
uIP 1.0 based webserver for LPC1114 + ENC28J60
Embed:
(wiki syntax)
Show/hide line numbers
httpd-fs.cpp
00001 /* 00002 * Copyright (c) 2001, Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions 00007 * are met: 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 3. Neither the name of the Institute nor the names of its contributors 00014 * may be used to endorse or promote products derived from this software 00015 * without specific prior written permission. 00016 * 00017 * THIS SOFTWARE IS PROVIDED BY THE INSTITUTE AND CONTRIBUTORS ``AS IS'' AND 00018 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00019 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00020 * ARE DISCLAIMED. IN NO EVENT SHALL THE INSTITUTE OR CONTRIBUTORS BE LIABLE 00021 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00022 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS 00023 * OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) 00024 * HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00025 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY 00026 * OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00027 * SUCH DAMAGE. 00028 * 00029 * This file is part of the lwIP TCP/IP stack. 00030 * 00031 * Author: Adam Dunkels <adam@sics.se> 00032 * 00033 * $Id: httpd-fs.c,v 1.1 2006/06/07 09:13:08 adam Exp $ 00034 */ 00035 00036 extern "C" { 00037 #include "httpd.h" 00038 } 00039 //#include "httpd-fs.h" 00040 #include "httpd-fs-struct.h" 00041 00042 #ifndef NULL 00043 #define NULL 0 00044 #endif /* NULL */ 00045 00046 #include "httpd-fs-data.h" 00047 00048 #if HTTPD_FS_STATISTICS 00049 static u16_t count[HTTPD_FS_NUMFILES]; 00050 #endif /* HTTPD_FS_STATISTICS */ 00051 00052 /*-----------------------------------------------------------------------------------*/ 00053 static u8_t 00054 httpd_fs_strcmp(const char *str1, const char *str2) 00055 { 00056 u8_t i; 00057 i = 0; 00058 loop: 00059 00060 if(str2[i] == 0 || 00061 str1[i] == '\r' || 00062 str1[i] == '\n') { 00063 return 0; 00064 } 00065 00066 if(str1[i] != str2[i]) { 00067 return 1; 00068 } 00069 00070 00071 ++i; 00072 goto loop; 00073 } 00074 /*-----------------------------------------------------------------------------------*/ 00075 int 00076 httpd_fs_open(const char *name, struct httpd_fs_file *file) 00077 { 00078 #if HTTPD_FS_STATISTICS 00079 u16_t i = 0; 00080 #endif /* HTTPD_FS_STATISTICS */ 00081 struct httpd_fsdata_file_noconst *f; 00082 00083 for(f = (struct httpd_fsdata_file_noconst *)HTTPD_FS_ROOT; 00084 f != NULL; 00085 f = (struct httpd_fsdata_file_noconst *)f->next) { 00086 00087 if(httpd_fs_strcmp(name, f->name) == 0) { 00088 file->data = f->data; 00089 file->len = f->len; 00090 #if HTTPD_FS_STATISTICS 00091 ++count[i]; 00092 #endif /* HTTPD_FS_STATISTICS */ 00093 return 1; 00094 } 00095 #if HTTPD_FS_STATISTICS 00096 ++i; 00097 #endif /* HTTPD_FS_STATISTICS */ 00098 00099 } 00100 return 0; 00101 } 00102 /*-----------------------------------------------------------------------------------*/ 00103 void 00104 httpd_fs_init(void) 00105 { 00106 #if HTTPD_FS_STATISTICS 00107 u16_t i; 00108 for(i = 0; i < HTTPD_FS_NUMFILES; i++) { 00109 count[i] = 0; 00110 } 00111 #endif /* HTTPD_FS_STATISTICS */ 00112 } 00113 /*-----------------------------------------------------------------------------------*/ 00114 #if HTTPD_FS_STATISTICS 00115 u16_t httpd_fs_count 00116 (char *name) 00117 { 00118 struct httpd_fsdata_file_noconst *f; 00119 u16_t i; 00120 00121 i = 0; 00122 for(f = (struct httpd_fsdata_file_noconst *)HTTPD_FS_ROOT; 00123 f != NULL; 00124 f = (struct httpd_fsdata_file_noconst *)f->next) { 00125 00126 if(httpd_fs_strcmp(name, f->name) == 0) { 00127 return count[i]; 00128 } 00129 ++i; 00130 } 00131 return 0; 00132 } 00133 #endif /* HTTPD_FS_STATISTICS */ 00134 /*-----------------------------------------------------------------------------------*/
Generated on Tue Jul 12 2022 12:52:12 by
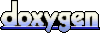