for EthernetInterface library compatibility.\\ ** Unoffical fix. may be a problem. **
Dependents: SNIC-httpclient-example SNIC-ntpclient-example
Fork of SNICInterface by
SNIC_UartCommandManager.cpp
00001 /* Copyright (C) 2014 Murata Manufacturing Co.,Ltd., MIT License 00002 * muRata, SWITCH SCIENCE Wi-FI module TypeYD SNIC-UART. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 #include "SNIC_UartCommandManager.h" 00020 #include "SNIC_Core.h" 00021 00022 C_SNIC_UartCommandManager::~C_SNIC_UartCommandManager() 00023 { 00024 } 00025 00026 void C_SNIC_UartCommandManager::setCommandID( unsigned char cmd_id ) 00027 { 00028 mCommandID = cmd_id; 00029 } 00030 00031 unsigned char C_SNIC_UartCommandManager::getCommandID() 00032 { 00033 return mCommandID; 00034 } 00035 00036 void C_SNIC_UartCommandManager::setCommandSID( unsigned char cmd_sid ) 00037 { 00038 mCommandSID = cmd_sid; 00039 } 00040 00041 unsigned char C_SNIC_UartCommandManager::getCommandSID() 00042 { 00043 return mCommandSID; 00044 } 00045 00046 void C_SNIC_UartCommandManager::setCommandStatus( unsigned char status ) 00047 { 00048 mCommandStatus = status; 00049 } 00050 00051 unsigned char C_SNIC_UartCommandManager::getCommandStatus() 00052 { 00053 return mCommandStatus; 00054 } 00055 00056 void C_SNIC_UartCommandManager::setResponseBuf( unsigned char *buf_p ) 00057 { 00058 mResponseBuf_p = buf_p; 00059 } 00060 00061 unsigned char *C_SNIC_UartCommandManager::getResponseBuf() 00062 { 00063 return mResponseBuf_p; 00064 } 00065 00066 void C_SNIC_UartCommandManager::setScanResultHandler( void (*handler_p)(tagSCAN_RESULT_T *scan_result) ) 00067 { 00068 mScanResultHandler_p = handler_p; 00069 } 00070 00071 00072 int C_SNIC_UartCommandManager::wait() 00073 { 00074 int ret = 0; 00075 00076 // Get thread ID 00077 mCommandThreadID = osThreadGetId(); 00078 00079 // Signal flags that are reported as event are automatically cleared. 00080 osEvent event_ret = osSignalWait( UART_COMMAND_SIGNAL, UART_COMMAND_WAIT_TIMEOUT); 00081 if( event_ret.status != osEventSignal ) 00082 { 00083 ret = -1; 00084 } 00085 00086 return ret; 00087 } 00088 00089 int C_SNIC_UartCommandManager::signal() 00090 { 00091 // set signal 00092 return osSignalSet(mCommandThreadID, UART_COMMAND_SIGNAL); 00093 } 00094 00095 bool C_SNIC_UartCommandManager::isWaitingCommand( unsigned int command_id, unsigned char *payload_p ) 00096 { 00097 bool ret = false; 00098 00099 if( (command_id == getCommandID()) 00100 && (payload_p[0] == getCommandSID()) ) 00101 { 00102 ret = true; 00103 } 00104 return ret; 00105 } 00106 00107 void C_SNIC_UartCommandManager::scanResultIndicate( unsigned char *payload_p, int payload_len ) 00108 { 00109 if( (payload_p == NULL) || (mScanResultHandler_p == NULL) ) 00110 { 00111 return; 00112 } 00113 00114 tagSCAN_RESULT_T scan_result; 00115 int ap_count = payload_p[2]; 00116 00117 if( ap_count == 0 ) 00118 { 00119 mScanResultHandler_p( NULL ); 00120 } 00121 00122 unsigned char *ap_info_p = &payload_p[3]; 00123 int ap_info_idx = 0; 00124 00125 for( int i = 0; i < ap_count; i++ ) 00126 { 00127 scan_result.channel = ap_info_p[ap_info_idx]; 00128 ap_info_idx++; 00129 scan_result.rssi = (signed)ap_info_p[ap_info_idx]; 00130 ap_info_idx++; 00131 scan_result.security= ap_info_p[ap_info_idx]; 00132 ap_info_idx++; 00133 memcpy( scan_result.bssid, &ap_info_p[ap_info_idx], BSSID_MAC_LENTH ); 00134 ap_info_idx += BSSID_MAC_LENTH; 00135 scan_result.network_type= ap_info_p[ap_info_idx]; 00136 ap_info_idx++; 00137 scan_result.max_rate= ap_info_p[ap_info_idx]; 00138 ap_info_idx++; 00139 ap_info_idx++; // reserved 00140 strcpy( scan_result.ssid, (char *)&ap_info_p[ap_info_idx] ); 00141 ap_info_idx += strlen( (char *)&ap_info_p[ap_info_idx] ); 00142 ap_info_idx++; 00143 00144 // Scanresult callback 00145 mScanResultHandler_p( &scan_result ); 00146 } 00147 } 00148 00149 void C_SNIC_UartCommandManager::bufferredPacket( unsigned char *payload_p, int payload_len ) 00150 { 00151 if( (payload_p == NULL) || (payload_len == 0) ) 00152 { 00153 return; 00154 } 00155 00156 C_SNIC_Core *instance_p = C_SNIC_Core::getInstance(); 00157 00158 int socket_id; 00159 unsigned short recv_len; 00160 00161 // Get socket id from payload 00162 socket_id = payload_p[2]; 00163 00164 // DEBUG_PRINT("bufferredPacket socket id:%d\r\n", socket_id); 00165 // Get Connection information 00166 C_SNIC_Core::tagCONNECT_INFO_T *con_info_p = instance_p->getConnectInfo( socket_id ); 00167 if( con_info_p == NULL ) 00168 { 00169 return; 00170 } 00171 00172 if( con_info_p->is_connected == false ) 00173 { 00174 DEBUG_PRINT(" Socket id \"%d\" is not connected\r\n", socket_id); 00175 return; 00176 } 00177 00178 // Get receive length from payload 00179 recv_len= ((payload_p[3]<<8) & 0xFF00) | payload_p[4]; 00180 00181 while( con_info_p->is_receive_complete == false ) 00182 { 00183 Thread::yield(); 00184 } 00185 00186 // DEBUG_PRINT("bufferredPacket recv_len:%d\r\n", recv_len); 00187 int i; 00188 for(i = 0; i < recv_len; i++ ) 00189 { 00190 if( con_info_p->recvbuf_p->isFull() ) 00191 { 00192 DEBUG_PRINT("Receive buffer is full.\r\n"); 00193 break; 00194 } 00195 // Add to receive buffer 00196 con_info_p->recvbuf_p->queue( payload_p[5+i] ); 00197 } 00198 //DEBUG_PRINT("###Receive queue[%d]\r\n", i); 00199 con_info_p->mutex.lock(); 00200 con_info_p->is_receive_complete = false; 00201 con_info_p->is_received = true; 00202 con_info_p->mutex.unlock(); 00203 // Thread::yield(); 00204 } 00205 00206 void C_SNIC_UartCommandManager::connectedTCPClient( unsigned char *payload_p, int payload_len ) 00207 { 00208 if( (payload_p == NULL) || (payload_len == 0) ) 00209 { 00210 return; 00211 } 00212 00213 C_SNIC_Core *instance_p = C_SNIC_Core::getInstance(); 00214 int socket_id; 00215 00216 // Get socket id of client from payload 00217 socket_id = payload_p[3]; 00218 00219 DEBUG_PRINT("[connectedTCPClient] socket id:%d\r\n", socket_id); 00220 00221 // Get Connection information 00222 C_SNIC_Core::tagCONNECT_INFO_T *con_info_p = instance_p->getConnectInfo( socket_id ); 00223 if( con_info_p == NULL ) 00224 { 00225 return; 00226 } 00227 00228 if( con_info_p->recvbuf_p == NULL ) 00229 { 00230 // DEBUG_PRINT( "create recv buffer[socket:%d]\r\n", socket_id); 00231 con_info_p->recvbuf_p = new CircBuffer<char>(SNIC_UART_RECVBUF_SIZE); 00232 } 00233 con_info_p->is_connected = true; 00234 con_info_p->is_received = false; 00235 con_info_p->is_accept = true; 00236 con_info_p->parent_socket = payload_p[2]; 00237 } 00238 00239 void C_SNIC_UartCommandManager::bufferredUDPPacket( unsigned char *payload_p, int payload_len ) 00240 { 00241 if( (payload_p == NULL) || (payload_len == 0) ) 00242 { 00243 return; 00244 } 00245 00246 C_SNIC_Core *instance_p = C_SNIC_Core::getInstance(); 00247 00248 // Get Connection information 00249 C_SNIC_Core::tagUDP_RECVINFO_T *con_info_p = instance_p->getUdpRecvInfo( payload_p[2] ); 00250 if( con_info_p == NULL ) 00251 { 00252 return; 00253 } 00254 00255 if( con_info_p->recvbuf_p == NULL ) 00256 { 00257 // DEBUG_PRINT( "create recv buffer[socket:%d]\r\n", payload_p[2]); 00258 con_info_p->recvbuf_p = new CircBuffer<char>(SNIC_UART_RECVBUF_SIZE); 00259 } 00260 con_info_p->mutex.lock(); 00261 con_info_p->is_received = true; 00262 con_info_p->mutex.unlock(); 00263 00264 // Set remote IP address and remote port 00265 con_info_p->from_ip = ((payload_p[3] << 24) | (payload_p[4] << 16) | (payload_p[5] << 8) | payload_p[6]); 00266 con_info_p->from_port = ((payload_p[7] << 8) | payload_p[8]); 00267 00268 unsigned short recv_len; 00269 // Get receive length from payload 00270 recv_len= ((payload_p[9]<<8) & 0xFF00) | payload_p[10]; 00271 for( int i = 0; i < recv_len; i++ ) 00272 { 00273 if( con_info_p->recvbuf_p->isFull() ) 00274 { 00275 DEBUG_PRINT("Receive buffer is full.\r\n"); 00276 break; 00277 } 00278 00279 // Add to receive buffer 00280 con_info_p->recvbuf_p->queue( payload_p[11+i] ); 00281 } 00282 }
Generated on Tue Jul 12 2022 18:43:50 by
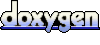