corrected error assignation of motors in methods right and left. now it work fun. Added function to read calibrated sensors data.
Fork of m3pi by
m3pi.h
00001 /* mbed m3pi Library 00002 * Copyright (c) 2007-2010 cstyles 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef M3PI_H 00024 #define M3PI_H 00025 00026 #include "mbed.h" 00027 #include "platform.h" 00028 00029 #ifdef MBED_RPC 00030 #include "rpc.h" 00031 #endif 00032 00033 #define SEND_SIGNATURE 0x81 00034 #define SEND_RAW_SENSOR_VALUES 0x86 00035 #define SEND_CALIB_SENSOR_VALUES 0x87 00036 #define SEND_TRIMPOT 0xB0 00037 #define SEND_BATTERY_MILLIVOLTS 0xB1 00038 #define DO_PLAY 0xB3 00039 #define PI_CALIBRATE 0xB4 00040 #define DO_CLEAR 0xB7 00041 #define DO_PRINT 0xB8 00042 #define DO_LCD_GOTO_XY 0xB9 00043 #define LINE_SENSORS_RESET_CALIBRATION 0xB5 00044 #define SEND_LINE_POSITION 0xB6 00045 #define AUTO_CALIBRATE 0xBA 00046 #define SET_PID 0xBB 00047 #define STOP_PID 0xBC 00048 #define M1_FORWARD 0xC1 00049 #define M1_BACKWARD 0xC2 00050 #define M2_FORWARD 0xC5 00051 #define M2_BACKWARD 0xC6 00052 00053 00054 00055 /** m3pi control class 00056 * 00057 * Example: 00058 * @code 00059 * // Drive the m3pi forward, turn left, back, turn right, at half speed for half a second 00060 00061 #include "mbed.h" 00062 #include "m3pi.h" 00063 00064 m3pi pi; 00065 00066 int main() { 00067 00068 wait(0.5); 00069 00070 pi.forward(0.5); 00071 wait (0.5); 00072 pi.left(0.5); 00073 wait (0.5); 00074 pi.backward(0.5); 00075 wait (0.5); 00076 pi.right(0.5); 00077 wait (0.5); 00078 00079 pi.stop(); 00080 00081 } 00082 * @endcode 00083 */ 00084 class m3pi : public Stream { 00085 00086 // Public functions 00087 public: 00088 00089 /** Create the m3pi object connected to the default pins 00090 * 00091 * @param nrst GPIO pin used for reset. Default is p23 00092 * @param tx Serial transmit pin. Default is p9 00093 * @param rx Serial receive pin. Default is p10 00094 */ 00095 m3pi(); 00096 00097 00098 /** Create the m3pi object connected to specific pins 00099 * 00100 */ 00101 m3pi(PinName nrst, PinName tx, PinName rx); 00102 00103 00104 00105 /** Force a hardware reset of the 3pi 00106 */ 00107 void reset (void); 00108 00109 /** Directly control the speed and direction of the left motor 00110 * 00111 * @param speed A normalised number -1.0 - 1.0 represents the full range. 00112 */ 00113 void left_motor (float speed); 00114 00115 /** Directly control the speed and direction of the right motor 00116 * 00117 * @param speed A normalised number -1.0 - 1.0 represents the full range. 00118 */ 00119 void right_motor (float speed); 00120 00121 /** Drive both motors forward as the same speed 00122 * 00123 * @param speed A normalised number 0 - 1.0 represents the full range. 00124 */ 00125 void forward (float speed); 00126 00127 /** Drive both motors backward as the same speed 00128 * 00129 * @param speed A normalised number 0 - 1.0 represents the full range. 00130 */ 00131 void backward (float speed); 00132 00133 /** Drive left motor backwards and right motor forwards at the same speed to turn on the spot 00134 * 00135 * @param speed A normalised number 0 - 1.0 represents the full range. 00136 */ 00137 void left (float speed); 00138 00139 /** Drive left motor forward and right motor backwards at the same speed to turn on the spot 00140 * @param speed A normalised number 0 - 1.0 represents the full range. 00141 */ 00142 void right (float speed); 00143 00144 /** Stop both motors 00145 * 00146 */ 00147 void stop (void); 00148 00149 /** Read the voltage of the potentiometer on the 3pi 00150 * @returns voltage as a float 00151 * 00152 */ 00153 float pot_voltage(void); 00154 00155 /** Read the battery voltage on the 3pi 00156 * @returns battery voltage as a float 00157 */ 00158 float battery(void); 00159 00160 /** Read the position of the detected line 00161 * @returns position as A normalised number -1.0 - 1.0 represents the full range. 00162 * -1.0 means line is on the left, or the line has been lost 00163 * 0.0 means the line is in the middle 00164 * 1.0 means the line is on the right 00165 */ 00166 float line_position (void); 00167 /** lecture capteurs calibres 00168 * @returns tableau val capteurs de gauche à droite 00169 * 0 blanc (reflexion max) 00170 * 1000 noir (pas de reflexion) 00171 */ 00172 void calibrated_sensors(unsigned short ltab[5]); 00173 00174 /** Calibrate the sensors. This turns the robot left then right, looking for a line 00175 * 00176 */ 00177 char sensor_auto_calibrate (void); 00178 00179 /** Set calibration manually to the current settings. 00180 * 00181 */ 00182 void calibrate(void); 00183 00184 /** Clear the current calibration settings 00185 * 00186 */ 00187 void reset_calibration (void); 00188 00189 void PID_start(int max_speed, int a, int b, int c, int d); 00190 00191 void PID_stop(); 00192 00193 /** Write to the 8 LEDs 00194 * 00195 * @param leds An 8 bit value to put on the LEDs 00196 */ 00197 void leds(int val); 00198 00199 /** Locate the cursor on the 8x2 LCD 00200 * 00201 * @param x The horizontal position, from 0 to 7 00202 * @param y The vertical position, from 0 to 1 00203 */ 00204 void locate(int x, int y); 00205 00206 /** Clear the LCD 00207 * 00208 */ 00209 void cls(void); 00210 00211 /** Send a character directly to the 3pi serial interface 00212 * @param c The character to send to the 3pi 00213 */ 00214 int putc(int c); 00215 00216 /** Receive a character directly to the 3pi serial interface 00217 * @returns c The character received from the 3pi 00218 */ 00219 int getc(); 00220 00221 /** Send a string buffer to the 3pi serial interface 00222 * @param text A pointer to a char array 00223 * @param int The character to send to the 3pi 00224 */ 00225 int print(char* text, int length); 00226 00227 #ifdef MBED_RPC 00228 virtual const struct rpc_method *get_rpc_methods(); 00229 #endif 00230 00231 private : 00232 00233 DigitalOut _nrst; 00234 Serial _ser; 00235 00236 void motor (int motor, float speed); 00237 virtual int _putc(int c); 00238 virtual int _getc(); 00239 00240 }; 00241 00242 #endif
Generated on Mon Jul 18 2022 21:55:03 by
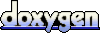