Orignal AVR Tiny USI Based I2C slave LCD Driver.
Dependents: MyLCD_OSARU MyLCD_printf
MyLCD.cpp
00001 /** 00002 ***************************************************************************** 00003 * File Name : MyLCD.cpp 00004 * 00005 * Title : ORGINAL I2C LCD Display Claass Source File 00006 * Revision : 0.1 00007 * Notes : 00008 * Target Board : mbed NXP LPC1768, mbed LPC1114FN28 etc 00009 * Tool Chain : ???? 00010 * 00011 * Revision History: 00012 * When Who Description of change 00013 * ----------- ----------- ----------------------- 00014 * 2013/12/11 Hiroshi M init 00015 ***************************************************************************** 00016 * 00017 * Copyright (C) 2013 Hiroshi M, MIT License 00018 * 00019 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00020 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00021 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00022 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00023 * furnished to do so, subject to the following conditions: 00024 * 00025 * The above copyright notice and this permission notice shall be included in all copies or 00026 * substantial portions of the Software. 00027 * 00028 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00029 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00030 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00031 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00032 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00033 * 00034 **/ 00035 00036 /* Includes ----------------------------------------------------------------- */ 00037 #include "mbed.h" 00038 #include "MyLCD.h" 00039 #include <string.h> 00040 00041 /* Private typedef ---------------------------------------------------------- */ 00042 /* Private define ----------------------------------------------------------- */ 00043 /* Private macro ------------------------------------------------------------ */ 00044 /* Private variables -------------------------------------------------------- */ 00045 00046 /* member fanctions --------------------------------------------------------- */ 00047 00048 00049 // Constractor 00050 MyLCD::MyLCD(I2C *i2c): _i2c(i2c) 00051 { 00052 _column = 0; 00053 _row = 0; 00054 } 00055 00056 int MyLCD::writeBytes(const char *data, int length, bool repeated) 00057 { 00058 wait_us(i2c_bit_wait_us); 00059 _i2c->start(); 00060 00061 wait_us(i2c_bit_wait_us); 00062 if (_i2c->write(i2c_addr) != 1) 00063 { 00064 wait_us(i2c_bit_wait_us); 00065 _i2c->stop(); 00066 return _i2cFAILURE; 00067 } 00068 00069 for (int i = 0; i < length; i++) 00070 { 00071 wait_us(i2c_bit_wait_us); 00072 if (_i2c->write(data[i]) != 1) 00073 { 00074 wait_us(i2c_bit_wait_us); 00075 _i2c->stop(); 00076 return _i2cFAILURE; 00077 } 00078 } 00079 if (!repeated) 00080 { 00081 wait_us(i2c_bit_wait_us); 00082 _i2c->stop(); 00083 } 00084 return _i2cSUCCESS; 00085 } 00086 00087 int MyLCD::gotoCursor( int x, int y ) 00088 { 00089 char buff[4] = { ESC, 'L', x, y }; 00090 00091 _column = x; 00092 _row = y; 00093 00094 return writeBytes(buff, sizeof(buff)); 00095 } 00096 00097 00098 int MyLCD::home(void) 00099 { 00100 char buff[2] = { ESC, 'H' }; 00101 00102 _column = 0; 00103 _row = 0; 00104 00105 return writeBytes(buff, sizeof(buff)); 00106 } 00107 00108 int MyLCD::clear(void) 00109 { 00110 char buff[2] = { ESC, 'C' }; 00111 00112 return writeBytes(buff, sizeof(buff)); 00113 } 00114 00115 int MyLCD::saveCustomCharacter(int romCharNum, char lcdCharData[]) 00116 { 00117 int ret; 00118 00119 char buff[3] = { ESC, 'S', romCharNum }; 00120 00121 ret = writeBytes(buff, sizeof(buff), true); 00122 00123 if (ret == _i2cSUCCESS) 00124 { 00125 ret = writeBytes(lcdCharData, 8, false); 00126 } 00127 wait_ms(100); 00128 00129 return ret; 00130 } 00131 00132 int MyLCD::mapCustomCharacter(int romCharNum, int lcdCharNum) 00133 { 00134 char buff[4] = { ESC, 'M', romCharNum, lcdCharNum }; 00135 00136 return writeBytes(buff, sizeof(buff)); 00137 } 00138 00139 00140 int MyLCD::offDisplay(void) 00141 { 00142 char buff[2] = { ESC, 'X' }; 00143 00144 return writeBytes(buff, sizeof(buff)); 00145 } 00146 00147 int MyLCD::onDisplay(void) 00148 { 00149 char buff[2] = { ESC, 'N' }; 00150 00151 return writeBytes(buff, sizeof(buff)); 00152 } 00153 00154 int MyLCD::blinkCharacter(void) 00155 { 00156 char buff[2] = { ESC, 'B' }; 00157 00158 return writeBytes(buff, sizeof(buff)); 00159 } 00160 00161 int MyLCD::dispCursor(void) 00162 { 00163 char buff[2] = { ESC, 'D' }; 00164 00165 return writeBytes(buff, sizeof(buff)); 00166 } 00167 00168 int MyLCD::blinkCursor(void) 00169 { 00170 char buff[2] = { ESC, 'E' }; 00171 00172 return writeBytes(buff, sizeof(buff)); 00173 } 00174 00175 int MyLCD::hideCursor(void) 00176 { 00177 char buff[2] = { ESC, 'H' }; 00178 00179 return writeBytes(buff, sizeof(buff)); 00180 } 00181 00182 int MyLCD::moveLeftCursor(void) 00183 { 00184 char buff[2] = { ESC, '-' }; 00185 00186 return writeBytes(buff, sizeof(buff)); 00187 } 00188 00189 int MyLCD::moveRightCursor(void) 00190 { 00191 char buff[2] = { ESC, '+' }; 00192 00193 return writeBytes(buff, sizeof(buff)); 00194 } 00195 00196 int MyLCD::moveLeftDisplay(void) 00197 { 00198 char buff[2] = { ESC, '<' }; 00199 00200 return writeBytes(buff, sizeof(buff)); 00201 } 00202 00203 int MyLCD::moveRightDisplay(void) 00204 { 00205 char buff[2] = { ESC, '>' }; 00206 00207 return writeBytes(buff, sizeof(buff)); 00208 } 00209 00210 // 一文字表示 00211 int MyLCD::printChar(char chr) 00212 { 00213 return writeBytes(&chr, 1); 00214 } 00215 00216 int MyLCD::printStr(const char *s) 00217 { 00218 return writeBytes(s, strlen(s)); 00219 } 00220 00221 00222 int MyLCD::_putc(int value) 00223 { 00224 if (value == '\n') 00225 { 00226 _column = 0; 00227 _row++; 00228 if (_row >= rows()) 00229 { 00230 _row = 0; 00231 } 00232 } 00233 else 00234 { 00235 character(_column, _row, value); 00236 _column++; 00237 if (_column >= columns()) 00238 { 00239 _column = 0; 00240 _row++; 00241 if (_row >= rows()) 00242 { 00243 _row = 0; 00244 } 00245 } 00246 } 00247 return value; 00248 } 00249 00250 int MyLCD::_getc() 00251 { 00252 return -1; 00253 } 00254 00255 void MyLCD::character(int column, int row, int c) 00256 { 00257 gotoCursor(_column, row ); 00258 printChar(c); 00259 } 00260 00261 int MyLCD::columns() 00262 { 00263 return display_columns; 00264 } 00265 00266 int MyLCD::rows() 00267 { 00268 return display_rows; 00269 }
Generated on Mon Jul 25 2022 02:21:29 by
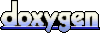