
HW 7.1 Attempt to use button timers to check for button status instead of interrupts
Fork of slider_diatonic_v1 by
slider_diatonic_v1.cpp
00001 #include "mbed.h" 00002 #include "SLCD.h" 00003 #include "TSISensor.h" 00004 00005 00006 #define CHANNELON 0 00007 #define CHANNELOFF 1 00008 #define LCDLEN 10 00009 #define DATATIME 100 //milli seccnds 00010 //LCD messages 00011 00012 00013 00014 // Operating parameters 00015 #define SIDETONE 700.0 00016 #define TONEMIN 200.0 00017 #define TONEINT 800.00 // So tone max is 1000 00018 #define TONEON 0.50 00019 #define TONEOFF 0.0 00020 #define SPEEDAST 0 00021 #define TONEAST 1 00022 #define NUMTONES 10 00023 #define LEDPERIOD 0.001 00024 //#define PRINTDEBUG 00025 00026 #define NUMOCTS 1 00027 //Button --> 00028 #define BUTOFF 1 00029 #define BUTON 0 00030 #define BUTTON PTC3 00031 #define PUSHTIME 1000 //wait to check for button press 00032 00033 Serial pc(USBTX, USBRX); 00034 00035 float diatonicScale[NUMTONES] = {246.94, 261.63,293.66,329.63,349.23,392.00,440.00,493.88,523.25,587.33}; 00036 SLCD slcd; //define LCD display 00037 00038 TSISensor tsiScaling; // Capacitive sensor/slider 00039 00040 00041 PwmOut led(LED_RED); 00042 DigitalOut outPin(PTC9); //J1-16 00043 PwmOut soundOut(PTA13); 00044 Timer dataTimer; 00045 // Global scalars 00046 char lcdData[LCDLEN]; 00047 00048 float tonePeriod; 00049 float toneFreq = SIDETONE; 00050 00051 //Timers--> 00052 Timer buttonTimer;//checks for button state change after set amout of time. 00053 00054 //buttons --> 00055 00056 int LButtonState = BUTOFF; //Set the button state to off 00057 DigitalIn LftButton(BUTTON); //PTC3 00058 00059 //Octave changer --> 00060 int octaveState = NUMOCTS; //Not sure what value this should be 00061 00062 void LCDMessNoDwell(char *lMess){ 00063 slcd.Home(); 00064 slcd.clear(); 00065 slcd.printf(lMess); 00066 } 00067 00068 void diatonicAdjust( float scaling) { 00069 int tempInt; 00070 int scaleIndex; 00071 static int oldScaleIndex = 0; 00072 /* There appears to be a set up time for setting the PWM time period 00073 only do a nes set up if the indext changes. 00074 */ 00075 scaleIndex = (int)(NUMTONES * scaling); 00076 if (scaleIndex != oldScaleIndex) { 00077 toneFreq = diatonicScale[scaleIndex]; 00078 tonePeriod = 1.0/toneFreq; 00079 soundOut.period(tonePeriod); // adjusting period 00080 soundOut.write(TONEON); // there is a setup time for both period and DF 00081 oldScaleIndex = scaleIndex; 00082 } else { 00083 return; 00084 } 00085 #ifdef PRINTDEBUG 00086 pc.printf(" %f,%d\n\r",scaling, scaleIndex ); 00087 #endif 00088 tempInt = (int)toneFreq; 00089 sprintf (lcdData,"%4d",tempInt); 00090 LCDMessNoDwell(lcdData); 00091 return; 00092 } 00093 void lightAdjust( float scaling) { // Control brightness of LED 00094 float tempDutyFactor; 00095 00096 tempDutyFactor = 1.0 - scaling; //LED is a sinking connection // anode is held at 5V 00097 led.write(tempDutyFactor); //adjusting duty factor 00098 return; 00099 } 00100 00101 int main(){ 00102 int tempInt; 00103 float tempValue; 00104 00105 tonePeriod = 1.0/toneFreq; 00106 soundOut.period(tonePeriod); 00107 led.period(tonePeriod); 00108 led.write(CHANNELOFF); 00109 outPin.write(CHANNELOFF); 00110 00111 tempInt = (int)toneFreq; 00112 sprintf (lcdData,"%4d",tempInt); 00113 LCDMessNoDwell(lcdData); 00114 // Start data timer 00115 dataTimer.start(); 00116 dataTimer.reset(); 00117 buttonTimer.start(); //checks for button state change after set amout of time. 00118 buttonTimer.reset(); 00119 00120 while (true) { 00121 00122 if(buttonTimer.read_ms() > PUSHTIME) { 00123 //check button first 00124 LButtonState = !LftButton; //button is off or on 00125 if(LButtonState) { 00126 octaveState++; 00127 octaveState = octaveState % NUMOCTS; 00128 } 00129 } 00130 00131 if (dataTimer.read_ms() > DATATIME){ // check to see if enough time has passed 00132 // to read the touch pad 00133 tempValue = tsiScaling.readPercentage(); 00134 if(tempValue > 0) { 00135 // soundOut.write(TONEON); // set duty factor to 50% 00136 diatonicAdjust(tempValue); 00137 lightAdjust(tempValue); 00138 } else { 00139 soundOut.write(TONEOFF); // set dutyfactor to 0% 00140 sprintf(lcdData, "%4d", octaveState + 1); 00141 LCDMessNoDwell("lcdData"); 00142 } 00143 dataTimer.reset(); 00144 } 00145 } // while forever 00146 }// end main
Generated on Thu Jul 21 2022 17:54:02 by
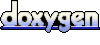