This is a port of cyaSSL 2.7.0.
Dependents: CyaSSL_DTLS_Cellular CyaSSL_DTLS_Ethernet
bn.h
00001 /* bn.h for openssl */ 00002 00003 00004 #ifndef CYASSL_BN_H_ 00005 #define CYASSL_BN_H_ 00006 00007 #include <cyassl/ctaocrypt/settings.h> 00008 00009 #ifdef __cplusplus 00010 extern "C" { 00011 #endif 00012 00013 typedef struct CYASSL_BIGNUM { 00014 int neg; /* openssh deference */ 00015 void* internal; /* our big num */ 00016 } CYASSL_BIGNUM; 00017 00018 00019 typedef struct CYASSL_BN_CTX CYASSL_BN_CTX; 00020 00021 00022 CYASSL_API CYASSL_BN_CTX* CyaSSL_BN_CTX_new(void); 00023 CYASSL_API void CyaSSL_BN_CTX_init(CYASSL_BN_CTX*); 00024 CYASSL_API void CyaSSL_BN_CTX_free(CYASSL_BN_CTX*); 00025 00026 CYASSL_API CYASSL_BIGNUM* CyaSSL_BN_new(void); 00027 CYASSL_API void CyaSSL_BN_free(CYASSL_BIGNUM*); 00028 CYASSL_API void CyaSSL_BN_clear_free(CYASSL_BIGNUM*); 00029 00030 00031 CYASSL_API int CyaSSL_BN_sub(CYASSL_BIGNUM*, const CYASSL_BIGNUM*, 00032 const CYASSL_BIGNUM*); 00033 CYASSL_API int CyaSSL_BN_mod(CYASSL_BIGNUM*, const CYASSL_BIGNUM*, 00034 const CYASSL_BIGNUM*, const CYASSL_BN_CTX*); 00035 00036 CYASSL_API const CYASSL_BIGNUM* CyaSSL_BN_value_one(void); 00037 00038 00039 CYASSL_API int CyaSSL_BN_num_bytes(const CYASSL_BIGNUM*); 00040 CYASSL_API int CyaSSL_BN_num_bits(const CYASSL_BIGNUM*); 00041 00042 CYASSL_API int CyaSSL_BN_is_zero(const CYASSL_BIGNUM*); 00043 CYASSL_API int CyaSSL_BN_is_one(const CYASSL_BIGNUM*); 00044 CYASSL_API int CyaSSL_BN_is_odd(const CYASSL_BIGNUM*); 00045 00046 CYASSL_API int CyaSSL_BN_cmp(const CYASSL_BIGNUM*, const CYASSL_BIGNUM*); 00047 00048 CYASSL_API int CyaSSL_BN_bn2bin(const CYASSL_BIGNUM*, unsigned char*); 00049 CYASSL_API CYASSL_BIGNUM* CyaSSL_BN_bin2bn(const unsigned char*, int len, 00050 CYASSL_BIGNUM* ret); 00051 00052 CYASSL_API int CyaSSL_mask_bits(CYASSL_BIGNUM*, int n); 00053 00054 CYASSL_API int CyaSSL_BN_rand(CYASSL_BIGNUM*, int bits, int top, int bottom); 00055 CYASSL_API int CyaSSL_BN_is_bit_set(const CYASSL_BIGNUM*, int n); 00056 CYASSL_API int CyaSSL_BN_hex2bn(CYASSL_BIGNUM**, const char* str); 00057 00058 CYASSL_API CYASSL_BIGNUM* CyaSSL_BN_dup(const CYASSL_BIGNUM*); 00059 CYASSL_API CYASSL_BIGNUM* CyaSSL_BN_copy(CYASSL_BIGNUM*, const CYASSL_BIGNUM*); 00060 00061 CYASSL_API int CyaSSL_BN_set_word(CYASSL_BIGNUM*, unsigned long w); 00062 00063 CYASSL_API int CyaSSL_BN_dec2bn(CYASSL_BIGNUM**, const char* str); 00064 CYASSL_API char* CyaSSL_BN_bn2dec(const CYASSL_BIGNUM*); 00065 00066 00067 typedef CYASSL_BIGNUM BIGNUM; 00068 typedef CYASSL_BN_CTX BN_CTX; 00069 00070 #define BN_CTX_new CyaSSL_BN_CTX_new 00071 #define BN_CTX_init CyaSSL_BN_CTX_init 00072 #define BN_CTX_free CyaSSL_BN_CTX_free 00073 00074 #define BN_new CyaSSL_BN_new 00075 #define BN_free CyaSSL_BN_free 00076 #define BN_clear_free CyaSSL_BN_clear_free 00077 00078 #define BN_num_bytes CyaSSL_BN_num_bytes 00079 #define BN_num_bits CyaSSL_BN_num_bits 00080 00081 #define BN_is_zero CyaSSL_BN_is_zero 00082 #define BN_is_one CyaSSL_BN_is_one 00083 #define BN_is_odd CyaSSL_BN_is_odd 00084 00085 #define BN_cmp CyaSSL_BN_cmp 00086 00087 #define BN_bn2bin CyaSSL_BN_bn2bin 00088 #define BN_bin2bn CyaSSL_BN_bin2bn 00089 00090 #define BN_mod CyaSSL_BN_mod 00091 #define BN_sub CyaSSL_BN_sub 00092 #define BN_value_one CyaSSL_BN_value_one 00093 00094 #define BN_mask_bits CyaSSL_mask_bits 00095 00096 #define BN_rand CyaSSL_BN_rand 00097 #define BN_is_bit_set CyaSSL_BN_is_bit_set 00098 #define BN_hex2bn CyaSSL_BN_hex2bn 00099 00100 #define BN_dup CyaSSL_BN_dup 00101 #define BN_copy CyaSSL_BN_copy 00102 00103 #define BN_set_word CyaSSL_BN_set_word 00104 00105 #define BN_dec2bn CyaSSL_BN_dec2bn 00106 #define BN_bn2dec CyaSSL_BN_bn2dec 00107 00108 00109 #ifdef __cplusplus 00110 } /* extern "C" */ 00111 #endif 00112 00113 00114 #endif /* CYASSL__H_ */ 00115
Generated on Tue Jul 12 2022 20:44:50 by
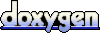