
IOT Project
Dependencies: WiflyInterface mbed
Fork of IOT-Websocket_Wifly_HelloWorld by
main.cpp
00001 //This Program is used to turn the LED ON/OFF via telent (Wifly connected) 00002 #include "mbed.h" 00003 #include "WiflyInterface.h" 00004 #include <string.h> 00005 00006 #define ECHO_SERVER_PORT 7 00007 #define FWD 8 00008 #define REV 4 00009 #define LEFT 3 00010 #define RIGHT 1 00011 #define STOP 2 00012 #define STRAIGHT_WHEEL 7 00013 00014 00015 PwmOut servo(p22); 00016 DigitalOut dir(LED1); 00017 BusOut motor(p5,p6,p7,p8); 00018 00019 //#define FWD 1 00020 //#define REV 0 00021 00022 00023 /* wifly interface: 00024 * - p9 and p10 are for the serial communication 00025 * - p19 is for the reset pin 00026 * - p26 is for the connection status 00027 * - "mbed" is the ssid of the network 00028 * - "password" is the password 00029 * - WPA is the security 00030 */ 00031 //apps board 00032 WiflyInterface wifly(p9, p10, p30, p29, "MY_WIFI", "", NONE); 00033 00034 //pololu 00035 //WiflyInterface wifly(p28, p27, p26, NC, "iotlab", "42F67YxLX4AawRdcj", WPA); 00036 00037 int main() { 00038 00039 wifly.init(); //Use DHCP 00040 printf("1\r\n"); 00041 while (!wifly.connect()); 00042 printf("IP Address is %s\n\r", wifly.getIPAddress()); 00043 00044 TCPSocketServer server; 00045 00046 server.bind(ECHO_SERVER_PORT); 00047 server.listen(); 00048 00049 printf("\nWait for new connection...\n"); 00050 TCPSocketConnection client; 00051 server.accept(client); 00052 00053 char buffer[256]; 00054 servo.period_us(50); 00055 motor = STOP; 00056 while (true) { 00057 //if (client.available()){ 00058 int n = client.receive(buffer, sizeof(buffer)); 00059 if (n <= 0) continue; 00060 buffer[n] = 0; 00061 printf("String is : %s\r\n",buffer); 00062 00063 client.send_all(buffer, n); 00064 if (!(strcmp (buffer, "w"))) 00065 motor = FWD; 00066 else if (!(strcmp(buffer,"x"))) 00067 motor = REV; 00068 else if (!(strcmp(buffer,"z"))) 00069 motor = STRAIGHT_WHEEL; 00070 else if (!(strcmp(buffer,"d"))) 00071 motor = RIGHT; 00072 else if (!(strcmp(buffer,"a"))) 00073 motor = LEFT; 00074 else if (!(strcmp(buffer,"s"))) 00075 motor = STOP; 00076 //} 00077 servo.pulsewidth_us(10); 00078 wait_us(1); 00079 } 00080 }
Generated on Fri Jul 15 2022 08:22:43 by
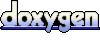