Orignal AVR Tiny USI Based I2C slave Charlieplexing 7SEG LED Driver.
Embed:
(wiki syntax)
Show/hide line numbers
My7SEG.cpp
00001 /** 00002 ***************************************************************************** 00003 * File Name : My7SEG.cpp 00004 * 00005 * Title : ORGINAL I2C LCD Display Claass Source File 00006 * Revision : 0.1 00007 * Notes : 00008 * Target Board : mbed NXP LPC1768, mbed LPC1114FN28 etc 00009 * Tool Chain : ???? 00010 * 00011 * Revision History: 00012 * When Who Description of change 00013 * ----------- ----------- ----------------------- 00014 * 2013/12/11 Hiroshi M init 00015 ***************************************************************************** 00016 * 00017 * Copyright (C) 2013 Hiroshi M, MIT License 00018 * 00019 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00020 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00021 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00022 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00023 * furnished to do so, subject to the following conditions: 00024 * 00025 * The above copyright notice and this permission notice shall be included in all copies or 00026 * substantial portions of the Software. 00027 * 00028 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00029 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00030 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00031 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00032 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00033 * 00034 **/ 00035 00036 /* Includes ----------------------------------------------------------------- */ 00037 #include "mbed.h" 00038 #include "My7SEG.h" 00039 00040 /* Private typedef ---------------------------------------------------------- */ 00041 /* Private define ----------------------------------------------------------- */ 00042 /* Private macro ------------------------------------------------------------ */ 00043 /* Private variables -------------------------------------------------------- */ 00044 /* member fanctions --------------------------------------------------------- */ 00045 00046 // Constractor 00047 My7SEG::My7SEG(I2C *i2c): _i2c(i2c) 00048 { 00049 } 00050 00051 int My7SEG::writeBytes(const char *data, int length, bool repeated) 00052 { 00053 wait_us(i2c_bit_wait_us); 00054 _i2c->start(); 00055 00056 wait_us(i2c_bit_wait_us); 00057 if (_i2c->write(i2c_addr) != 1) 00058 { 00059 wait_us(i2c_bit_wait_us); 00060 _i2c->stop(); 00061 return _i2cFAILURE; 00062 } 00063 00064 for (int i = 0; i < length; i++) 00065 { 00066 wait_us(i2c_bit_wait_us); 00067 if (_i2c->write(data[i]) != 1) 00068 { 00069 wait_us(i2c_bit_wait_us); 00070 _i2c->stop(); 00071 return _i2cFAILURE; 00072 } 00073 } 00074 if (!repeated) 00075 { 00076 wait_us(i2c_bit_wait_us); 00077 _i2c->stop(); 00078 } 00079 return _i2cSUCCESS; 00080 } 00081 00082 int My7SEG::clear(void) 00083 { 00084 char buff[2] = { ESC, 'C' }; 00085 00086 return writeBytes(buff, sizeof(buff)); 00087 } 00088 00089 int My7SEG::shift_left(void) 00090 { 00091 char buff[2] = { ESC, '<' }; 00092 00093 return writeBytes(buff, sizeof(buff)); 00094 } 00095 00096 int My7SEG::shift_right(void) 00097 { 00098 char buff[2] = { ESC, '>' }; 00099 00100 return writeBytes(buff, sizeof(buff)); 00101 } 00102 00103 int My7SEG::rotato_left(void) 00104 { 00105 char buff[2] = { ESC, '[' }; 00106 00107 return writeBytes(buff, sizeof(buff)); 00108 } 00109 00110 int My7SEG::rotato_right(void) 00111 { 00112 char buff[2] = { ESC, ']' }; 00113 00114 return writeBytes(buff, sizeof(buff)); 00115 } 00116 00117 // 一文字表示 00118 int My7SEG::printChar(char c) 00119 { 00120 return writeBytes(&c, 1); 00121 } 00122 00123 int My7SEG::printPosChar(int pos, char chr) 00124 { 00125 char buff[4]; 00126 00127 if( pos < 0 && 7 < pos ) 00128 { 00129 return _i2cFAILURE; 00130 } 00131 00132 buff[0] = ESC; 00133 buff[1] = 'L'; 00134 buff[2] = '0' + pos; 00135 buff[3] = chr; 00136 00137 return writeBytes(buff, sizeof(buff)); 00138 } 00139 00140 int My7SEG::printStr(const char *s) 00141 { 00142 int ret; 00143 char c; 00144 00145 ret = _i2cSUCCESS; 00146 while ((c = *s++)!=NULL) 00147 { 00148 ret = writeBytes(&c, 1); 00149 if (ret == _i2cFAILURE) 00150 { 00151 return ret; 00152 } 00153 } 00154 00155 return ret; 00156 } 00157 00158 ///////////////////////////////////////////////
Generated on Tue Aug 16 2022 18:57:02 by
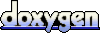