Library for the I2C connected LCD display EA DOGM204.
Embed:
(wiki syntax)
Show/hide line numbers
lcd_DOGM204_i2c.h
00001 /** EA DOGM204 LCD header file 00002 * 00003 * Provides access to the I2C connected Electronic Assembly DOGM204 LCD display 00004 * with SSD1803A controller (www.lcd-module.de) 00005 * 00006 * Version: 1.00.000 00007 * Date : 02.01.2020 00008 * Author: Marjan Hanc, www.m-hub.eu 00009 * 00010 * Note: This library does not support SPI and 4/8-Bit I/O modes of display. 00011 * It is assumed, that the RS address selection pin is statically connected 00012 * either to the GND (SA0=0) or VCC (SA0=1). 00013 **/ 00014 00015 #ifndef MBED_LCD_DOGM204 00016 #define MBED_LCD_DOGM204 00017 00018 #include "mbed.h" 00019 00020 class DOGM204I2C { 00021 00022 public: 00023 00024 enum LCD_Commands { 00025 LCD_ADR = 0x78, // SA0=0, 0x7A when SA0=1 00026 LCD_CLEAR = 0x00, // Clear Display 00027 LCD_RTHOME = 0x02 // Return home 00028 }; 00029 00030 enum LCD_Status { 00031 LCD_STATUS = 0x00, 00032 LCD_DATA = 0x40, 00033 LCD_BUSY = 0x80 00034 }; 00035 00036 enum LCD_Charset { 00037 LCD_ROMA = 0x00, 00038 LCD_ROMB = 0x04, 00039 LCD_ROMC = 0x0C 00040 }; 00041 00042 enum LCD_Mode { 00043 LCD_CURSOR_LINE = 0x00, 00044 LCD_CURSOR_BLOCK = 0x02, 00045 LCD_TOPVIEW = 0x05, 00046 LCD_BOTVIEW = 0x06, 00047 LCD_2LINE_MODE = 0x08, // Base setting for 1 & 2 line mode 00048 LCD_4LINE_MODE = 0x09, // Base setting for 3 & 4 line mode 00049 LCD_FONT_5DOT = 0x00, 00050 LCD_FONT_6DOT = 0x04 00051 }; 00052 00053 enum LCD_Settings { 00054 LCD_DISPLAY_ON = 0x04, 00055 LCD_DISPLAY_OFF = 0x03, 00056 LCD_CURSOR_ON = 0x02, 00057 LCD_CURSOR_OFF = 0x05, 00058 LCD_BLINK_ON = 0x01, 00059 LCD_BLINK_OFF = 0x06 00060 }; 00061 00062 enum LCD_Positions { 00063 LCD_HOME = 0x80, 00064 LCD_LINE1 = 0x00, 00065 LCD_LINE2 = 0x20, 00066 LCD_LINE3 = 0x40, 00067 LCD_LINE4 = 0x60 00068 }; 00069 00070 enum Frequency { 00071 Frequency_100KHz = 100000, 00072 Frequency_400KHz = 400000 00073 }; 00074 00075 DOGM204I2C(PinName sda, PinName scl, char SA, int frequency); 00076 00077 void display_set(char mode); 00078 void set_pos(char pos); 00079 void write_char(char line, char pos, char ch); 00080 void write(char *s); 00081 void cls(); 00082 void init(); 00083 00084 private: 00085 char _baseAdr; // LCD base address (0x78 or 0x7A, depends on SA0) 00086 00087 I2C i2c; // I2C interface 00088 00089 bool lcd_i2c_write(char cmd, char dta); 00090 char lcd_i2c_read(char cmd); 00091 bool lcd_write_cmd(char data); 00092 bool lcd_write_data(char data); 00093 }; 00094 00095 00096 #endif
Generated on Sat Jul 16 2022 14:27:04 by
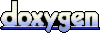