for EthernetInterface library compatibility.\\ ** Unoffical fix. may be a problem. **
Dependents: SNIC-httpclient-example SNIC-ntpclient-example
Fork of SNICInterface by
TCPSocketConnection.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 /* Copyright (C) 2014 Murata Manufacturing Co.,Ltd., MIT License 00019 * port to the muRata, SWITCH SCIENCE Wi-FI module TypeYD SNIC-UART. 00020 */ 00021 #ifndef TCPSOCKET_H 00022 #define TCPSOCKET_H 00023 00024 #include "Socket.h" 00025 #include "Endpoint.h" 00026 00027 /** 00028 Interface class for TCP socket of using SNIC UART. 00029 */ 00030 class TCPSocketConnection : public Socket, public Endpoint { 00031 00032 public: 00033 /** TCP socket connection 00034 */ 00035 TCPSocketConnection(); 00036 virtual ~TCPSocketConnection(); 00037 00038 /** Connects this TCP socket to the server 00039 @param host The strings of ip address. 00040 @param port The host's port to connect to. 00041 @return 0 on success, -1 on failure. 00042 @note This function is blocked until a returns. 00043 When you use it by UI thread, be careful. 00044 */ 00045 int connect( const char *ip_addr_p, unsigned short port ); 00046 00047 /** Check if the socket is connected 00048 @return true if connected, false otherwise. 00049 */ 00050 bool is_connected(void); 00051 00052 /** Send data to the remote host. 00053 @param data The buffer to send to the host. 00054 @param length The length of the buffer to send. 00055 @return the number of written bytes on success (>=0) or -1 on failure 00056 */ 00057 int send(char *data_p, int length); 00058 00059 /** Send data to the remote host. 00060 @param data The buffer to send to the host. 00061 @param length The length of the buffer to send. 00062 @return the number of written bytes on success (>=0) or -1 on failure 00063 */ 00064 int send_all(char *data_p, int length); 00065 00066 /** Receive data from the remote host. 00067 @param data The buffer in which to store the data received from the host. 00068 @param length The maximum length of the buffer. 00069 @return the number of received bytes on success (>=0) or -1 on failure 00070 */ 00071 int receive(char *data_p, int length); 00072 00073 /** Send data to the remote host. 00074 @param data The buffer to send to the host. 00075 @param length The length of the buffer to send. 00076 @return the number of written bytes on success (>=0) or -1 on failure 00077 */ 00078 int receive_all(char *data_p, int length); 00079 00080 void setAcceptSocket( int socket_id ); 00081 private: 00082 00083 }; 00084 #endif
Generated on Tue Jul 12 2022 18:43:50 by
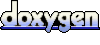