
sdf
Dependencies: mbed C12832 Servo RangeFinder Pulse
main.cpp
00001 #include "mbed.h" 00002 #include <MoistureSensor.h> 00003 #include "RangeFinder.h" 00004 #include <Motor_relay.h> 00005 #include "Servo.h" 00006 #include <C12832.h> 00007 00008 int dry = 0.61; 00009 int wet = 0.37; 00010 moisture_sensor grove(p17); 00011 RangeFinder rf(p18, 10, 5800.0, 100000); 00012 DigitalOut led(LED1); 00013 MOTOR_RELAY motor_relay1(p22); 00014 MOTOR_RELAY motor_relay2(p21); 00015 Servo valve1(p23); 00016 Servo valve2(p24); 00017 C12832 mylcd(p5,p7,p6,p8,p11); 00018 #define on 1 00019 #define off 0 00020 int main (void) 00021 { 00022 00023 float moisturevalue = 0.0f; 00024 float moisturepercentage = 0.0f; 00025 led = 1; 00026 float d; 00027 while (true) { 00028 00029 moisturevalue = grove.read(); 00030 moisturepercentage=(((moisturevalue- 0.37)/(0.61-0.37))*100); 00031 debug("Moisture reading is %2.2f percent dry\n\r",moisturepercentage); 00032 mylcd.locate(0,0); 00033 mylcd.printf("Moist: %.2f",moisturepercentage); 00034 00035 wait(1.0f); 00036 00037 d = rf.read_m(); 00038 if (d == -1.0) { 00039 printf("Timeout Error.\n\r"); 00040 } else if (d > 5.0) { 00041 // Seeed's sensor has a maximum range of 4m, it returns 00042 // something like 7m if the ultrasound pulse isn't reflected. 00043 printf("No object within detection range.\n"); 00044 } else { 00045 printf("Distance = %f m.\n", d); 00046 mylcd.locate(0,10); 00047 mylcd.printf("Distance = %f m.\n", d); 00048 } 00049 wait(0.5); 00050 led = !led; 00051 00052 00053 if(moisturepercentage>80) { 00054 mylcd.locate(0,10); 00055 mylcd.printf("outletvalve:open pump:on"); 00056 motor_relay1.SetMotor(1); 00057 valve1.position(0); 00058 } 00059 if(moisturepercentage<20) { 00060 mylcd.locate(0,10); 00061 mylcd.printf("outletvalve:close pump:off"); 00062 motor_relay1.SetMotor(0); 00063 valve1.position(90); 00064 } 00065 if(20<moisturepercentage<80) { 00066 mylcd.locate(0,10); 00067 mylcd.printf("Moisture:ok pump:off"); 00068 motor_relay1.SetMotor(0); 00069 } 00070 if (d>0.07 ) { 00071 mylcd.locate(0,20); 00072 mylcd.printf("inletvalve:open pump:on"); 00073 motor_relay2.SetMotor(1); 00074 valve2.position(90); 00075 } 00076 if(d<0.02) { 00077 mylcd.locate(0,20); 00078 mylcd.printf("inletvalve:closed pump:off"); 00079 motor_relay2.SetMotor(0); 00080 valve2.position(0); 00081 } 00082 if (0.02<d<0.07) { 00083 mylcd.locate(0,20); 00084 mylcd.printf("water level:ok pump:off"); 00085 motor_relay2.SetMotor(0); 00086 } 00087 } 00088 return 0; 00089 } 00090 00091
Generated on Wed Jul 27 2022 00:26:44 by
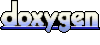