Library for controlling a strip of Adafruit NeoPixels with WS2812 drivers. Because of the strict timing requirements of the self-clocking data signal, the critical parts of code are written in ARM assembly. Currently, only the NXP LPC1768 platform is supported. More information about NeoPixels can be found at http://learn.adafruit.com/adafruit-neopixel-uberguide/overview
Dependents: NeoPixels ECE4180_Werable_LCD_DEMO neopixel_spi NeoPixels ... more
NeoStrip.h
00001 /** 00002 * NeoStrip.h 00003 * 00004 * Allen Wild 00005 * March 2014 00006 * 00007 * Library for the control of Adafruit NeoPixel addressable RGB LEDs. 00008 */ 00009 00010 00011 #ifndef NEOSTRIP_H 00012 #define NEOSTRIP_H 00013 00014 #ifndef TARGET_LPC1768 00015 #error NeoStrip only supports the NXP LPC1768! 00016 #endif 00017 00018 // NeoColor struct definition to hold 24 bit 00019 // color data for each pixel, in GRB order 00020 typedef struct _NeoColor 00021 { 00022 uint8_t green; 00023 uint8_t red; 00024 uint8_t blue; 00025 } NeoColor; 00026 00027 /** 00028 * NeoStrip objects manage the buffering and assigning of 00029 * addressable NeoPixels 00030 */ 00031 class NeoStrip 00032 { 00033 public: 00034 00035 /** 00036 * Create a NeoStrip object 00037 * 00038 * @param pin The mbed data pin name 00039 * @param N The number of pixels in the strip 00040 */ 00041 NeoStrip(PinName pin, int N); 00042 00043 /** 00044 * Set an overall brightness scale for the entire strip. 00045 * When colors are set using setPixel(), they are scaled 00046 * according to this brightness. 00047 * 00048 * Because NeoPixels are very bright and draw a lot of current, 00049 * the brightness is set to 0.5 by default. 00050 * 00051 * @param bright The brightness scale between 0 and 1.0 00052 */ 00053 void setBrightness(float bright); 00054 00055 /** 00056 * Set a single pixel to the specified color. 00057 * 00058 * This method (and all other setPixel methods) uses modulo-N arithmetic 00059 * when addressing pixels. If p >= N, the pixel address will wrap around. 00060 * 00061 * @param p The pixel number (starting at 0) to set 00062 * @param color A 24-bit color packed into a single int, 00063 * using standard hex color codes (e.g. 0xFF0000 is red) 00064 */ 00065 void setPixel(int p, int color); 00066 00067 /** 00068 * Set a single pixel to the specified color, with red, green, and blue 00069 * values in separate arguments. 00070 */ 00071 void setPixel(int p, uint8_t red, uint8_t green, uint8_t blue); 00072 00073 /** 00074 * Set n pixels starting at pixel p. 00075 * 00076 * @param p The first pixel in the strip to set. 00077 * @param n The number of pixels to set. 00078 * @param colors An array of length n containing the 24-bit colors for each pixel 00079 */ 00080 void setPixels(int p, int n, const int *colors); 00081 00082 /** 00083 * Reset all pixels in the strip to be of (0x000000) 00084 */ 00085 void clear(); 00086 00087 /** 00088 * Write the colors out to the strip; this method must be called 00089 * to see any hardware effect. 00090 * 00091 * This function disables interrupts while the strip data is being sent, 00092 * each pixel takes approximately 30us to send, plus a 50us reset pulse 00093 * at the end. 00094 */ 00095 void write(); 00096 00097 protected: 00098 NeoColor *strip; // pixel data buffer modified by setPixel() and used by neo_out() 00099 int N; // the number of pixels in the strip 00100 int Nbytes; // the number of bytes of pixel data (always N*3) 00101 float bright; // the master strip brightness 00102 gpio_t gpio; // gpio struct for initialization and getting register addresses 00103 }; 00104 00105 #endif 00106 00107
Generated on Tue Jul 12 2022 18:57:23 by
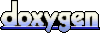