
FOC Implementation for putting multirotor motors in robots
Embed:
(wiki syntax)
Show/hide line numbers
SVM.cpp
00001 00002 #include "mbed.h" 00003 #include "SVM.h" 00004 #include "Inverter.h" 00005 #define min(x,y,z) (x < y ? (x < z ? x : z) : (y < z ? y : z)) 00006 #define max(x,y,z) (x > y ? (x > z ? x : z) : (y > z ? y : z)) 00007 00008 SPWM::SPWM(Inverter *inverter, float V_Bus){ 00009 _inverter = inverter; 00010 _V_Bus = V_Bus; 00011 } 00012 00013 //sinusoidal PWM 00014 void SPWM::Update_DTC(float V_A, float V_B, float V_C){ 00015 float DTC_A = V_A/_V_Bus + .5f; 00016 float DTC_B = V_B/_V_Bus + .5f; 00017 float DTC_C = V_C/_V_Bus + .5f; 00018 00019 if(DTC_A > .95f) DTC_A = .95f; 00020 else if(DTC_A < .05f) DTC_A = .05f; 00021 if(DTC_B > .95f) DTC_B = .95f; 00022 else if(DTC_B < .05f) DTC_B = .05f; 00023 if(DTC_C > .95f) DTC_C = .95f; 00024 else if(DTC_C < .05f) DTC_C = .05f; 00025 _inverter->SetDTC(DTC_A, DTC_B, DTC_C); 00026 } 00027 00028 SVPWM::SVPWM(Inverter *inverter, float V_Bus){ 00029 _inverter = inverter; 00030 _V_Bus = V_Bus; 00031 } 00032 00033 //space vector pwm (better bus utilization) 00034 void SVPWM::Update_DTC(float V_A, float V_B, float V_C){ 00035 00036 float Voff = (min(V_A, V_B, V_C) + max(V_A, V_B, V_C))/2.0f; 00037 00038 V_A = V_A - Voff; 00039 V_B = V_B - Voff; 00040 V_C = V_C - Voff; 00041 00042 float DTC_A = V_A/_V_Bus + .5f; 00043 float DTC_B = V_B/_V_Bus + .5f; 00044 float DTC_C = V_C/_V_Bus + .5f; 00045 00046 00047 00048 if(DTC_A > .95f) DTC_A = .95f; 00049 else if(DTC_A < .05f) DTC_A = .05f; 00050 if(DTC_B > .95f) DTC_B = .95f; 00051 else if(DTC_B < .05f) DTC_B = .05f; 00052 if(DTC_C > .95f) DTC_C = .95f; 00053 else if(DTC_C < .05f) DTC_C = .05f; 00054 _inverter->SetDTC(DTC_A, DTC_B, DTC_C); 00055 } 00056
Generated on Wed Jul 13 2022 02:31:45 by
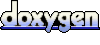