This is a port of cyaSSL 2.7.0.
Dependents: CyaSSL_DTLS_Cellular CyaSSL_DTLS_Ethernet
compress.c
00001 /* compress.c 00002 * 00003 * Copyright (C) 2006-2013 wolfSSL Inc. 00004 * 00005 * This file is part of CyaSSL. 00006 * 00007 * CyaSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * CyaSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA 00020 */ 00021 00022 00023 #ifdef HAVE_CONFIG_H 00024 #include <config.h> 00025 #endif 00026 00027 #include <cyassl/ctaocrypt/settings.h> 00028 00029 #ifdef HAVE_LIBZ 00030 00031 00032 #include <cyassl/ctaocrypt/compress.h> 00033 #include <cyassl/ctaocrypt/ctaoerror2.h> 00034 #include <cyassl/ctaocrypt/logging.h> 00035 #ifdef NO_INLINE 00036 #include <cyassl/ctaocrypt/misc.h> 00037 #else 00038 #include <ctaocrypt/src/misc.c> 00039 #endif 00040 00041 #include <zlib.h> 00042 00043 00044 /* alloc user allocs to work with zlib */ 00045 static void* myAlloc(void* opaque, unsigned int item, unsigned int size) 00046 { 00047 (void)opaque; 00048 return XMALLOC(item * size, opaque, DYNAMIC_TYPE_LIBZ); 00049 } 00050 00051 00052 static void myFree(void* opaque, void* memory) 00053 { 00054 (void)opaque; 00055 XFREE(memory, opaque, DYNAMIC_TYPE_LIBZ); 00056 } 00057 00058 00059 #ifdef HAVE_MCAPI 00060 #define DEFLATE_DEFAULT_WINDOWBITS 11 00061 #define DEFLATE_DEFAULT_MEMLEVEL 1 00062 #else 00063 #define DEFLATE_DEFAULT_WINDOWBITS 15 00064 #define DEFLATE_DEFAULT_MEMLEVEL 8 00065 #endif 00066 00067 00068 int Compress(byte* out, word32 outSz, const byte* in, word32 inSz, word32 flags) 00069 /* 00070 * out - pointer to destination buffer 00071 * outSz - size of destination buffer 00072 * in - pointer to source buffer to compress 00073 * inSz - size of source to compress 00074 * flags - flags to control how compress operates 00075 * 00076 * return: 00077 * negative - error code 00078 * positive - bytes stored in out buffer 00079 * 00080 * Note, the output buffer still needs to be larger than the input buffer. 00081 * The right chunk of data won't compress at all, and the lookup table will 00082 * add to the size of the output. The libz code says the compressed 00083 * buffer should be srcSz + 0.1% + 12. 00084 */ 00085 { 00086 z_stream stream; 00087 int result = 0; 00088 00089 stream.next_in = (Bytef*)in; 00090 stream.avail_in = (uInt)inSz; 00091 #ifdef MAXSEG_64K 00092 /* Check for source > 64K on 16-bit machine: */ 00093 if ((uLong)stream.avail_in != inSz) return COMPRESS_INIT_E; 00094 #endif 00095 stream.next_out = out; 00096 stream.avail_out = (uInt)outSz; 00097 if ((uLong)stream.avail_out != outSz) return COMPRESS_INIT_E; 00098 00099 stream.zalloc = (alloc_func)myAlloc; 00100 stream.zfree = (free_func)myFree; 00101 stream.opaque = (voidpf)0; 00102 00103 if (deflateInit2(&stream, Z_DEFAULT_COMPRESSION, Z_DEFLATED, 00104 DEFLATE_DEFAULT_WINDOWBITS, DEFLATE_DEFAULT_MEMLEVEL, 00105 flags ? Z_FIXED : Z_DEFAULT_STRATEGY) != Z_OK) 00106 return COMPRESS_INIT_E; 00107 00108 if (deflate(&stream, Z_FINISH) != Z_STREAM_END) { 00109 deflateEnd(&stream); 00110 return COMPRESS_E; 00111 } 00112 00113 result = (int)stream.total_out; 00114 00115 if (deflateEnd(&stream) != Z_OK) 00116 result = COMPRESS_E; 00117 00118 return result; 00119 } 00120 00121 00122 int DeCompress(byte* out, word32 outSz, const byte* in, word32 inSz) 00123 /* 00124 * out - pointer to destination buffer 00125 * outSz - size of destination buffer 00126 * in - pointer to source buffer to compress 00127 * inSz - size of source to compress 00128 * flags - flags to control how compress operates 00129 * 00130 * return: 00131 * negative - error code 00132 * positive - bytes stored in out buffer 00133 */ 00134 { 00135 z_stream stream; 00136 int result = 0; 00137 00138 stream.next_in = (Bytef*)in; 00139 stream.avail_in = (uInt)inSz; 00140 /* Check for source > 64K on 16-bit machine: */ 00141 if ((uLong)stream.avail_in != inSz) return DECOMPRESS_INIT_E; 00142 00143 stream.next_out = out; 00144 stream.avail_out = (uInt)outSz; 00145 if ((uLong)stream.avail_out != outSz) return DECOMPRESS_INIT_E; 00146 00147 stream.zalloc = (alloc_func)myAlloc; 00148 stream.zfree = (free_func)myFree; 00149 stream.opaque = (voidpf)0; 00150 00151 if (inflateInit2(&stream, DEFLATE_DEFAULT_WINDOWBITS) != Z_OK) 00152 return DECOMPRESS_INIT_E; 00153 00154 if (inflate(&stream, Z_FINISH) != Z_STREAM_END) { 00155 inflateEnd(&stream); 00156 return DECOMPRESS_E; 00157 } 00158 00159 result = (int)stream.total_out; 00160 00161 if (inflateEnd(&stream) != Z_OK) 00162 result = DECOMPRESS_E; 00163 00164 return result; 00165 } 00166 00167 00168 #endif /* HAVE_LIBZ */ 00169
Generated on Tue Jul 12 2022 20:44:50 by
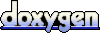