
倒立振子ロボットで使用してるユニポーラのステッピングモータの駆動テストプログラムです。
Embed:
(wiki syntax)
Show/hide line numbers
StepperMotor.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 00020 #ifndef MBED_STEPPER_MOTOR_H 00021 #define MBED_STEPPER_MOTOR_H 00022 00023 #include "mbed.h" 00024 00025 typedef enum {PWM_ON, PWM_OFF} pwm_state; 00026 typedef enum {CLOCK_WISE, ANTI_CLOCK_WISE} motor_dir; 00027 00028 struct speed_data_t { 00029 int max_pulse_count; 00030 int pwm_ratio; 00031 uint8_t *clockwise_ptn; 00032 uint8_t *anticlockwise_ptn; 00033 int ptn_count; 00034 }; 00035 00036 #define PULSE_INTERVAL 14 // 13us 00037 00038 class StepperMotor 00039 { 00040 public: 00041 StepperMotor(PinName x_pin_no, PinName y_pin_no, PinName nx_pin_no, PinName ny_pin_no); 00042 ~StepperMotor(); 00043 00044 void SetSpeed(int speed, motor_dir direction); 00045 void PulseEnable(void); 00046 void PulseDisable(void); 00047 00048 private: 00049 pwm_state state; 00050 motor_dir direction; 00051 int ptn_index; 00052 int ptn_count; 00053 int pwm_ratio; 00054 int pwm_on_count; 00055 int pwm_off_count; 00056 int max_pulse_count; 00057 int pulse_count; 00058 uint8_t *ptn; 00059 uint8_t ptn_data; 00060 00061 DigitalOut _x; 00062 DigitalOut _y; 00063 DigitalOut _nx; 00064 DigitalOut _ny; 00065 00066 Ticker Pulse; 00067 00068 void SetPulse14us(void); 00069 void PulseOut(void); 00070 void PulseStop(void); 00071 }; 00072 00073 #endif
Generated on Wed Jul 20 2022 12:05:17 by
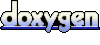