
Universal Translator
Dependencies: EthernetNetIf TextLCD mbed PS2 HTTPClient
main.cpp
00001 #include "mbed.h" 00002 #include "UnivTrans.h" 00003 00004 TextLCD lcd(p14, p16, p17, p18, p19, p20); // rs, e, d4-d7 00005 DigitalOut led1(LED1); 00006 DigitalOut led2(LED2); 00007 DigitalOut led3(LED3); 00008 DigitalOut led4(LED4); 00009 00010 EthernetNetIf eth; // Ethernet Control 00011 HTTPClient http; // HTTP Client Stack Library 00012 00013 VS1002 mp3( // Initialize MP3 Hardware Decoder class 00014 p5, p6, p7, p8, "sd", // SD Card SPI Connections & Setup 00015 p11, p12, p13, p10, // VS1053/1002 SPI Connections 00016 p21, p22, p23, p15); // DREQ,DCS,VOL 00017 // Due to the current TextLCD setup, the only Analog pin 00018 // available is p15, thus it is used for volume control 00019 00020 int main() { 00021 Serial pc(USBTX, USBRX); 00022 PS2Keyboard KeyB(p29, p30); // CLK, DAT 00023 Ticker tm; // Controls Blinking of LEDs/consistent ops 00024 00025 // Start Setting Up Hardware 00026 printf("Setting up...\n"); 00027 EthernetErr ethErr = eth.setup(); 00028 if(ethErr) { 00029 printf("Error %d in setup.\n", ethErr); 00030 return -1; 00031 } 00032 printf("Setup OK\n"); 00033 00034 // Initialize MP3 Hardware 00035 #ifndef FS_ONLY 00036 mp3._RST = 1; 00037 mp3.cs_high(); //chip disabled 00038 mp3.sci_initialise(); //initialise MBED 00039 mp3.sci_write(0x00,(SM_SDINEW+SM_STREAM+SM_DIFF)); 00040 mp3.sci_write(0x03, 0x9800); 00041 mp3.sdi_initialise(); 00042 #endif 00043 00044 //Blink now to signal successful hardware/internet setup 00045 tm.attach(&blink,.5); 00046 00047 //Now let's get to the real code 00048 00049 char rowstring[6*16]; 00050 memset(rowstring,0,6*16); 00051 00052 while(true){ 00053 checkKeys(KeyB,rowstring); 00054 Net::poll(); 00055 } 00056 00057 return 0; 00058 }
Generated on Sat Jul 16 2022 22:03:47 by
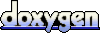