Homework_5_IOT
Dependencies: C12832_lcd EthernetInterface LCD_fonts LM75B MMA7660 NTPClient SimpleSMTPClient WebSocketClient mbed-rtos mbed
main.cpp
00001 /*******************************************************/ 00002 /* IMPLEMENT THE THERMOSTAT */ 00003 /*System turned ON/OFF using joystick or Accelerometer */ 00004 /*Temperature Set using Joystick */ 00005 /*System Status and heater state shown on LED */ 00006 /*LCD displays: Time. */ 00007 /* If system is ON current and set low */ 00008 /* and High Temperature */ 00009 /* Heater/Cooler ON */ 00010 /* If system is OFF SYSTEM OFF */ 00011 /*The timing, current temperature, accelerometer reading*/ 00012 /*are sent periodically to websockets.org/bhakti */ 00013 /*The duration after which the information is sent is */ 00014 /*defined by macro SECONDS which is currently set to 10*/ 00015 /*******************************************************/ 00016 00017 #include "mbed.h" 00018 #include "C12832_lcd.h" 00019 #include "LM75B.h" 00020 #include "DebouncedIn.h" 00021 #include "MMA7660.h" 00022 #include "EthernetInterface.h" 00023 #include "NTPClient.h" 00024 #include "SimpleSMTPClient.h" 00025 #include "Small_6.h" 00026 #include "Websocket.h" 00027 00028 #define hys 3 00029 #define HEATER 0 00030 #define COOLER 1 00031 #define No_HEAT_COOL 2 00032 #define SEND 1 00033 #define DO_NOT_SEND 0 00034 #define SECONDS 10 00035 00036 DebouncedIn reset(p14); 00037 C12832_LCD lcd; 00038 LM75B current(p28,p27); 00039 MMA7660 MMA(p28, p27); 00040 Serial pc(USBTX,USBRX); 00041 AnalogIn max_temp(p19); 00042 AnalogIn min_temp(p20); 00043 00044 //System ON/OFF 00045 BusIn heat_on (p16,p13); 00046 00047 //System ON/OFF LED 00048 DigitalOut thermostat (LED1); 00049 00050 //Heater/Cooler ON/OFF LED. This can be furthur connected to the relay 00051 DigitalOut heater (LED2); 00052 DigitalOut cooler (LED3); 00053 00054 Ticker check_move; 00055 Ticker Send_info; 00056 00057 bool no_move; //This variable is set if no movement is detected 00058 float acc_x = MMA.x(); 00059 float acc_y = MMA.y(); 00060 float acc_z = MMA.z(); 00061 float acc_x_old,acc_y_old,acc_z_old; 00062 int heat_cool; //1: Heater ON , 0:Cooler ON , 2: Heater and Cooler OFF 00063 bool send_info; //This variable is used to define when the information is 00064 //to be sent to the web. 00065 00066 /****************************************************************************/ 00067 /*Function name: check: This function is used to detect motion around the */ 00068 /*thermostat.It sets the variable no move if the previous and current */ 00069 /*value of the accelerometer value is same. Accelerometer value unchanged */ 00070 /*indicates that there is no motion around the thermostat */ 00071 /****************************************************************************/ 00072 void check() 00073 { 00074 acc_x_old = acc_x; 00075 acc_y_old = acc_y; 00076 acc_z_old = acc_z; 00077 acc_x = MMA.x(); 00078 acc_y = MMA.y(); 00079 acc_z = MMA.z(); 00080 if (acc_x_old == acc_x && acc_y_old == acc_y && acc_z_old == acc_z) 00081 { 00082 no_move = 1; 00083 } 00084 else 00085 no_move = 0; 00086 00087 } 00088 /******************************************************************************/ 00089 00090 /******************************************************************************/ 00091 /*Function name: send_ws() */ 00092 /*This function is used to set a boolean variable to SEND so that the */ 00093 /*information can be sent over the web. The variable is set to SEND after no. */ 00094 /*of seconds defined by SECONDS(currently 10sec) */ 00095 /******************************************************************************/ 00096 void send_ws() 00097 { 00098 send_info = SEND; 00099 } 00100 00101 /*******************************************************************************/ 00102 00103 int main() 00104 { 00105 int ret_msg,ret_msg2,ret_curr_time,eth_ret,ws_ret; 00106 EthernetInterface eth; 00107 lcd.cls(); 00108 pc.printf("\n\n/* SimpleMTPClient library demonstration \n"); 00109 00110 pc.printf("Setting up ...