SPI SRAM 23X library
Embed:
(wiki syntax)
Show/hide line numbers
sram23x.h
00001 #ifndef __SRAM23X__H_ 00002 #define __SRAM23X__H_ 00003 00004 // Includes 00005 #include <string> 00006 00007 #include "mbed.h" 00008 00009 // Example 00010 /* 00011 */ 00012 00013 // Defines 00014 #define SRAM23X_READ 0x03 00015 #define SRAM23X_WRITE 0x02 00016 #define SRAM23X_RDSR 0x05 00017 #define SRAM23X_WRSR 0x01 00018 #define SRAM23X_SEQ 0x40 00019 00020 #define SRAM23X_NoError 0x00 00021 #define SRAM23X_OutOfRange 0x01 00022 #define SRAM23X_CsError 0x02 00023 #define SRAM23X_MallocError 0x03 00024 00025 #define SRAM23X_MaxError 4 00026 00027 static std::string _ErrorMessageSRAM23X[SRAM23X_MaxError] = { 00028 "", 00029 "Data address out of range" 00030 "Chip select error", 00031 "Memory allocation error" 00032 }; 00033 00034 // Class 00035 class SRAM23X { 00036 public: 00037 enum TypeSram {S23X640=8192,S23X256=32768} Type; 00038 00039 /* 00040 * Constructor, initialize the sram on spi interface. 00041 * @param mosi : mosi spi pin (PinName) 00042 * @param miso : miso spi pin (PinName) 00043 * @param sclk : sclk spi pin (PinName) 00044 * @param cs : chip select pin (PinName) 00045 * @param type : sram type (TypeSram) 00046 * @return none 00047 */ 00048 SRAM23X(PinName mosi, PinName miso, PinName sclk, PinName cs, TypeSram type); 00049 00050 /* 00051 * Sequential read bytes 00052 * @param address : start address (uint16_t) 00053 * @param data : bytes array to read (int8_t *) 00054 * @param size : number of bytes to read (uint16_t) 00055 * @return none 00056 */ 00057 void read(uint16_t address, int8_t *data, uint16_t size); 00058 00059 /* 00060 * Sequential read anything 00061 * @param address : start address (uint16_t) 00062 * @param data : data array to read (void *) 00063 * @param size : number of bytes to read (uint16_t) 00064 * @return none 00065 */ 00066 void read(uint16_t address, void *data, uint16_t size); 00067 00068 /* 00069 * Sequential read int16 00070 * @param address : start address (uint16_t) 00071 * @param data : short array to read (int16_t *) 00072 * @param size : number of bytes to read (uint16_t) 00073 * @return none 00074 */ 00075 void read(uint16_t address, int16_t *data, uint16_t size); 00076 00077 /* 00078 * Sequential read int32 00079 * @param address : start address (uint16_t) 00080 * @param data : int array to read (int32_t *) 00081 * @param size : number of bytes to read (uint16_t) 00082 * @return none 00083 */ 00084 void read(uint16_t address, int32_t *data, uint16_t size); 00085 00086 /* 00087 * Sequential read float 00088 * @param address : start address (uint16_t) 00089 * @param data : float array to read (float *) 00090 * @param size : number of bytes to read (uint16_t) 00091 * @return none 00092 */ 00093 void read(uint16_t address, float *data, uint16_t size); 00094 00095 /* 00096 * Sequential write bytes 00097 * @param address : start address(uint16_t) 00098 * @param data : bytes array to write (int8_t *) 00099 * @param size : number of bytes to write (uint16_t) 00100 * @return none 00101 */ 00102 void write(uint16_t address, int8_t *data, uint16_t size); 00103 00104 /* 00105 * Sequential write anything 00106 * @param address : start address (uint16_t) 00107 * @param data : data array to write (void *) 00108 * @param size : number of bytes to write (uint16_t) 00109 * @return none 00110 */ 00111 void write(uint16_t address, void *data, uint16_t size); 00112 00113 /* 00114 * Sequential write int16 00115 * @param address : start address (uint16_t) 00116 * @param data : short array to write (int8_t *) 00117 * @param size : number of bytes to write (uint16_t) 00118 * @return none 00119 */ 00120 void write(uint16_t address, int16_t *data, uint16_t size); 00121 00122 /* 00123 * Sequential write int32 00124 * @param address : address to write (uint16_t) 00125 * @param data : int array to write (int32_t *) 00126 * @param size : number of bytes to write (uint16_t) 00127 * @return none 00128 */ 00129 void write(uint16_t address, int32_t *data, uint16_t size); 00130 00131 /* 00132 * Sequential write float 00133 * @param address : address to write (uint16_t) 00134 * @param data : float array to write (float *) 00135 * @param size : number of bytes to write (uint16_t) 00136 * @return none 00137 */ 00138 void write(uint16_t address, float *data, uint16_t size); 00139 00140 /* 00141 * Get sram size in bytes 00142 * @param : none 00143 * @return size in bytes (uint16_t) 00144 */ 00145 uint16_t getSize(void); 00146 00147 /* 00148 * Select chip 00149 * @param : none 00150 * @return none 00151 */ 00152 void select(void); 00153 00154 /* 00155 * Deselect chip 00156 * @param : none 00157 * @return none 00158 */ 00159 void deselect(void); 00160 00161 /* 00162 * Read status register 00163 * @param : none 00164 * @return status register value (uint8_t) 00165 */ 00166 uint8_t readStatus(void); 00167 00168 /* 00169 * Write status register 00170 * @param : status value (uint8_t) 00171 * @return none 00172 */ 00173 void writeStatus(uint8_t status); 00174 00175 /* 00176 * Get current error message 00177 * @param : none 00178 * @return current error message(std::string) 00179 */ 00180 std::string getErrorMessage(void) 00181 { 00182 return(_ErrorMessageSRAM23X[_errnum]); 00183 } 00184 00185 /* 00186 * Get the current error number (SRAM23X_NoError if no error) 00187 * @param : none 00188 * @return current error number (uint8_t) 00189 */ 00190 uint8_t getError(void); 00191 00192 //---------- local variables ---------- 00193 private: 00194 SPI _spi; // Local spi communication interface instance 00195 DigitalOut *_cs; // Chip select 00196 uint8_t _errnum; // Error number 00197 TypeSram _type; // SRAM type 00198 uint16_t _size; // Size in bytes 00199 bool checkAddress(uint16_t address); // Check address range 00200 //------------------------------------- 00201 00202 /* 00203 * Initialization 00204 * @param address : address to write (uint16_t) 00205 * @param size : size to write in bytes (uint16_t) 00206 * @param mode : read or write (uint8_t) 00207 * @return error code, 0 if no error 00208 */ 00209 uint8_t init(uint16_t address, uint16_t size, uint8_t mode); 00210 }; 00211 #endif
Generated on Wed Jul 20 2022 13:32:55 by
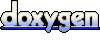