
part of the preparation works for Ina-city Hackerthon
Fork of Wio_3G_example by
main.cpp
00001 #include "mbed.h" 00002 #include <sstream> 00003 #include "easy-connect.h" 00004 #include "https_request.h" 00005 #include "ssl_ca_pem.h" 00006 00007 // For your API Token, refer to "API token" in your application setting page 00008 const char API_TOKEN[] = "api-token"; 00009 // Your domain name can be seen in the usage explanation with curl. 00010 const char URL[] = "https://{domain}.cybozu.com/k/v1/record.json"; 00011 00012 // app_id, application id, can be checked with your application's URL 00013 // e.g. https://{domain}.cybozu.com/k/2/ -> app_id is 2 00014 int app_id = 1; 00015 00016 #if defined(__CC_ARM) 00017 // To avoid "invalid multibyte character sequence" warning 00018 #pragma diag_suppress 870 00019 #endif 00020 00021 #if !defined(TARGET_WIO_3G) 00022 #error Selected target is not supported. 00023 #endif 00024 00025 // on-board resources 00026 Serial pc(USBTX, USBRX, 115200); 00027 DigitalOut GrovePower(PB_10, 1); 00028 00029 #define D20 (PB_4) 00030 00031 // Grove sensors 00032 00033 // buzzer 00034 DigitalOut buzzer(D38); 00035 00036 // button or touch sensor 00037 InterruptIn btn(D20); 00038 int id = 0; 00039 00040 void push() 00041 { 00042 id++; 00043 } 00044 00045 // JSON simplicity parser 00046 char* j_paser( const char *buf , char *word , char *out ) 00047 { 00048 int i = 0; 00049 char *p; 00050 char _word[64] = "\"\0"; 00051 00052 strcat(_word , word ); 00053 strcat(_word , "\"" ); 00054 00055 p = strstr( (char*)buf , _word ) + 2 + strlen(_word); 00056 00057 while( (p[i] != ',')&&(p[i] != '\n')&&(p[i] != '"') ) { 00058 out[i] = p[i]; 00059 i++; 00060 } 00061 out[i] = '\0'; 00062 00063 return p; 00064 } 00065 00066 // main method. retrieve data from kintone by HTTP GET 00067 int main() 00068 { 00069 btn.mode(PullUp); 00070 btn.fall(push); // set interrupt handler 00071 00072 NetworkInterface* network = NULL; 00073 network = easy_connect(true); // If true, prints out connection details. 00074 if (!network) { 00075 pc.printf("\n----- Network Error -----\n"); 00076 return -1; 00077 } 00078 00079 pc.printf("\n----- Network Connected -----\n"); 00080 wait(2.0); 00081 00082 while(1) { 00083 // Set url 00084 std::stringstream ss_url; 00085 std::string s_url(URL); 00086 00087 ss_url << s_url << "?app=" << app_id << "&id=" << id; 00088 string url = ss_url.str(); 00089 pc.printf("%s\n", url.c_str()); 00090 00091 pc.printf("\n----- HTTPS GET request -----\n"); 00092 HttpsRequest* get_req = new HttpsRequest(network, SSL_CA_PEM, HTTP_GET, url.c_str()); 00093 get_req->set_header("X-Cybozu-API-Token", API_TOKEN); 00094 HttpResponse* get_res = get_req->send(); 00095 00096 pc.printf("\n----- HTTPS GET response [%d]-----\n", get_res->get_status_code()); 00097 00098 if(get_res->get_status_code() == 200) { 00099 pc.printf("\n----- HTTPS GET response 200 -----\n"); 00100 const char* body = get_res->get_body_as_string().c_str(); 00101 00102 pc.printf("%s\n", body); 00103 00104 // Response JSON parse 00105 char value[256]; 00106 00107 char* p = j_paser(body, "日付", value); 00108 j_paser(p,"value", value); 00109 pc.printf("date:%s\n", value); 00110 00111 p = j_paser(body, "文字列__1行_", value); 00112 j_paser(p,"value", value); 00113 pc.printf("%s\n", value); 00114 00115 buzzer = 1; 00116 wait(0.1); 00117 buzzer = 0; 00118 } 00119 00120 delete get_req; 00121 wait(10.0); 00122 } 00123 }
Generated on Sat Jul 16 2022 06:48:29 by
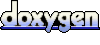