
TFT
Fork of Ovation_Controller_1 by
Embed:
(wiki syntax)
Show/hide line numbers
font_new.cpp
00001 #include "mbed.h" 00002 #include <stdio.h> 00003 #include "font_new.h" 00004 00005 void Write_Command(unsigned char command); 00006 void SendData(unsigned long color); 00007 void WindowSet(int s_x, int e_x,int s_y,int e_y); 00008 00009 void put_pixcels( int xs, int width, int ys, int height, unsigned char *c, int f_color, int b_color ); 00010 void put_pixcels_alpha( int xs, int width, int ys, int height, unsigned char *c, int color, int key ); 00011 00012 int FontDrawSpace( int width, int height, int xpos, int ypos, int f_color, int b_color ); 00013 00014 typedef struct _draw_font_parameters { 00015 font *font_ptr; 00016 int inter_char_space; 00017 int alpha_mode; 00018 int f_color; 00019 int b_color; 00020 } 00021 draw_font_parameters; 00022 draw_font_parameters dfp; 00023 00024 font Calibri14; 00025 font Calibri28; 00026 font Calibri72; 00027 font Calibri78_1; 00028 00029 void FontDrawInit( void ) 00030 { 00031 Calibri14.height = 23; 00032 Calibri14.max_width = 17; 00033 Calibri14.space_width = 7; 00034 Calibri14.data_table = c14_data_table; 00035 Calibri14.offset_table = c14_offset_table; 00036 Calibri14.index_table = c14_index_table; 00037 Calibri14.width_table = c14_width_table; 00038 00039 Calibri28.height = 45; 00040 Calibri28.max_width = 34; 00041 Calibri28.space_width = 10; 00042 Calibri28.data_table = c28_data_table; 00043 Calibri28.offset_table = c28_offset_table; 00044 Calibri28.index_table = c28_index_table; 00045 Calibri28.width_table = c28_width_table; 00046 00047 Calibri72.height = 117; 00048 Calibri72.max_width = 87; 00049 Calibri72.space_width = 20; 00050 Calibri72.data_table = c72_data_table; 00051 Calibri72.offset_table = c72_offset_table; 00052 Calibri72.index_table = c72_index_table; 00053 Calibri72.width_table = c72_width_table; 00054 00055 Calibri78_1.height = 117; 00056 Calibri78_1.max_width = 49; 00057 Calibri78_1.space_width = 20; 00058 Calibri78_1.data_table = c78_1_data_table; 00059 Calibri78_1.offset_table = c78_1_offset_table; 00060 Calibri78_1.index_table = c78_1_index_table; 00061 Calibri78_1.width_table = c78_1_width_table; 00062 00063 dfp.font_ptr = &Calibri14; 00064 dfp.inter_char_space = 0; 00065 dfp.alpha_mode = 0; 00066 dfp.f_color = 0x0000FF; 00067 dfp.b_color = 0xFFFFFF; 00068 00069 #if 0 00070 // printf( "c14_data_table : %p\r\n", c14_data_table ); 00071 // printf( "c14_data_table : %p\r\n", Calibri14.data_table ); 00072 // printf( "c14_offset_table : %p\r\n", Calibri14.offset_table ); 00073 // printf( "c14_index_table : %p\r\n", Calibri14.index_table ); 00074 // printf( "c14_width_table : %p\r\n", Calibri14.width_table ); 00075 00076 // printf( "(dfp.font_ptr->)data_table : %p\r\n", dfp.font_ptr->data_table ); 00077 #endif 00078 } 00079 00080 //#ifdef TEST 00081 //extern unsigned char screen[ SC_WIDTH * SC_HEIGHT ]; 00082 //#endif 00083 00084 void put_pixcels( int xs, int width, int ys, int height, unsigned char *c, int f_color, int b_color ) 00085 { 00086 int i; 00087 int j; 00088 00089 #ifdef TEST 00090 for ( j = 0; j < height; j++ ) 00091 for ( i = 0; i < width; i++ ) 00092 *(screen + ((((height - 1) - j) + ys) * SC_WIDTH) + i + xs) = (unsigned char)((*c++ == 'F') ? f_color : b_color); 00093 #else 00094 WindowSet( xs, (xs + width) - 1, ys, (ys + height) - 1 ); 00095 00096 Write_Command( 