 speed = -1; 00029 } 00030 00031 _pwm = abs(speed); 00032 return speed; 00033 } 00034 00035 float Motor::speed(float speed) 00036 { 00037 if(speed > 1) { 00038 speed = 1; 00039 } 00040 if(speed < -1) { 00041 speed = -1; 00042 } 00043 if(speed < 0) { 00044 _dir1=1; 00045 _dir2=0; 00046 } else { 00047 _dir1=0; 00048 _dir2=1; 00049 } 00050 _pwm = abs(speed); 00051 return speed; 00052 } 00053 00054 Motor& Motor::operator= (float speed) 00055 { 00056 if(speed > 1) { 00057 speed = 1; 00058 } 00059 if(speed < -1) { 00060 speed = -1; 00061 } 00062 if(speed < 0) { 00063 _dir1=1; 00064 _dir2=0; 00065 } else { 00066 _dir1=0; 00067 _dir2=1; 00068 } 00069 _pwm = abs(speed); 00070 return *this; 00071 }
Generated on Fri Jul 15 2022 02:07:01 by
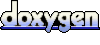