This is CoAP library with a focus on simplicity. It offers minimal CoAP PDU construction and decoding to and from byte buffers.
Dependents: cantcoap_cellular_test yeswecancoap
cantcoap.h
00001 /** 00002 Copyright (c) 2013, Ashley Mills. 00003 All rights reserved. 00004 00005 Redistribution and use in source and binary forms, with or without 00006 modification, are permitted provided that the following conditions are met: 00007 00008 1. Redistributions of source code must retain the above copyright notice, this 00009 list of conditions and the following disclaimer. 00010 2. Redistributions in binary form must reproduce the above copyright notice, 00011 this list of conditions and the following disclaimer in the documentation 00012 and/or other materials provided with the distribution. 00013 00014 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00015 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00016 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00017 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR 00018 ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00019 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00020 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00021 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00022 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00023 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00024 */ 00025 00026 #pragma once 00027 00028 #include <stdint.h> 00029 00030 #define COAP_HDR_SIZE 4 00031 #define COAP_OPTION_HDR_BYTE 1 00032 00033 // CoAP PDU format 00034 00035 // 0 1 2 3 00036 // 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 0 1 00037 // +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 00038 // |Ver| T | TKL | Code | Message ID | 00039 // +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 00040 // | Token (if any, TKL bytes) ... 00041 // +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 00042 // | Options (if any) ... 00043 // +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 00044 // |1 1 1 1 1 1 1 1| Payload (if any) ... 00045 // +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 00046 00047 class CoapPDU { 00048 00049 00050 public: 00051 /// CoAP message types. Note, values only work as enum. 00052 enum Type { 00053 COAP_CONFIRMABLE=0x00, 00054 COAP_NON_CONFIRMABLE=0x10, 00055 COAP_ACKNOWLEDGEMENT=0x20, 00056 COAP_RESET=0x30 00057 }; 00058 00059 // CoAP response codes. 00060 enum Code { 00061 COAP_EMPTY=0x00, 00062 COAP_GET, 00063 COAP_POST, 00064 COAP_PUT, 00065 COAP_DELETE, 00066 COAP_CREATED=0x41, 00067 COAP_DELETED, 00068 COAP_VALID, 00069 COAP_CHANGED, 00070 COAP_CONTENT, 00071 COAP_BAD_REQUEST=0x80, 00072 COAP_UNAUTHORIZED, 00073 COAP_BAD_OPTION, 00074 COAP_FORBIDDEN, 00075 COAP_NOT_FOUND, 00076 COAP_METHOD_NOT_ALLOWED, 00077 COAP_NOT_ACCEPTABLE, 00078 COAP_PRECONDITION_FAILED=0x8C, 00079 COAP_REQUEST_ENTITY_TOO_LARGE=0x8D, 00080 COAP_UNSUPPORTED_CONTENT_FORMAT=0x8F, 00081 COAP_INTERNAL_SERVER_ERROR=0xA0, 00082 COAP_NOT_IMPLEMENTED, 00083 COAP_BAD_GATEWAY, 00084 COAP_SERVICE_UNAVAILABLE, 00085 COAP_GATEWAY_TIMEOUT, 00086 COAP_PROXYING_NOT_SUPPORTED, 00087 COAP_UNDEFINED_CODE=0xFF 00088 }; 00089 00090 /// CoAP option numbers. 00091 enum Option { 00092 COAP_OPTION_IF_MATCH=1, 00093 COAP_OPTION_URI_HOST=3, 00094 COAP_OPTION_ETAG, 00095 COAP_OPTION_IF_NONE_MATCH, 00096 COAP_OPTION_OBSERVE, 00097 COAP_OPTION_URI_PORT, 00098 COAP_OPTION_LOCATION_PATH, 00099 COAP_OPTION_URI_PATH=11, 00100 COAP_OPTION_CONTENT_FORMAT, 00101 COAP_OPTION_MAX_AGE=14, 00102 COAP_OPTION_URI_QUERY, 00103 COAP_OPTION_ACCEPT=17, 00104 COAP_OPTION_LOCATION_QUERY=20, 00105 COAP_OPTION_BLOCK2=23, 00106 COAP_OPTION_BLOCK1=27, 00107 COAP_OPTION_SIZE2, 00108 COAP_OPTION_PROXY_URI=35, 00109 COAP_OPTION_PROXY_SCHEME=39, 00110 COAP_OPTION_SIZE1=60 00111 }; 00112 00113 /// CoAP content-formats. 