
An example program using my NeoStrip library to control an Adafruit 8x8 NeoPixel matrix (http://www.adafruit.com/products/1487)
Dependents: ECE4180_Werable_LCD_DEMO
main.cpp
00001 /* 00002 * Adafruit NeoPixel 8x8 matrix example 00003 * 00004 * This program displays a couple simple patterns on an 8x8 NeoPixel matrix. 00005 * 00006 * 3 buttons are used for DigitalIns, 2 control the brightness up and down, 00007 * and the third switches patterns 00008 */ 00009 00010 #include "mbed.h" 00011 #include "NeoStrip.h" 00012 #include "gt.h" 00013 00014 #define N 64 00015 #define PATTERNS 3 00016 00017 int hueToRGB(float h); 00018 void pattern0(); 00019 void pattern1(); 00020 void pattern2(); 00021 00022 // array of function pointers to the various patterns 00023 void (*patterns[])(void) = {&pattern0, &pattern1, &pattern2}; 00024 00025 NeoStrip strip(p18, N); 00026 DigitalIn b1(p20); // brightness up 00027 DigitalIn b2(p19); // brightness down 00028 DigitalIn b3(p21); // next pattern 00029 00030 // timer used for debugging 00031 Timer timer; 00032 00033 int main() 00034 { 00035 b1.mode(PullDown); 00036 b2.mode(PullDown); 00037 b3.mode(PullDown); 00038 00039 int pattern = 0; 00040 float bright = 0.2; // 20% is plenty for indoor use 00041 bool b3o = b3; // old copy of button 3 to poll for changes 00042 00043 strip.setBrightness(bright); // set default brightness 00044 00045 while (true) 00046 { 00047 timer.reset(); // use a timer to measure loop execution time for debugging purposes 00048 timer.start(); // for this application, the main loop takes approximately 3ms to run 00049 00050 // button 1 increases brightness 00051 if (b1 && bright < 1) 00052 { 00053 bright += 0.01; 00054 if (bright > 1) 00055 bright = 1; 00056 strip.setBrightness(bright); 00057 } 00058 00059 // button 2 decreases brightness 00060 if (b2 && bright > 0) 00061 { 00062 bright -= 0.01; 00063 if (bright < 0) 00064 bright = 0; 00065 strip.setBrightness(bright); 00066 } 00067 00068 // button 3 changes the pattern, only do stuff when its state has changed 00069 if (b3 != b3o) 00070 { 00071 if (b3 && ++pattern == PATTERNS) 00072 pattern = 0; 00073 b3o = b3; 00074 } 00075 00076 // run the pattern update function which sets the strip's pixels 00077 patterns[pattern](); 00078 strip.write(); 00079 00080 timer.stop(); 00081 // print loop time if b3 is pressed 00082 if (b3) 00083 printf("Loop Time: %dus\n", timer.read_us()); 00084 00085 wait_ms(10); 00086 } 00087 } 00088 00089 // pattern0 displays a static image 00090 void pattern0() 00091 { 00092 strip.setPixels(0, N, gt_img); 00093 } 00094 00095 // display a shifting rainbow, all colors have maximum 00096 // saturation and value, with evenly spaced hue 00097 void pattern1() 00098 { 00099 static float dh = 360.0 / N; 00100 static float x = 0; 00101 00102 for (int i = 0; i < N; i++) 00103 strip.setPixel(i, hueToRGB((dh * i) - x)); 00104 00105 x += 1; 00106 if (x > 360) 00107 x = 0; 00108 } 00109 00110 // display a shifting gradient between red and blue 00111 void pattern2() 00112 { 00113 // offset for each pixel to allow the pattern to move 00114 static float x = 0; 00115 00116 float r, b, y; 00117 00118 for (int i = 0; i < N; i++) 00119 { 00120 // y is a scaled position between 0 (red) and 1.0 (blue) 00121 y = 1.0 * i / (N - 1) + x; 00122 if (y > 1) 00123 y -= 1; 00124 00125 // if on the left half, red is decreasing and blue is increasng 00126 if (y < 0.5) 00127 { 00128 b = 2 * y; 00129 r = 1 - b; 00130 } 00131 00132 // else red is increasing and blue is decreasing 00133 else 00134 { 00135 r = 2 * (y - 0.5); 00136 b = 1 - r; 00137 } 00138 00139 // scale to integers and set the pixel 00140 strip.setPixel(i, (uint8_t)(r * 255), 0, (uint8_t)(b * 200)); 00141 } 00142 00143 x += 0.003; 00144 if (x > 1) 00145 x = 0; 00146 } 00147 00148 // Converts HSV to RGB with the given hue, assuming 00149 // maximum saturation and value 00150 int hueToRGB(float h) 00151 { 00152 // lots of floating point magic from the internet and scratching my head 00153 float r, g, b; 00154 if (h > 360) 00155 h -= 360; 00156 if (h < 0) 00157 h += 360; 00158 int i = (int)(h / 60.0); 00159 float f = (h / 60.0) - i; 00160 float q = 1 - f; 00161 00162 switch (i % 6) 00163 { 00164 case 0: r = 1; g = f; b = 0; break; 00165 case 1: r = q; g = 1; b = 0; break; 00166 case 2: r = 0; g = 1; b = f; break; 00167 case 3: r = 0; g = q; b = 1; break; 00168 case 4: r = f; g = 0; b = 1; break; 00169 case 5: r = 1; g = 0; b = q; break; 00170 default: r = 0; g = 0; b = 0; break; 00171 } 00172 00173 // scale to integers and return the packed value 00174 uint8_t R = (uint8_t)(r * 255); 00175 uint8_t G = (uint8_t)(g * 255); 00176 uint8_t B = (uint8_t)(b * 255); 00177 00178 return (R << 16) | (G << 8) | B; 00179 } 00180
Generated on Thu Jul 14 2022 04:04:31 by
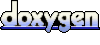