This is a port of cyaSSL 2.7.0.
Dependents: CyaSSL_DTLS_Cellular CyaSSL_DTLS_Ethernet
md2.c
00001 /* md2.c 00002 * 00003 * Copyright (C) 2006-2013 wolfSSL Inc. 00004 * 00005 * This file is part of CyaSSL. 00006 * 00007 * CyaSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * CyaSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA 00020 */ 00021 00022 00023 #ifdef HAVE_CONFIG_H 00024 #include <config.h> 00025 #endif 00026 00027 #include <cyassl/ctaocrypt/settings.h> 00028 00029 #ifdef CYASSL_MD2 00030 00031 #include <cyassl/ctaocrypt/md2.h> 00032 #ifdef NO_INLINE 00033 #include <cyassl/ctaocrypt/misc.h> 00034 #else 00035 #include <ctaocrypt/src/misc.c> 00036 #endif 00037 00038 00039 void InitMd2(Md2* md2) 00040 { 00041 XMEMSET(md2->X, 0, MD2_X_SIZE); 00042 XMEMSET(md2->C, 0, MD2_BLOCK_SIZE); 00043 XMEMSET(md2->buffer, 0, MD2_BLOCK_SIZE); 00044 md2->count = 0; 00045 } 00046 00047 00048 void Md2Update(Md2* md2, const byte* data, word32 len) 00049 { 00050 static const byte S[256] = 00051 { 00052 41, 46, 67, 201, 162, 216, 124, 1, 61, 54, 84, 161, 236, 240, 6, 00053 19, 98, 167, 5, 243, 192, 199, 115, 140, 152, 147, 43, 217, 188, 00054 76, 130, 202, 30, 155, 87, 60, 253, 212, 224, 22, 103, 66, 111, 24, 00055 138, 23, 229, 18, 190, 78, 196, 214, 218, 158, 222, 73, 160, 251, 00056 245, 142, 187, 47, 238, 122, 169, 104, 121, 145, 21, 178, 7, 63, 00057 148, 194, 16, 137, 11, 34, 95, 33, 128, 127, 93, 154, 90, 144, 50, 00058 39, 53, 62, 204, 231, 191, 247, 151, 3, 255, 25, 48, 179, 72, 165, 00059 181, 209, 215, 94, 146, 42, 172, 86, 170, 198, 79, 184, 56, 210, 00060 150, 164, 125, 182, 118, 252, 107, 226, 156, 116, 4, 241, 69, 157, 00061 112, 89, 100, 113, 135, 32, 134, 91, 207, 101, 230, 45, 168, 2, 27, 00062 96, 37, 173, 174, 176, 185, 246, 28, 70, 97, 105, 52, 64, 126, 15, 00063 85, 71, 163, 35, 221, 81, 175, 58, 195, 92, 249, 206, 186, 197, 00064 234, 38, 44, 83, 13, 110, 133, 40, 132, 9, 211, 223, 205, 244, 65, 00065 129, 77, 82, 106, 220, 55, 200, 108, 193, 171, 250, 36, 225, 123, 00066 8, 12, 189, 177, 74, 120, 136, 149, 139, 227, 99, 232, 109, 233, 00067 203, 213, 254, 59, 0, 29, 57, 242, 239, 183, 14, 102, 88, 208, 228, 00068 166, 119, 114, 248, 235, 117, 75, 10, 49, 68, 80, 180, 143, 237, 00069 31, 26, 219, 153, 141, 51, 159, 17, 131, 20 00070 }; 00071 00072 while (len) { 00073 word32 L = (MD2_PAD_SIZE - md2->count) < len ? 00074 (MD2_PAD_SIZE - md2->count) : len; 00075 XMEMCPY(md2->buffer + md2->count, data, L); 00076 md2->count += L; 00077 data += L; 00078 len -= L; 00079 00080 if (md2->count == MD2_PAD_SIZE) { 00081 int i; 00082 byte t; 00083 00084 md2->count = 0; 00085 XMEMCPY(md2->X + MD2_PAD_SIZE, md2->buffer, MD2_PAD_SIZE); 00086 t = md2->C[15]; 00087 00088 for(i = 0; i < MD2_PAD_SIZE; i++) { 00089 md2->X[32 + i] = md2->X[MD2_PAD_SIZE + i] ^ md2->X[i]; 00090 t = md2->C[i] ^= S[md2->buffer[i] ^ t]; 00091 } 00092 00093 t=0; 00094 for(i = 0; i < 18; i++) { 00095 int j; 00096 for(j = 0; j < MD2_X_SIZE; j += 8) { 00097 t = md2->X[j+0] ^= S[t]; 00098 t = md2->X[j+1] ^= S[t]; 00099 t = md2->X[j+2] ^= S[t]; 00100 t = md2->X[j+3] ^= S[t]; 00101 t = md2->X[j+4] ^= S[t]; 00102 t = md2->X[j+5] ^= S[t]; 00103 t = md2->X[j+6] ^= S[t]; 00104 t = md2->X[j+7] ^= S[t]; 00105 } 00106 t = (t + i) & 0xFF; 00107 } 00108 } 00109 } 00110 } 00111 00112 00113 void Md2Final(Md2* md2, byte* hash) 00114 { 00115 byte padding[MD2_BLOCK_SIZE]; 00116 word32 padLen = MD2_PAD_SIZE - md2->count; 00117 word32 i; 00118 00119 for (i = 0; i < padLen; i++) 00120 padding[i] = (byte)padLen; 00121 00122 Md2Update(md2, padding, padLen); 00123 Md2Update(md2, md2->C, MD2_BLOCK_SIZE); 00124 00125 XMEMCPY(hash, md2->X, MD2_DIGEST_SIZE); 00126 00127 InitMd2(md2); 00128 } 00129 00130 00131 #endif /* CYASSL_MD2 */
Generated on Tue Jul 12 2022 20:44:51 by
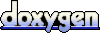