Big Mouth Billy Bass automation library
Embed:
(wiki syntax)
Show/hide line numbers
config_sample.hpp
00001 // copy this to config.hpp and edit as needed 00002 #ifndef __included_config_hpp 00003 #define __included_config_hpp 00004 00005 // configuration for Billy Bass 00006 #define MAX_FISH 2 00007 00008 // SD card layout: all files in BASS_DIRECTORY ("/sd/SD_Files/") 00009 // 00010 // Each pair of files in that directory are named like: 00011 // "<seqnum>_<fishnum>_filename.raw" (16-bit mono raw little-endian samples, sample rate=SAMPLE_RATE_HZ) 00012 // and 00013 // "<seqnum>_<fishnum>_filename.txt" (tab-separated text file with starttime,endtime,<mouth|head|body|tail> 00014 // text files are as exported from Audacity's label tracks 00015 // 00016 #define SD_NAME "sd" 00017 #define SD_ROOT "/" SD_NAME 00018 #define BASS_DIRECTORY SD_ROOT "/SD_Files" 00019 // length of BASS_DIRECTORY without NUL 00020 #define BASS_DIRECTORY_LENGTH 12 00021 #define MAX_BASENAME_LENGTH 30 00022 #define MAX_PATH_LEN \ 00023 BASS_DIRECTORY_LENGTH + 1 \ 00024 + MAX_BASENAME_LENGTH + 1 00025 00026 // limits of .txt files 00027 #define MAX_ACTIONS_LINES_PER_SONG 60 00028 #define MAX_ACTIONS_PER_SONG MAX_ACTIONS_LINES_PER_SONG*2 00029 #define MAX_TEXT_FILE_LENGTH 2048 00030 00031 // define this to 1 to make start times earlier and 00032 // enforce minimum on times 00033 #define FIX_TIMES 0 00034 00035 // Sample configuration 00036 typedef int16_t Sample_t; // 16-bit raw, LE samples 00037 const float SAMPLE_RATE_HZ = 8000.0; 00038 const unsigned SAMPLE_PERIOD_USEC = (unsigned)(1.0e6/SAMPLE_RATE_HZ); 00039 00040 // Player configuration 00041 const size_t BUFFER_SIZE = 512; 00042 const size_t SAMPLES_PER_BUFFER = BUFFER_SIZE / sizeof(Sample_t); 00043 const float SECONDS_PER_CHUNK = SAMPLES_PER_BUFFER / SAMPLE_RATE_HZ; 00044 00045 #define SERIAL_BAUD 115200 00046 #define ANALOG_OUTPUT_BIAS 0x8000 00047 00048 #define BODY_ON_DELAY 0.65 00049 #define BODY_OFF_DELAY 0.65 00050 #define TAIL_ON_DELAY 0.40 00051 #define TAIL_OFF_DELAY 0.40 00052 #define MOUTH_ON_DELAY 0.10 00053 #define MOUTH_OFF_DELAY 0.10 00054 #define MOUTH_MIN_ON_TIME 0.05 00055 00056 // Power: 00057 // Power GND J9/14 00058 // Vin (6V) J9/16 00059 00060 // I/O configuration 00061 #define BASS1_TAIL PTB0 /* J10/2 */ 00062 #define BASS1_MOUTH PTB1 /* J10/4 */ 00063 #define BASS1_BODY PTB2 /* J10/6 */ 00064 #define BASS1_BUTTON PTD5 /* J2/4 */ 00065 00066 #define BASS2_TAIL PTB3 /* J10/8 */ 00067 #define BASS2_MOUTH PTC2 /* J10/10 */ 00068 #define BASS2_BODY PTC1 /* J10/12 */ 00069 #define BASS2_BUTTON PTA13 /* J3/2 */ 00070 00071 // SD Card (3.3V) 00072 // PTD0 J2/6 D10 - Used for CS of SPI 00073 // PTD2 J2/8 D11 - Used for MOSI of SPI 00074 // PTD3 J2/10 D12 - Used for MISO of SPI 00075 // PTC5 J1/9 Used for SCLK of SPI (must solder if using shield) 00076 #define SD_MOSI PTD2 00077 #define SD_MISO PTD3 00078 #define SD_SCLK PTC5 00079 #define SD_CS PTD0 00080 00081 #endif
Generated on Sun Jul 17 2022 08:00:03 by
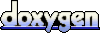