\n"); 00111 eth.init(); 00112 eth_ret = eth.connect(); 00113 if (eth_ret != 0) 00114 { 00115 lcd.cls(); 00116 lcd.printf("Ethernet not connected"); 00117 return -1; 00118 } 00119 pc.printf("Connected OK\n"); 00120 00121 // IP Address 00122 pc.printf("IP Address is %s\n", eth.getIPAddress()); 00123 lcd.locate(0,1); 00124 lcd.printf("%s", eth.getIPAddress()); 00125 wait(2); 00126 00127 00128 NTPClient ntp; 00129 ntp.setTime("time.nist.gov"); 00130 lcd.cls(); 00131 00132 Websocket ws("ws://sockets.mbed.org:443/ws/bhakti/rw"); 00133 ws_ret = ws.connect(); 00134 if (!ws_ret) 00135 { 00136 lcd.cls(); 00137 lcd.printf("Nor connected to URL"); 00138 return -1; 00139 } 00140 00141 /*Messages to be sent over the web*/ 00142 char msg[100]; 00143 char msg2[100]; 00144 char curr_time[100]; 00145 00146 float min_set; 00147 float max_set; 00148 00149 //This variable is used to check if the system is ON or OFF 00150 bool status=0; 00151 00152 time_t ctTime; 00153 lcd.cls(); 00154 check_move.attach(&check,120.0); 00155 Send_info.attach(&send_ws,SECONDS); 00156 while (1) { 00157 sprintf(msg,"Temperature is %0.2f",current.read()); 00158 sprintf(msg2,"Accelerometer readings are X= %0.2f,Y= %0.2f, Z= %0.2f",MMA.x(),MMA.y(),MMA.z()); 00159 sprintf(curr_time,"Time: %s",ctime(&ctTime)); 00160 if (send_info == SEND) { 00161 ret_msg2 = ws.send(msg2); 00162 ret_msg = ws.send(msg); 00163 ret_curr_time=ws.send(curr_time); 00164 if (ret_msg == -1 || ret_msg2 == -1 || ret_curr_time == -1) 00165 { 00166 lcd.cls(); 00167 lcd.printf("One of the messages not sent"); 00168 } 00169 send_info = DO_NOT_SEND; 00170 } 00171 min_set = min_temp.read() * 50; //scale the pot value from 0 to 1 -> 0 to 50 00172 max_set = max_temp.read() * 50; 00173 ctTime = time(NULL) - 25200; //Adjust time as per local time 00174 lcd.set_font((unsigned char*) Small_6); //set LCD font 00175 lcd.locate(1,1); 00176 lcd.printf("%s",ctime(&ctTime)); 00177 lcd.locate(1,8); 00178 00179 /*If system is ON print current and set temperature*/ 00180 /*Else print LCD OFF on LCD*/ 00181 00182 if (status) { 00183 lcd.printf("Curr:%.2f",current.read()); 00184 lcd.locate(1,16); 00185 lcd.printf("MAX:%0.2f, MIN:%0.2f",max_set,min_set); 00186 lcd.locate(1,24); 00187 switch (heat_cool) { 00188 case HEATER : lcd.printf("Heater ON "); 00189 break; 00190 case COOLER : lcd.printf("Cooler ON "); 00191 break; 00192 case No_HEAT_COOL : lcd.printf("Heat/Cool OFF"); 00193 break; 00194 } 00195 00196 } else { 00197 lcd.locate(1,10); 00198 lcd.printf("System OFF"); 00199 wait (0.1); 00200 } 00201 00202 /*If system is turned ON: Joystick is pushed towards edge of LCD 00203 OR movement is detected 00204 Turn System ON LED on: LED1 and Set the status variable to 1 00205 Else if system is turned OFF by user turn OFF the system status LED 00206 and the LED that is connected to the relay*/ 00207 00208 if (heat_on == 0x2 || !no_move) 00209 { 00210 thermostat = 1; 00211 status = 1; 00212 } else if (heat_on == 0x1 || no_move) { 00213 lcd.cls(); 00214 thermostat = 0; 00215 heater = 0; 00216 status = 0; 00217 } 00218 00219 //Comparison logic and turn Heater/Cooler ON/OFF 00220 if ((min_set > (current.read()+ hys)) && thermostat == 1) { 00221 heater = 1; 00222 cooler = 0; 00223 status = 1; 00224 heat_cool = HEATER; 00225 } 00226 else if ((max_set < (current.read()-hys)) && thermostat == 1) { 00227 cooler = 1; 00228 heater = 0; 00229 status = 1; 00230 heat_cool = COOLER; 00231 } 00232 else if (!thermostat) { 00233 heater = 0; 00234 cooler = 0; 00235 status = 0; 00236 } 00237 else { 00238 heater = 0; 00239 cooler = 0; 00240 status = 1; 00241 heat_cool = No_HEAT_COOL; 00242 } 00243 00244 } 00245 }
Generated on Fri Jul 22 2022 14:43:07 by
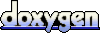