This Library for DOGS-102 Graphic LCD module. Based on Igor Skochinsky's "DOGLCDDemo" program.
Dependents: DOGS102_Example1 DOGS102_Example2
Fork of DOGLCDDemo by
TrimeshObject.h
00001 /* 00002 * libmbed-graphics 2D and wireframe 3D graphics library for the MBED 00003 * microcontroller platform 00004 * Copyright (C) <2009> Michael Sheldon <mike@mikeasoft.com> 00005 * 00006 * This library is free software; you can redistribute it and/or 00007 * modify it under the terms of the GNU Library General Public 00008 * License as published by the Free Software Foundation; either 00009 * version 2 of the License, or (at your option) any later version. 00010 * 00011 * This library is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00014 * Library General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Library General Public 00017 * License along with this library; if not, write to the 00018 * Free Software Foundation, Inc., 59 Temple Place - Suite 330, 00019 * Boston, MA 02111-1307, USA. 00020 */ 00021 00022 #ifndef MBED_TRIMESHOBJECT_H 00023 #define MBED_TRIMESHOBJECT_H 00024 00025 #include "Object3D.h" 00026 00027 /* Class: TrimeshObject 00028 * Constructs 3D objects from triangle meshes specified by lists of 00029 * vertices and faces. 00030 */ 00031 class TrimeshObject : public Object3D { 00032 00033 public: 00034 /* Constructor: TrimeshObject 00035 * Instantiate a trimesh object. 00036 * 00037 * Parameters: 00038 * vertices - A multidimensional array containing a list of vertices. 00039 * faces - A multidimensional array containing a list of faces, connecting vertices together to form triangles. 00040 * num_faces - The number of faces in the "faces" array. 00041 */ 00042 TrimeshObject(int vertices[][3], int faces[][3], int num_faces); 00043 00044 /* Function: render 00045 * Draws the trimesh object to the specified graphical context. 00046 * 00047 * Parameters: 00048 * g - The graphical context to which the trimesh object should be rendered. 00049 */ 00050 virtual void render(Graphics &g); 00051 00052 protected: 00053 int (*_vertices)[3]; 00054 int (*_faces)[3]; 00055 int _num_faces; 00056 00057 }; 00058 00059 #endif
Generated on Tue Jul 12 2022 17:26:39 by
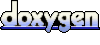