0x2c ); 00097 00098 for ( j = 0; j < height; j++ ) 00099 for ( i = 0; i < width; i++ ) 00100 SendData( (*c++ == 'F') ? f_color : b_color ); 00101 #endif 00102 } 00103 00104 00105 void put_pixcels_alpha( int xs, int width, int ys, int height, unsigned char *c, int color, int key ) 00106 { 00107 int i; 00108 int j; 00109 00110 #ifdef TEST 00111 00112 for ( j = 0; j < height; j++ ) 00113 { 00114 for ( i = 0; i < width; i++ ) 00115 { 00116 if ( *c == 'F' ) 00117 *(screen + ((((height - 1) - j) + ys) * SC_WIDTH) + i + xs) = color; 00118 c++; 00119 } 00120 } 00121 00122 #else 00123 00124 for ( j = 0; j < height; j++ ) 00125 { 00126 for ( i = 0; i < width; i++ ) 00127 { 00128 if ( *c != key ) 00129 { 00130 WindowSet( xs + i, xs + i, ys + j, ys + j ); 00131 Write_Command( 0x2c ); 00132 SendData( *c++ ); 00133 } 00134 } 00135 } 00136 00137 #endif 00138 00139 } 00140 00141 int FontDrawStringWidth( char *s, font *font_ptr ) 00142 { 00143 int str_width = 0; 00144 char c; 00145 00146 while ( c = *s++ ) 00147 { 00148 if ( ' ' == c ) 00149 { 00150 str_width += font_ptr->space_width; 00151 } 00152 else 00153 { 00154 str_width += font_ptr->width_table[ font_ptr->index_table[ c ] ]; 00155 00156 if ( dfp.inter_char_space ) 00157 str_width += dfp.inter_char_space; 00158 } 00159 } 00160 00161 return ( str_width ); 00162 } 00163 00164 00165 unsigned char pad[ 90 * 120 ]; 00166 00167 00168 int FontDrawChar( char c, int xpos, int ypos, int f_color, int b_color, font *font_ptr ) 00169 { 00170 unsigned char index; 00171 unsigned int offset; 00172 int width; 00173 int height; 00174 unsigned char *data; 00175 int i; 00176 int j; 00177 00178 index = font_ptr->index_table[ c ]; 00179 offset = font_ptr->offset_table[ index ]; 00180 00181 height = font_ptr->height; 00182 00183 if ( ' ' == c ) 00184 return ( FontDrawSpace( font_ptr->space_width, height, xpos, ypos, f_color, b_color ) ); 00185 00186 width = font_ptr->width_table[ index ]; 00187 00188 //#if 0 00189 // printf( "char = '%c' on %d / %d - %d / %d - %p\r\n", c, xpos, ypos, f_color, b_color, font_ptr ); 00190 // printf( "c14_data_table : %p\r\n", c14_data_table ); 00191 // printf( "c14_offset_table : %p\r\n", c14_offset_table ); 00192 // printf( "c14_index_table : %p\r\n", c14_index_table ); 00193 // printf( "c14_width_table : %p\r\n", c14_width_table ); 00194 // printf( "(dfp.font_ptr->)data_table : %p\r\n", dfp.font_ptr->data_table ); 00195 // printf( "font_ptr->height : %p\r\n", font_ptr->height ); 00196 // printf( "font_ptr->max_width : %p\r\n", font_ptr->max_width ); 00197 // printf( "font_ptr->space_width : %p\r\n", font_ptr->space_width ); 00198 // printf( "font_ptr->data_table : %p\r\n", font_ptr->data_table ); 00199 // printf( "font_ptr->offset_table : %p (%p)\r\n", font_ptr->offset_table, font_ptr->offset_table - (unsigned int *)font_ptr->data_table ); 00200 // printf( "font_ptr->index_table : %p (%p)\r\n", font_ptr->index_table, font_ptr->index_table - font_ptr->data_table ); 00201 // printf( "font_ptr->width_table : %p (%p)\r\n", font_ptr->width_table, font_ptr->width_table - font_ptr->data_table ); 00202 // printf( "index = %u\r\n", index ); 00203 // printf( "offset = %u\r\n", offset ); 00204 // printf( "width = %u\r\n", width ); 00205 // printf( "height = %u\r\n", height ); 00206 // printf( "\r\n" ); 00207 //#endif 00208 data = (unsigned char *)(&(font_ptr->data_table[ offset ])); 00209 00210 //#define CHAR_FLIP_NORMAL_HORIZONTAL 00211 00212 #define CHAR_FLIP_NORMAL_VERTICAL 00213 00214 for ( i = 0; i < width; i++ ) 00215 { 00216 for ( j = 0; j < height; j++ ) 00217 { 00218 #if defined( CHAR_FLIP_NORMAL_HORIZONTAL ) && !defined( CHAR_FLIP_NORMAL_VERTICAL ) 00219 *(pad + (j * width) + ((width - 1) - i)) = (*(data + (i * ((height + 7) / 8)) + (j / 8)) << (j % 8)) & 0x80 ? 