for EthernetInterface library compatibility.\\ ** Unoffical fix. may be a problem. **
Dependents: SNIC-httpclient-example SNIC-ntpclient-example
Fork of SNICInterface by
SNIC_UartCommandManager.h
00001 /* Copyright (C) 2014 Murata Manufacturing Co.,Ltd., MIT License 00002 * muRata, SWITCH SCIENCE Wi-FI module TypeYD SNIC-UART. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 #ifndef _SNIC_UART_COMMAND_MANAGER_H_ 00020 #define _SNIC_UART_COMMAND_MANAGER_H_ 00021 #include "MurataObject.h" 00022 #include "mbed.h" 00023 #include "rtos.h" 00024 00025 /** Max length of SSID */ 00026 #define SSID_MAX_LENGTH 32 00027 /** Max length of BSSID */ 00028 #define BSSID_MAC_LENTH 6 00029 /** Length of Country code */ 00030 #define COUNTRYC_CODE_LENTH 2 00031 00032 /** Wait signal ID of UART command */ 00033 #define UART_COMMAND_SIGNAL 0x00000001 00034 /** Timeout of UART command wait(ms)*/ 00035 #define UART_COMMAND_WAIT_TIMEOUT 10000 00036 00037 /** Scan result structure used by scanresults handler 00038 */ 00039 typedef struct { 00040 bool is_complete; 00041 /** Channel */ 00042 unsigned char channel; 00043 /** RSSI */ 00044 signed char rssi; 00045 /** Security type */ 00046 unsigned char security; 00047 /** BSSID */ 00048 unsigned char bssid[BSSID_MAC_LENTH]; 00049 /** Network type */ 00050 unsigned char network_type; 00051 /** Max data rate */ 00052 unsigned char max_rate; 00053 /** SSID */ 00054 char ssid[SSID_MAX_LENGTH+1]; 00055 }tagSCAN_RESULT_T; 00056 00057 /** Internal class for managing the SNIC UART command. 00058 */ 00059 class C_SNIC_UartCommandManager: public C_MurataObject 00060 { 00061 friend class C_SNIC_Core; 00062 friend class C_SNIC_WifiInterface; 00063 friend class TCPSocketConnection; 00064 friend class TCPSocketServer; 00065 friend class UDPSocket; 00066 friend class Socket; 00067 00068 private: 00069 virtual ~C_SNIC_UartCommandManager(); 00070 00071 /** Set Command ID 00072 @param cmd_id Command ID 00073 */ 00074 void setCommandID( unsigned char cmd_id ); 00075 00076 /** Get Command ID 00077 @return Command ID 00078 */ 00079 unsigned char getCommandID(); 00080 00081 /** Set Command SubID 00082 @param cmd_sid Command Sub ID 00083 */ 00084 void setCommandSID( unsigned char cmd_sid ); 00085 00086 /** Get Command SubID 00087 @return Command Sub ID 00088 */ 00089 unsigned char getCommandSID(); 00090 00091 /** Set Command status 00092 @param status Command status 00093 */ 00094 void setCommandStatus( unsigned char status ); 00095 00096 /** Get Command status 00097 @return Command status 00098 */ 00099 unsigned char getCommandStatus(); 00100 00101 /** Set Response buffer 00102 @param buf_p Pointer of response buffer 00103 */ 00104 void setResponseBuf( unsigned char *buf_p ); 00105 00106 /** Get Response buffer 00107 @return Pointer of response buffer 00108 */ 00109 unsigned char *getResponseBuf(); 00110 00111 /** Set scan result callback hander 00112 @param handler_p Pointer of callback function 00113 */ 00114 void setScanResultHandler( void (*handler_p)(tagSCAN_RESULT_T *scan_result) ); 00115 00116 void bufferredPacket( unsigned char *payload_p, int payload_len ); 00117 00118 void bufferredUDPPacket( unsigned char *payload_p, int payload_len ); 00119 00120 void scanResultIndicate( unsigned char *payload_p, int payload_len ); 00121 00122 void connectedTCPClient( unsigned char *payload_p, int payload_len ); 00123 00124 /** Checks in the command which is waiting from Command ID and Sub ID. 00125 @param command_id Command ID 00126 @param payload_p Command payload 00127 @return true: Waiting command / false: Not waiting command 00128 */ 00129 bool isWaitingCommand( unsigned int command_id, unsigned char *payload_p ); 00130 00131 int wait(); 00132 00133 int signal(); 00134 00135 private: 00136 /** Command request thread ID */ 00137 osThreadId mCommandThreadID; 00138 /** Command ID */ 00139 unsigned char mCommandID; 00140 /** Command SubID */ 00141 unsigned char mCommandSID; 00142 /** Status of command response */ 00143 unsigned char mCommandStatus; 00144 /** ResponseData of command response */ 00145 unsigned char *mResponseBuf_p; 00146 /** Scan result handler */ 00147 void (*mScanResultHandler_p)(tagSCAN_RESULT_T *scan_result); 00148 }; 00149 00150 #endif
Generated on Tue Jul 12 2022 18:43:50 by
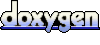