Big Mouth Billy Bass automation library
Embed:
(wiki syntax)
Show/hide line numbers
song.hpp
00001 #ifndef __included_song_hpp 00002 #define __included_song_hpp 00003 00004 #include <DirHandle.h> 00005 00006 #include "billybass.hpp" 00007 #include "config.hpp" 00008 #include "action.hpp" 00009 00010 class Song 00011 { 00012 public: 00013 typedef Action actions_t[ MAX_ACTIONS_PER_SONG ]; 00014 00015 // _name is relative to BASS_DIRECTORY 00016 // return a pointer to a fully read-in Song if valid 00017 static Song *newSong(char const *_name); 00018 00019 00020 void reset() { 00021 sequenceNumber = 0; 00022 whichFish = NO_FISH; 00023 basename = 0; 00024 extension = 0; 00025 numActions = 0; 00026 } 00027 00028 BillyBass * myFish() { 00029 return BillyBass::bassNumber(whichFish); 00030 } 00031 bool isValid() const { 00032 return basename != 0 && whichFish != NO_FISH; 00033 } 00034 bool parseFilename(char const *_name); 00035 bool readActions(); 00036 00037 unsigned getSequenceNumber() const { 00038 return sequenceNumber; 00039 } 00040 unsigned getWhichFish() const { 00041 return whichFish; 00042 } 00043 char const *getSampleFileName() { 00044 if (!extension) return 0; 00045 strcpy(extension, sampleExtension); 00046 return fullname; 00047 } 00048 char const *getTextFileName() { 00049 if (!extension) return 0; 00050 strcpy(extension, textExtension); 00051 return fullname; 00052 } 00053 Action *getActions() { 00054 return actions; 00055 } 00056 bool addAction(float _time, int _state, DigitalOut* _out, char _code); 00057 00058 unsigned getNumActions() const { 00059 return numActions; 00060 } 00061 00062 void print(FILE *f) { 00063 fprintf(f, "%s fish=%u seq=%u\r\n", getSampleFileName(), whichFish, sequenceNumber); 00064 Action *lastAction = actions + numActions; 00065 for (Action *action = actions; action < lastAction; action++) { 00066 fprintf(f, "%0.02f %s %d\r\n", action->actionTime, myFish()->outputName(action->output), action->desiredState); 00067 } 00068 } 00069 00070 protected: 00071 Song() { 00072 reset(); 00073 } 00074 ~Song() {} 00075 00076 static char const directoryName[ BASS_DIRECTORY_LENGTH + 1 ]; 00077 static unsigned const NO_FISH; 00078 static char const *textExtension; 00079 static char const *sampleExtension; 00080 static Song theSong; 00081 00082 unsigned sequenceNumber; // 0-relative 00083 unsigned whichFish; // 0-relative 00084 char fullname[ MAX_PATH_LEN ]; // with directory name 00085 char *basename; // points into fullname 00086 char *extension; // points into fullname 00087 actions_t actions; 00088 unsigned numActions; 00089 }; 00090 00091 #endif // __included_song_hpp
Generated on Sun Jul 17 2022 08:00:04 by
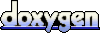