'F' : 'B'; 00220 #elif !defined( CHAR_FLIP_NORMAL_HORIZONTAL ) && defined( CHAR_FLIP_NORMAL_VERTICAL ) 00221 *(pad + (((height - 1) - j) * width) + i) = (*(data + (i * ((height + 7) / 8)) + (j / 8)) << (j % 8)) & 0x80 ? 'F' : 'B'; 00222 #elif defined( CHAR_FLIP_NORMAL_HORIZONTAL ) && defined( CHAR_FLIP_NORMAL_VERTICAL ) 00223 *(pad + (((height - 1) - j) * width) + ((width - 1) - i)) = (*(data + (i * ((height + 7) / 8)) + (j / 8)) << (j % 8)) & 0x80 ? 'F' : 'B'; 00224 #else 00225 *(pad + (j * width) + i) = (*(data + (i * ((height + 7) / 8)) + (j / 8)) << (j % 8)) & 0x80 ? 'F' : 'B'; 00226 #endif 00227 00228 00229 00230 #if 0 00231 #if 0 00232 *(pad + (j * width) + i) = (*(data + (i * ((height + 7) / 8)) + (j / 8)) << (j % 8)) & 0x80 ? 'F' : 'B'; 00233 #else 00234 *(pad + (((height - 1) - j) * width) + i) = (*(data + (i * ((height + 7) / 8)) + (j / 8)) << (j % 8)) & 0x80 ? 'F' : 'B'; 00235 #endif 00236 #endif 00237 } 00238 } 00239 00240 if ( dfp.alpha_mode ) 00241 put_pixcels_alpha( xpos, width, ypos, height, pad, f_color, b_color ); 00242 else 00243 put_pixcels( xpos, width, ypos, height, pad, f_color, b_color ); 00244 00245 if ( dfp.inter_char_space ) 00246 width += FontDrawSpace( dfp.inter_char_space, height, xpos + width, ypos, f_color, b_color ); 00247 00248 return ( width ); 00249 } 00250 00251 00252 int FontDrawSpace( int width, int height, int xpos, int ypos, int f_color, int b_color ) 00253 { 00254 int i; 00255 unsigned char pad[ width * height ]; 00256 00257 if ( dfp.alpha_mode ) 00258 return ( width ); 00259 00260 for ( i = 0; i < width * height; i++ ) 00261 *(pad + i) = b_color; 00262 00263 put_pixcels( xpos, width, ypos, height, pad, f_color, b_color ); 00264 00265 return ( width ); 00266 } 00267 00268 00269 void FontDrawString( char *s, int xpos, int ypos, int f_color, int b_color, font *font_ptr ) 00270 { 00271 char c; 00272 00273 while ( c = *s++ ) 00274 xpos += FontDrawChar( c, xpos, ypos, f_color, b_color, font_ptr ); 00275 } 00276 00277 void FontDraw_SetInterCharSpace( int space ) 00278 { 00279 dfp.inter_char_space = space; 00280 } 00281 00282 00283 void FontDraw_SetAlphaMode( int mode ) 00284 { 00285 dfp.alpha_mode = mode; 00286 } 00287 00288 void FontDraw_SetFont( font f ) 00289 { 00290 dfp.font_ptr = &f; 00291 } 00292 00293 void FontDraw_SetForegroundColor( int v ) 00294 { 00295 dfp.f_color = v; 00296 } 00297 00298 void FontDraw_SetBackgroundColor( int v ) 00299 { 00300 dfp.b_color = v; 00301 } 00302 00303 void FontDraw_puts( int x, int y, char *s ) 00304 { 00305 FontDrawString( s, x, y, dfp.f_color, dfp.b_color, dfp.font_ptr ); 00306 } 00307 00308 00309 #include <stdarg.h> 00310 00311 void FontDraw_printf( int x, int y, char *format, ... ) 00312 { 00313 char s[ 80 ]; 00314 va_list args; 00315 00316 va_start( args, format ); 00317 vsnprintf( s, 32, format, args ); 00318 va_end( args ); 00319 00320 FontDraw_puts( x, y, s ); 00321 }
Generated on Thu Jul 14 2022 01:02:03 by
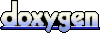