Embed:
(wiki syntax)
Show/hide line numbers
Accelerometer.cpp
00001 #include "Accelerometer.h" 00002 Accelerometer::Accelerometer(PinName xoutPin,PinName youtPin,PinName zoutPin,PinName sleepPin,PinName zeroGDetectPin,PinName gSelectPin): 00003 00004 xout(xoutPin),yout(youtPin),zout(zoutPin),zeroGDetect(zeroGDetectPin),sleep(sleepPin),gSelect(gSelectPin),zeroG(zeroGDetectPin) 00005 { 00006 sleep = 1; //normal mode 00007 gSelect = 0; //1.5G mode 00008 scale = 0.8; 00009 } 00010 00011 float Accelerometer ::getAccel(){ 00012 float x = getAccelX(); 00013 float y = getAccelY(); 00014 float z = getAccelZ(); 00015 return sqrt (x*x + y*y + z*z); 00016 00017 } 00018 00019 float Accelerometer :: getAccelX(){ 00020 return ((xout*3.3)-1.65)/scale; 00021 } 00022 00023 float Accelerometer :: getAccelY(){ 00024 return ((yout*3.3)-1.65)/scale; 00025 } 00026 00027 float Accelerometer :: getAccelZ(){ 00028 return ((zout*3.3)-1.65)/scale; 00029 } 00030 00031 float Accelerometer :: getTiltX() { 00032 00033 float x =getAccelX(); 00034 float y =getAccelY(); 00035 float z =getAccelZ(); 00036 float a =sqrt(x*x + y*y + z*z); 00037 return asin (x/a); 00038 00039 } 00040 00041 float Accelerometer :: getTiltY(){ 00042 00043 float x =getAccelX(); 00044 float y =getAccelY(); 00045 float z =getAccelZ(); 00046 float a =sqrt(x*x + y*y + z*z); 00047 return asin (y/a); 00048 00049 } 00050 00051 float Accelerometer :: getTiltZ(){ 00052 00053 float x =getAccelX(); 00054 float y =getAccelY(); 00055 float z =getAccelZ(); 00056 float a =sqrt(x*x + y*y + z*z); 00057 return asin (z/a); 00058 00059 } 00060 00061 void Accelerometer :: setScale(Scale scale){ 00062 switch (scale){ 00063 00064 case SCALE_1_5G: 00065 this->scale =0.8; 00066 gSelect = 0; 00067 break; 00068 case SCALE_6G: 00069 this->scale = 0.206; 00070 gSelect = 1; 00071 break; 00072 00073 } 00074 00075 } 00076 00077 00078 void Accelerometer ::setSleep(bool on){ 00079 sleep= !on; 00080 } 00081 00082 00083 bool Accelerometer :: detectedZeroG(){ 00084 return zeroGDetect; 00085 } 00086 00087 void Accelerometer ::setZeroGDetectListener(void(*func)(void)){ 00088 zeroG.rise(func); 00089 } 00090 00091 template<typename T> void Accelerometer ::setZeroGDetectListener(T*t,void (T::*func)(void)){ 00092 zeroG.rise(t,func); 00093 }
Generated on Sat Aug 6 2022 16:30:54 by
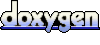