Orignal AVR Tiny USI Based I2C slave LCD Driver.
Dependents: MyLCD_OSARU MyLCD_printf
MyLCD.h
00001 /** 00002 ***************************************************************************** 00003 * File Name : MyLCD.h 00004 * 00005 * Title : ORGINAL I2C LCD Display Claass Header File 00006 * Revision : 0.1 00007 * Notes : 00008 * Target Board : mbed NXP LPC1768, mbed LPC1114FN28 etc 00009 * Tool Chain : ???? 00010 * 00011 * Revision History: 00012 * When Who Description of change 00013 * ----------- ----------- ----------------------- 00014 * 2013/12/06 Hiroshi M init 00015 ***************************************************************************** 00016 * 00017 * Copyright (C) 2013 Hiroshi M, MIT License 00018 * 00019 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00020 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00021 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00022 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00023 * furnished to do so, subject to the following conditions: 00024 * 00025 * The above copyright notice and this permission notice shall be included in all copies or 00026 * substantial portions of the Software. 00027 * 00028 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00029 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00030 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00031 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00032 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00033 * 00034 **/ 00035 00036 #ifndef __MYLCD_H_ 00037 #define __MYLCD_H_ 00038 00039 /* Includes ----------------------------------------------------------------- */ 00040 #include "mbed.h" 00041 /* typedef ------------------------------------------------------------------ */ 00042 00043 /* define ------------------------------------------------------------------- */ 00044 #define ESC 0x1B 00045 #define I2C_ADDR_MYLCD 0x32 00046 #define _i2cSUCCESS 0 00047 #define _i2cFAILURE 1 00048 00049 /* macro -------------------------------------------------------------------- */ 00050 /* variables ---------------------------------------------------------------- */ 00051 /* class -------------------------------------------------------------------- */ 00052 class MyLCD : public Stream 00053 { 00054 private: 00055 static const int i2c_addr = I2C_ADDR_MYLCD << 1; 00056 static const int i2c_bit_wait_us = 20; 00057 static const int i2c_command_wait_ms = 4; 00058 static const int display_columns = 16; 00059 static const int display_rows = 2; 00060 00061 I2C *_i2c; 00062 int _column, _row; 00063 int writeBytes(const char *data, int length, bool repeated=false); 00064 00065 protected: 00066 virtual int _putc(int value); 00067 virtual int _getc(); 00068 00069 void character(int column, int row, int c); 00070 int columns(); 00071 int rows(); 00072 00073 public: 00074 MyLCD(I2C *i2c); 00075 #if DOXYGEN_ONLY 00076 int putc(int value); 00077 int printf(const char* format, ...); 00078 #endif 00079 00080 int gotoCursor( int x, int y ); 00081 int home(void); 00082 int clear(void); 00083 int offDisplay(void); 00084 int onDisplay(void); 00085 int blinkCharacter(void); 00086 int dispCursor(void); 00087 int blinkCursor(void); 00088 int hideCursor(void); 00089 int moveLeftCursor(void); 00090 int moveRightCursor(void); 00091 int moveLeftDisplay(void); 00092 int moveRightDisplay(void); 00093 int printChar(char chr); 00094 int printStr(const char *s); 00095 00096 int saveCustomCharacter(int romCharNum, char lcdCharData[]); 00097 int mapCustomCharacter(int romCharNum, int lcdCharNum); 00098 }; 00099 00100 #endif /* __MYLCD_H_ */
Generated on Mon Jul 25 2022 02:21:29 by
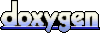