Library for the I2C connected LCD display EA DOGM204.
Embed:
(wiki syntax)
Show/hide line numbers
lcd_DOGM204_i2c.cpp
00001 /** EA DOGM204 LCD class 00002 * 00003 * Provides access to the I2C connected Electronic Assembly DOGM204 LCD display 00004 * with SSD1803A controller (www.lcd-module.de) 00005 * 00006 * Version: 1.00.000 00007 * Date : 02.01.2020 00008 * Author: Marjan Hanc, www.m-hub.eu 00009 * 00010 * Note: This library does not support SPI and 4/8-Bit I/O modes of the display. 00011 * It is assumed, that the RS address selection pin is statically connected 00012 * either to the GND (SA=0) or VCC (SA=1). 00013 **/ 00014 00015 #include "lcd_DOGM204_i2c.h" 00016 #include "mbed.h" 00017 00018 /*\brief Initialises the LCD with respective I2C interface 00019 \param sda I2C SDA pin mapping 00020 \param scl I2C SCL pin mapping 00021 \param SA SA0 (selector) address of the LCD display 00022 \param freq Frequency of the I2C clock 00023 */ 00024 00025 DOGM204I2C::DOGM204I2C(PinName sda, PinName scl, char SA, int frequency) : i2c(sda,scl) { 00026 00027 if (SA > 1) 00028 error("DOGM204I2C: SA is out of range, must be 0..1\n"); 00029 else 00030 _baseAdr = LCD_ADR + (SA << 1); // sets the base address of the LCD 00031 00032 if (frequency > Frequency_400KHz) 00033 error("DOGM204I2C: I2C frequency out of range, must be less than 400 kHz\n"); 00034 00035 i2c.frequency(frequency); 00036 } 00037 00038 /*\brief Sends command with one data byte to the LCD 00039 \param cmd LCD command 00040 \param dta Data byte 00041 \return status Returns true, if the command was successful 00042 */ 00043 00044 bool DOGM204I2C::lcd_i2c_write(char cmd, char dta) 00045 { 00046 char data[] = {cmd, dta}; 00047 00048 if (i2c.write(_baseAdr, data, 2)) { 00049 00050 return false; 00051 } 00052 00053 return true; 00054 } 00055 00056 /*\brief Reads one byte (command status) of the LCD 00057 \param cmd LCD command 00058 \return data Data byte 00059 */ 00060 00061 char DOGM204I2C::lcd_i2c_read(char cmd) 00062 { 00063 char data[] = {cmd}; 00064 00065 i2c.write(_baseAdr, data, 1); // note, that the base address occupies bits b1..b7 00066 i2c.read(_baseAdr + 1, data, 1); // and that the b0 is R=1/W=0 00067 00068 return(data[0]); 00069 } 00070 00071 /*\brief Writes single command to the LCD by checking the display status first 00072 \note The command is not fail safe. If the display doesn't return "not busy" it might hang forever 00073 \param cmd LCD command 00074 \return stat Returns true if successful 00075 */ 00076 00077 bool DOGM204I2C::lcd_write_cmd(char data) 00078 { 00079 //check and wait, if LCD is busy 00080 while(lcd_i2c_read(LCD_STATUS) & LCD_BUSY); 00081 00082 // write command, control byte C0=0 & D/C = 0 00083 return lcd_i2c_write(0x80, data); 00084 } 00085 00086 /*\brief Writes data byte to the LCD by checking the display's busy flag BF first 00087 \note The command is not fail safe. If the display doesn't return "not busy" it might hang forever. 00088 \param data LCD data 00089 \return stat Returns true if successful 00090 */ 00091 bool DOGM204I2C::lcd_write_data(char data) 00092 { 00093 //check and wait, if LCD is busy 00094 while(lcd_i2c_read(LCD_STATUS) & LCD_BUSY); 00095 //write data, control byte C0=0 & D/C = 1 00096 return lcd_i2c_write(0x40, data); 00097 } 00098 00099 // -- Public functions -- 00100 00101 /* \brief Sets the LCD display to the provided mode 00102 * \param mode LCD_DISPLAY_ON|OFF LCD_CURSOR_ON|OFF LCD_BLINK_ON|OFF 00103 */ 00104 void DOGM204I2C::display_set(char mode) 00105 { 00106 if (!lcd_write_cmd(0x08+mode)) 00107 error("DOGM204I2C: Write command in lcd_display_set() failed!\n"); 00108 } 00109 00110 /* \brief Sets the cursor to the given position counted from the origin 00111 * \param pos position 00112 */ 00113 void DOGM204I2C::set_pos(char pos) 00114 { 00115 if (!lcd_write_cmd(LCD_HOME+pos)) 00116 error("DOGM204I2C: Write command in lcd_set_pos() failed!\n"); 00117 } 00118 00119 /* \brief Writes a single character at given position in the given line 00120 * \param pos LCD_LINEx+position 00121 */ 00122 void DOGM204I2C::write_char(char line, char pos, char ch) 00123 { 00124 char lcdpos = pos + 0x20 * line; 00125 00126 if (!lcd_write_cmd(lcdpos)) 00127 error("DOGM204I2C: Write command in lcd_write_char() failed!\n"); 00128 else 00129 if (!lcd_write_data(ch)) 00130 error("DOGM204I2C: Write data in lcd_write_char() failed!\n"); 00131 } 00132 00133 /* \brief Writes null terminated string to the LCD 00134 * \param s Null terminated string 00135 */ 00136 void DOGM204I2C::write(char *s) 00137 { 00138 while(*s) 00139 { 00140 if (!lcd_write_data(*s++)) { 00141 error("DOGM204I2C: Write data in lcd_write() failed!\n"); 00142 break; 00143 } 00144 } 00145 } 00146 00147 /* \brief Clears the LCD display and sets the cursor to the top-left position 00148 */ 00149 void DOGM204I2C::cls() 00150 { 00151 lcd_write_cmd(LCD_CLEAR); // Clear display 00152 lcd_write_cmd(LCD_RTHOME); // Set cursor position to home (top left) 00153 } 00154 00155 /* \brief Initializes LCD display 00156 * \note This sequence will set 4 lines, 5 dots, and top orientation at maximum 00157 contrast with cursor off 00158 */ 00159 void DOGM204I2C::init() 00160 { 00161 lcd_write_cmd(0x3A); // 8-Bit data length, extension Bit RE=1; REV=0 00162 lcd_write_cmd(LCD_4LINE_MODE | LCD_FONT_5DOT ); // 4 lines, 5 dots charset 00163 00164 lcd_write_cmd(0x80); // Pixel shift 0 00165 00166 lcd_write_cmd(LCD_TOPVIEW); // Set LCD orientation 00167 lcd_write_cmd(0x1E); // Bias setting BS1=1 00168 00169 lcd_write_cmd(0x39); // 8-Bit data length extension Bit RE=0; IS=1 00170 lcd_write_cmd(0x1B); // BS0=1 -> Bias=1/6 00171 00172 lcd_write_cmd(0x6E); // Divider ON and set value 00173 lcd_write_cmd(0x57); // Booster ON and set contrast (BB1=C5, DB0=C4) 00174 lcd_write_cmd(0x7B); // Set optimum contrast (DB3-DB0=C3-C0) 00175 00176 lcd_write_cmd(0x38); // 8-Bit data length extension Bit RE=0; IS=0 00177 } 00178
Generated on Sat Jul 16 2022 14:27:04 by
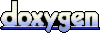