abc
Dependents: Microwave_MBED MicrowaveSimulation_LPC1768 RTOS_Alarm_Clock USB_Project_Host ... more
DebouncedInterrupt.cpp
00001 /** 00002 * DebouncedInterrupt.cpp 00003 **/ 00004 #include "DebouncedInterrupt.h" 00005 00006 Timeout timeout; 00007 00008 DebouncedInterrupt::DebouncedInterrupt(PinName pin) 00009 { 00010 _in = new InterruptIn(pin); 00011 _din = new DigitalIn(pin); 00012 } 00013 00014 DebouncedInterrupt::~DebouncedInterrupt() 00015 { 00016 delete _in; 00017 delete _din; 00018 } 00019 00020 void DebouncedInterrupt::attach(void (*fptr)(void), const unsigned int& debounce_ms) 00021 { 00022 if(fptr) { 00023 fCallback = fptr; 00024 _last_bounce_count = _bounce_count = 0; 00025 _debounce_us = 1000*debounce_ms; 00026 00027 _in->rise(this, &DebouncedInterrupt::_onInterrupt); 00028 _in->fall(this, &DebouncedInterrupt::_onInterrupt); 00029 } 00030 } 00031 00032 void DebouncedInterrupt::reset() 00033 { 00034 timeout.detach(); 00035 } 00036 00037 unsigned int DebouncedInterrupt::get_bounce() 00038 { 00039 return _last_bounce_count; 00040 } 00041 00042 void DebouncedInterrupt::_callback() 00043 { 00044 _last_bounce_count = _bounce_count; 00045 _bounce_count = 0; 00046 if(_din->read()) { 00047 fCallback(); 00048 } 00049 } 00050 00051 void DebouncedInterrupt::_onInterrupt() 00052 { 00053 _bounce_count++; 00054 timeout.attach_us(this, &DebouncedInterrupt::_callback, _debounce_us); 00055 }
Generated on Sun Jul 17 2022 20:34:59 by
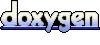