
VNG bot battle
Dependencies: BLE_API mbed nRF51822
MotorController.h
00001 /* 00002 * MotorController.h 00003 * 00004 * Created on: Aug 23, 2016 00005 * Author: tanpt 00006 */ 00007 00008 #ifndef MOTORCONTROLLER_H_ 00009 #define MOTORCONTROLLER_H_ 00010 00011 #include "common.h" 00012 00013 class MotorController { 00014 public: 00015 enum Direction { 00016 DIRECTION_FORWARD = 0, 00017 DIRECTION_BACKWARD = 1 00018 }; 00019 00020 enum BoostState { 00021 BOOST_DISABLE = 0, 00022 BOOST_ENBALE = 1 00023 }; 00024 00025 MotorController(); 00026 ~MotorController(); 00027 00028 int8_t setMotorRight(Direction direction, uint16_t speed); 00029 00030 int8_t setMotorLeft(Direction direction, uint16_t speed); 00031 00032 int8_t moveForward(uint16_t speed); 00033 00034 int8_t moveBackward(uint16_t speed); 00035 00036 int8_t turnRight(uint16_t speed); 00037 00038 int8_t turnLeft(uint16_t speed); 00039 00040 int8_t moveBackRight(uint16_t speed); 00041 00042 int8_t moveBackLeft(uint16_t speed); 00043 00044 int8_t setBoost(BoostState state); 00045 00046 private: 00047 static DigitalOut motorLeftDirection_; 00048 static PwmOut motorLeftSpeed_; 00049 static DigitalOut motorRightDirection_; 00050 static PwmOut motorRightSpeed_; 00051 00052 static DigitalOut motorBoostSpeed_; 00053 }; 00054 00055 #endif /* MOTORCONTROLLER_H_ */
Generated on Fri Aug 5 2022 18:35:57 by
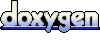