
Simple Menu Program for the Application Board LCD
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // A simple menu program, displaying on the mbed Application Board 00002 // Ideas on how to eliminate the 6 warnings would be appreciated! 00003 // Bo Barry 1/11/2014 (My first 'published' program) 00004 00005 00006 #include "mbed.h" 00007 DigitalOut myled(LED1); 00008 #include "C12832_lcd.h" 00009 C12832_LCD lcd; 00010 #include <stdio.h> 00011 00012 void load_menu(void); 00013 void sum(void); 00014 void rest(void); 00015 00016 int main(void) 00017 { 00018 00019 lcd.locate(0,2); 00020 lcd.cls(); 00021 lcd.printf("mbed Application Board!"); 00022 wait(2); 00023 load_menu(); 00024 return 0; 00025 } 00026 00027 void load_menu(void) 00028 { 00029 int choice; 00030 do 00031 { 00032 lcd.locate(0,2); 00033 lcd.cls(); 00034 lcd.printf("Menu\n"); 00035 lcd.printf("1. Sum 2. Rest 3. Exit\n"); 00036 scanf("%d",&choice); 00037 00038 switch(choice) 00039 { 00040 case 1: sum(); 00041 break; 00042 case 2: rest(); 00043 break; 00044 case 3: 00045 lcd.locate(0,2); 00046 lcd.cls(); 00047 lcd.printf("Quitting program!"); 00048 exit(0); 00049 break; 00050 default: lcd.printf("Invalid choice!"); 00051 break; 00052 } 00053 00054 } while (choice != 3); 00055 00056 } 00057 00058 void sum(void) 00059 { 00060 int num1, num2; 00061 // int ch; 00062 00063 printf("Enter number 1: "); 00064 scanf("%d",&num1); 00065 lcd.locate(0,2); 00066 lcd.cls(); 00067 printf("Enter number 2: "); 00068 scanf("%d",&num2); 00069 lcd.locate(0,2); 00070 lcd.cls(); 00071 printf("The sum of the numbers was: %d",num1+num2); 00072 wait(2); 00073 00074 // Flushes input buffer from the newline from scanf() 00075 // while ( (ch = getchar()) != '\n' && ch != EOF) ; 00076 lcd.locate(0,2); 00077 lcd.cls(); 00078 printf("Press ENTER to continue."); 00079 // while ( (ch = getchar()) != '\n' && ch != EOF); 00080 return; 00081 } 00082 00083 void rest(void) 00084 { 00085 lcd.locate(0,2); 00086 lcd.cls(); 00087 // int ch; 00088 printf("Sleepy sleepy... zZZzZzZz\n"); 00089 wait(2); 00090 printf("You now feel awake again!"); 00091 wait(2); 00092 // Flushes input buffer 00093 // while ((ch = getchar()) != '\n' && ch != EOF) ; 00094 00095 lcd.locate(0,2); 00096 lcd.cls(); 00097 printf("Press ENTER to continue."); 00098 // while ((ch = getchar()) != '\n' && ch != EOF); 00099 00100 return; 00101 }
Generated on Wed Jul 13 2022 01:41:11 by
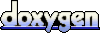