
API for driving Adafruit's NeoPixels using RedBear's BLE Nano or BLE nRF51822
Dependencies: BLE_API mbed nRF51822
Dependents: RedBearNano_NeoPixels_Example NeoTester
neopixel.cpp
00001 /* Lava 00002 * 00003 * WS2812B Tricolor LED (neopixel) controller 00004 * 00005 * 00006 * Example code: 00007 00008 neopixel_strip_t m_strip; 00009 uint8_t dig_pin_num = 6; 00010 uint8_t leds_per_strip = 24; 00011 uint8_t error; 00012 uint8_t led_to_enable = 10; 00013 uint8_t red = 255; 00014 uint8_t green = 0; 00015 uint8_t blue = 159; 00016 00017 neopixel_init(&m_strip, dig_pin_num, leds_per_strip); 00018 neopixel_clear(&m_strip); 00019 error = neopixel_set_color_and_show(&m_strip, led_to_enable, red, green, blue); 00020 if (error) { 00021 //led_to_enable was not within number leds_per_strip 00022 } 00023 //clear and remove strip 00024 neopixel_clear(&m_strip); 00025 neopixel_destroy(&m_strip); 00026 00027 00028 * For use with BLE stack, see information below: 00029 - Include in main.c 00030 #include "ble_radio_notification.h" 00031 - Call (see nrf_soc.h: NRF_RADIO_NOTIFICATION_DISTANCES and NRF_APP_PRIORITIES) 00032 ble_radio_notification_init(NRF_APP_PRIORITY_xxx, 00033 NRF_RADIO_NOTIFICATION_DISTANCE_xxx, 00034 your_radio_callback_handler); 00035 - Create 00036 void your_radio_callback_handler(bool radio_active) 00037 { 00038 if (radio_active == false) 00039 { 00040 neopixel_show(&strip1); 00041 neopixel_show(&strip2); 00042 //...etc 00043 } 00044 } 00045 - Do not use neopixel_set_color_and_show(...) with BLE, instead use uint8_t neopixel_set_color(...); 00046 */ 00047 00048 #include "mbed.h" // remove line if not using mbed 00049 00050 #include <stdbool.h> 00051 #include <stdint.h> 00052 #include <stdlib.h> 00053 #include "nrf_delay.h" 00054 #include "nrf_gpio.h" 00055 #include "neopixel.h" 00056 00057 00058 void neopixel_init(neopixel_strip_t *strip, uint8_t pin_num, uint16_t num_leds) 00059 { 00060 strip->leds = (color_t*) malloc(sizeof(color_t) * num_leds); 00061 strip->pin_num = pin_num; 00062 strip->num_leds = num_leds; 00063 nrf_gpio_cfg_output(pin_num); 00064 NRF_GPIO->OUTCLR = (1UL << pin_num); 00065 for (int i = 0; i < num_leds; i++) 00066 { 00067 strip->leds[i].simple.g = 0; 00068 strip->leds[i].simple.r = 0; 00069 strip->leds[i].simple.b = 0; 00070 } 00071 } 00072 00073 void neopixel_clear(neopixel_strip_t *strip) 00074 { 00075 for (int i = 0; i < strip->num_leds; i++) 00076 { 00077 strip->leds[i].simple.g = 0; 00078 strip->leds[i].simple.r = 0; 00079 strip->leds[i].simple.b = 0; 00080 } 00081 neopixel_show(strip); 00082 } 00083 00084 void neopixel_show(neopixel_strip_t *strip) 00085 { 00086 const uint8_t PIN = strip->pin_num; 00087 NRF_GPIO->OUTCLR = (1UL << PIN); 00088 nrf_delay_us(50); 00089 for (int i = 0; i < strip->num_leds; i++) 00090 { 00091 for (int j = 0; j < 3; j++) 00092 { 00093 if ((strip->leds[i].grb[j] & 128) > 0) {NEOPIXEL_SEND_ONE} 00094 else {NEOPIXEL_SEND_ZERO} 00095 00096 if ((strip->leds[i].grb[j] & 64) > 0) {NEOPIXEL_SEND_ONE} 00097 else {NEOPIXEL_SEND_ZERO} 00098 00099 if ((strip->leds[i].grb[j] & 32) > 0) {NEOPIXEL_SEND_ONE} 00100 else {NEOPIXEL_SEND_ZERO} 00101 00102 if ((strip->leds[i].grb[j] & 16) > 0) {NEOPIXEL_SEND_ONE} 00103 else {NEOPIXEL_SEND_ZERO} 00104 00105 if ((strip->leds[i].grb[j] & 8) > 0) {NEOPIXEL_SEND_ONE} 00106 else {NEOPIXEL_SEND_ZERO} 00107 00108 if ((strip->leds[i].grb[j] & 4) > 0) {NEOPIXEL_SEND_ONE} 00109 else {NEOPIXEL_SEND_ZERO} 00110 00111 if ((strip->leds[i].grb[j] & 2) > 0) {NEOPIXEL_SEND_ONE} 00112 else {NEOPIXEL_SEND_ZERO} 00113 00114 if ((strip->leds[i].grb[j] & 1) > 0) {NEOPIXEL_SEND_ONE} 00115 else {NEOPIXEL_SEND_ZERO} 00116 } 00117 } 00118 } 00119 00120 uint8_t neopixel_set_color(neopixel_strip_t *strip, uint16_t index, uint8_t red, uint8_t green, uint8_t blue ) 00121 { 00122 if (index < strip->num_leds) 00123 { 00124 strip->leds[index].simple.r = red; 00125 strip->leds[index].simple.g = green; 00126 strip->leds[index].simple.b = blue; 00127 } 00128 else 00129 return 1; 00130 return 0; 00131 } 00132 00133 uint8_t neopixel_set_color_and_show(neopixel_strip_t *strip, uint16_t index, uint8_t red, uint8_t green, uint8_t blue) 00134 { 00135 if (index < strip->num_leds) 00136 { 00137 strip->leds[index].simple.r = red; 00138 strip->leds[index].simple.g = green; 00139 strip->leds[index].simple.b = blue; 00140 neopixel_show(strip); 00141 } 00142 else 00143 return 1; 00144 return 0; 00145 } 00146 00147 void neopixel_destroy(neopixel_strip_t *strip) 00148 { 00149 free(strip->leds); 00150 strip->num_leds = 0; 00151 strip->pin_num = 0; 00152 } 00153 /* 00154 int main() { 00155 DigitalOut mypin(D4); 00156 neopixel_strip_t m_strip; 00157 uint8_t dig_pin_num = P0_21; 00158 uint8_t leds_per_strip = 30; 00159 uint8_t error; 00160 uint8_t led_to_enable = 30; 00161 uint8_t red = 0; 00162 uint8_t green = 128; 00163 uint8_t blue = 128; 00164 00165 neopixel_init(&m_strip, dig_pin_num, leds_per_strip); 00166 neopixel_clear(&m_strip); 00167 //error = neopixel_set_color_and_show(&m_strip, led_to_enable, red, green, blue); 00168 for (int cv=0; cv < 60; cv++) { 00169 00170 neopixel_set_color_and_show(&m_strip, cv, red, green, blue); 00171 wait_ms(500); 00172 } 00173 00174 if (error) { 00175 //led_to_enable was not within number leds_per_strip 00176 } 00177 00178 //error = neopixel_set_color_and_show(&m_strip, 1, red, green, blue); 00179 while (1) { 00180 wait_ms(500); 00181 } 00182 //clear and remove strip 00183 //neopixel_clear(&m_strip); 00184 //neopixel_destroy(&m_strip); 00185 } 00186 */
Generated on Thu Jul 14 2022 06:29:37 by
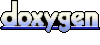