
uIP 1.0 based webserver for LPC1114 + ENC28J60
Embed:
(wiki syntax)
Show/hide line numbers
uip-fw.h
Go to the documentation of this file.
00001 /** 00002 * \addtogroup uipfw 00003 * @{ 00004 */ 00005 00006 /** 00007 * \file 00008 * uIP packet forwarding header file. 00009 * \author Adam Dunkels <adam@sics.se> 00010 */ 00011 00012 /* 00013 * Copyright (c) 2004, Swedish Institute of Computer Science. 00014 * All rights reserved. 00015 * 00016 * Redistribution and use in source and binary forms, with or without 00017 * modification, are permitted provided that the following conditions 00018 * are met: 00019 * 1. Redistributions of source code must retain the above copyright 00020 * notice, this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright 00022 * notice, this list of conditions and the following disclaimer in the 00023 * documentation and/or other materials provided with the distribution. 00024 * 3. Neither the name of the Institute nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE INSTITUTE AND CONTRIBUTORS ``AS IS'' AND 00029 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00031 * ARE DISCLAIMED. IN NO EVENT SHALL THE INSTITUTE OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS 00034 * OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) 00035 * HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY 00037 * OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00038 * SUCH DAMAGE. 00039 * 00040 * This file is part of the Contiki operating system. 00041 * 00042 * Author: Adam Dunkels <adam@sics.se> 00043 * 00044 */ 00045 #ifndef __UIP_FW_H__ 00046 #define __UIP_FW_H__ 00047 00048 #include "uip.h" 00049 00050 /** 00051 * Representation of a uIP network interface. 00052 */ 00053 struct uip_fw_netif { 00054 struct uip_fw_netif *next; /**< Pointer to the next interface when 00055 linked in a list. */ 00056 uip_ipaddr_t ipaddr; /**< The IP address of this interface. */ 00057 uip_ipaddr_t netmask; /**< The netmask of the interface. */ 00058 uint8_t (* output)(void); 00059 /**< A pointer to the function that 00060 sends a packet. */ 00061 }; 00062 00063 /** 00064 * Instantiating macro for a uIP network interface. 00065 * 00066 * Example: 00067 \code 00068 struct uip_fw_netif slipnetif = 00069 {UIP_FW_NETIF(192,168,76,1, 255,255,255,0, slip_output)}; 00070 \endcode 00071 * \param ip1,ip2,ip3,ip4 The IP address of the network interface. 00072 * 00073 * \param nm1,nm2,nm3,nm4 The netmask of the network interface. 00074 * 00075 * \param outputfunc A pointer to the output function of the network interface. 00076 * 00077 * \hideinitializer 00078 */ 00079 #define UIP_FW_NETIF(ip1,ip2,ip3,ip4, nm1,nm2,nm3,nm4, outputfunc) \ 00080 NULL, \ 00081 { {ip1, ip2, ip3, ip4} }, \ 00082 { {nm1, nm2, nm3, nm4} }, \ 00083 outputfunc 00084 00085 /** 00086 * Set the IP address of a network interface. 00087 * 00088 * \param netif A pointer to the uip_fw_netif structure for the network interface. 00089 * 00090 * \param addr A pointer to an IP address. 00091 * 00092 * \hideinitializer 00093 */ 00094 #define uip_fw_setipaddr(netif, addr) \ 00095 do { (netif)->ipaddr[0] = ((uint16_t *)(addr))[0]; \ 00096 (netif)->ipaddr[1] = ((uint16_t *)(addr))[1]; } while(0) 00097 /** 00098 * Set the netmask of a network interface. 00099 * 00100 * \param netif A pointer to the uip_fw_netif structure for the network interface. 00101 * 00102 * \param addr A pointer to an IP address representing the netmask. 00103 * 00104 * \hideinitializer 00105 */ 00106 #define uip_fw_setnetmask(netif, addr) \ 00107 do { (netif)->netmask[0] = ((uint16_t *)(addr))[0]; \ 00108 (netif)->netmask[1] = ((uint16_t *)(addr))[1]; } while(0) 00109 00110 void uip_fw_init(void); 00111 uint8_t uip_fw_forward(void); 00112 uint8_t uip_fw_output(void); 00113 void uip_fw_register(struct uip_fw_netif *netif); 00114 void uip_fw_default(struct uip_fw_netif *netif); 00115 void uip_fw_periodic(void); 00116 00117 00118 /** 00119 * A non-error message that indicates that a packet should be 00120 * processed locally. 00121 * 00122 * \hideinitializer 00123 */ 00124 #define UIP_FW_LOCAL 0 00125 00126 /** 00127 * A non-error message that indicates that something went OK. 00128 * 00129 * \hideinitializer 00130 */ 00131 #define UIP_FW_OK 0 00132 00133 /** 00134 * A non-error message that indicates that a packet was forwarded. 00135 * 00136 * \hideinitializer 00137 */ 00138 #define UIP_FW_FORWARDED 1 00139 00140 /** 00141 * A non-error message that indicates that a zero-length packet 00142 * transmission was attempted, and that no packet was sent. 00143 * 00144 * \hideinitializer 00145 */ 00146 #define UIP_FW_ZEROLEN 2 00147 00148 /** 00149 * An error message that indicates that a packet that was too large 00150 * for the outbound network interface was detected. 00151 * 00152 * \hideinitializer 00153 */ 00154 #define UIP_FW_TOOLARGE 3 00155 00156 /** 00157 * An error message that indicates that no suitable interface could be 00158 * found for an outbound packet. 00159 * 00160 * \hideinitializer 00161 */ 00162 #define UIP_FW_NOROUTE 4 00163 00164 /** 00165 * An error message that indicates that a packet that should be 00166 * forwarded or output was dropped. 00167 * 00168 * \hideinitializer 00169 */ 00170 #define UIP_FW_DROPPED 5 00171 00172 00173 #endif /* __UIP_FW_H__ */ 00174 00175 /** @} */
Generated on Tue Jul 12 2022 12:52:12 by
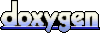