
Code used in the SICE E210/P442 lab practical
Dependencies: ADXL362 TSL2561 mbed
main.cpp
00001 // Code used for the SP18 E210/P442 Lab Practical 00002 #include "mbed.h" 00003 #include "ADXL362.h" 00004 #include "TSL2561.h" 00005 00006 AnalogOut my_output(PA_4); 00007 00008 // Interface pulled from ADXL362.cpp 00009 // ADXL362::ADXL362(PinName CS, PinName MOSI, PinName MISO, PinName SCK) : 00010 ADXL362 adxl362(PA_0,PA_7,PA_6,PA_1); 00011 TSL2561 lightsensor(PB_7,PB_6); 00012 00013 00014 #define PI (3.141592653589793238462) 00015 #define AMPLITUDE (1.0) // x * 3.3V 00016 #define PHASE (PI * 1) // 2*pi is one period 00017 #define RANGE (0x7FFF) 00018 #define OFFSET (0x7FFF) 00019 00020 #define SPI_CYCLES 100 00021 // Configuration for sinewave output 00022 #define BUFFER_SIZE (360) 00023 uint16_t buffer[BUFFER_SIZE]; 00024 00025 void calculate_sinewave(void); 00026 00027 int main() { 00028 printf("Sinewave example\n"); 00029 calculate_sinewave(); 00030 adxl362.reset(); 00031 wait_ms(1000); // we need to wait at least 500ms after ADXL362 reset 00032 adxl362.set_mode(ADXL362::MEASUREMENT); 00033 wait_ms(1000); // we need to wait at least 500ms after ADXL362 reset 00034 volatile int8_t x,y,z; 00035 volatile uint16_t whoami; 00036 volatile float light; 00037 int spi_trigger_target = 0; 00038 00039 while(1) { 00040 for (int i = 0; i < BUFFER_SIZE; i++) { 00041 my_output.write_u16(buffer[i]); 00042 wait_us(10); 00043 } 00044 x=adxl362.scanx_u8(); 00045 y=adxl362.scany_u8(); 00046 z=adxl362.scanz_u8(); 00047 if (spi_trigger_target++ == SPI_CYCLES){ 00048 spi_trigger_target = 0; 00049 whoami = adxl362.read_reg(ADXL362::DEVID_AD); 00050 } 00051 light = lightsensor.lux(); 00052 // printf("Accelerometer x = %d y = %d z = %d\r\n",x,y,z); 00053 // printf("Light sensor %f\n\r",lightsensor.lux()); 00054 // wait_ms(100); 00055 00056 } 00057 } 00058 00059 // Create the sinewave buffer 00060 void calculate_sinewave(void){ 00061 for (int i = 0; i < BUFFER_SIZE; i++) { 00062 double rads = (PI * i)/180.0; // Convert degree in radian 00063 buffer[i] = (uint16_t)(AMPLITUDE * (RANGE * (cos(rads + PHASE))) + OFFSET); 00064 } 00065 }
Generated on Sun Jul 17 2022 00:07:03 by
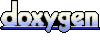