Big Mouth Billy Bass automation library
Embed:
(wiki syntax)
Show/hide line numbers
action.hpp
00001 #ifndef __included_action_hpp 00002 #define __included_action_hpp 00003 00004 #include "billybass.hpp" 00005 00006 // 0.000000\t5.646050\thead 00007 // 0.064498\t1.069177\ttail 00008 // 0.357219\t0.580481\tmouth 00009 00010 #define MAX_ACTION_LINE_LENGTH 30 00011 00012 struct Action { 00013 Action(float _time = -1.0, 00014 bool _state = false, 00015 DigitalOut *_out = 0, 00016 char const *_outName = 0) 00017 : actionTime(_time) 00018 , output(_out) 00019 , desiredState(_state) 00020 , code('.') { 00021 if (_outName) code = _outName[0]; 00022 } 00023 00024 bool operator < (Action const &other) const { 00025 if (actionTime < other.actionTime) return true; 00026 if (actionTime > other.actionTime) return false; 00027 return (code < other.code); 00028 } 00029 00030 // return <0 if *p1 is before *p2 00031 static int compare(const void* p1, const void* p2) { 00032 float tdiff = static_cast<Action const *>(p1)->actionTime - static_cast<Action const *>(p2)->actionTime; 00033 if (tdiff != 0.0) return tdiff; 00034 return static_cast<Action const *>(p1)->code - static_cast<Action const *>(p2)->code; 00035 } 00036 00037 bool isValid() const { 00038 return actionTime >= 0.0 && output != 0; 00039 } 00040 00041 void act() { 00042 output->write(desiredState ? 1 : 0); 00043 } 00044 00045 bool isPast(float _now) { 00046 return _now >= actionTime; 00047 } 00048 00049 bool actIfPast(float _now) { 00050 if (isPast(_now)) { 00051 act(); 00052 return true; 00053 } else return false; 00054 } 00055 00056 void set(float _time, int _state, DigitalOut* _out, char _code = '.') { 00057 actionTime = _time; 00058 desiredState = _state; 00059 output = _out; 00060 code = _code; 00061 } 00062 00063 float actionTime; 00064 DigitalOut *output; 00065 uint8_t desiredState; 00066 char code; 00067 }; 00068 00069 #endif
Generated on Sun Jul 17 2022 08:00:03 by
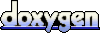