00114 enum ContentFormat { 00115 COAP_CONTENT_FORMAT_TEXT_PLAIN = 0, 00116 COAP_CONTENT_FORMAT_APP_LINK = 40, 00117 COAP_CONTENT_FORMAT_APP_XML, 00118 COAP_CONTENT_FORMAT_APP_OCTET, 00119 COAP_CONTENT_FORMAT_APP_EXI = 47, 00120 COAP_CONTENT_FORMAT_APP_JSON = 50 00121 }; 00122 00123 /// Sequence of these is returned by CoapPDU::getOptions() 00124 struct CoapOption { 00125 uint16_t optionDelta; 00126 uint16_t optionNumber; 00127 uint16_t optionValueLength; 00128 int totalLength; 00129 uint8_t *optionPointer; 00130 uint8_t *optionValuePointer; 00131 }; 00132 00133 // construction and destruction 00134 CoapPDU(); 00135 CoapPDU(uint8_t *pdu, int pduLength); 00136 CoapPDU(uint8_t *buffer, int bufferLength, int pduLength); 00137 ~CoapPDU(); 00138 int reset(); 00139 int validate(); 00140 00141 // version 00142 int setVersion(uint8_t version); 00143 uint8_t getVersion(); 00144 00145 // message type 00146 void setType(CoapPDU::Type type); 00147 CoapPDU::Type getType(); 00148 00149 // tokens 00150 int setTokenLength(uint8_t tokenLength); 00151 int getTokenLength(); 00152 uint8_t* getTokenPointer(); 00153 int setToken(uint8_t *token, uint8_t tokenLength); 00154 00155 // message code 00156 void setCode(CoapPDU::Code code); 00157 CoapPDU::Code getCode(); 00158 CoapPDU::Code httpStatusToCode(int httpStatus); 00159 00160 // message ID 00161 int setMessageID(uint16_t messageID); 00162 uint16_t getMessageID(); 00163 00164 // options 00165 int addOption(uint16_t optionNumber, uint16_t optionLength, uint8_t *optionValue); 00166 // gets a list of all options 00167 CoapOption* getOptions(); 00168 int getNumOptions(); 00169 // shorthand helpers 00170 int setURI(char *uri); 00171 int setURI(char *uri, int urilen); 00172 int getURI(char *dst, int dstlen, int *outLen); 00173 int addURIQuery(char *query); 00174 00175 // content format helper 00176 int setContentFormat(CoapPDU::ContentFormat format); 00177 00178 // payload 00179 uint8_t* mallocPayload(int bytes); 00180 int setPayload(uint8_t *value, int len); 00181 uint8_t* getPayloadPointer(); 00182 int getPayloadLength(); 00183 uint8_t* getPayloadCopy(); 00184 00185 // pdu 00186 int getPDULength(); 00187 uint8_t* getPDUPointer(); 00188 void setPDULength(int len); 00189 00190 // debugging 00191 static void printBinary(uint8_t b); 00192 void print(); 00193 void printBin(); 00194 void printHex(); 00195 void printOptionHuman(uint8_t *option); 00196 void printHuman(); 00197 void printPDUAsCArray(); 00198 00199 private: 00200 // variables 00201 uint8_t *_pdu; 00202 int _pduLength; 00203 00204 int _constructedFromBuffer; 00205 int _bufferLength; 00206 00207 uint8_t *_payloadPointer; 00208 int _payloadLength; 00209 00210 int _numOptions; 00211 uint16_t _maxAddedOptionNumber; 00212 00213 // functions 00214 void shiftPDUUp(int shiftOffset, int shiftAmount); 00215 void shiftPDUDown(int startLocation, int shiftOffset, int shiftAmount); 00216 uint8_t codeToValue(CoapPDU::Code c); 00217 00218 // option stuff 00219 int findInsertionPosition(uint16_t optionNumber, uint16_t *prevOptionNumber); 00220 int computeExtraBytes(uint16_t n); 00221 int insertOption(int insertionPosition, uint16_t optionDelta, uint16_t optionValueLength, uint8_t *optionValue); 00222 uint16_t getOptionDelta(uint8_t *option); 00223 void setOptionDelta(int optionPosition, uint16_t optionDelta); 00224 uint16_t getOptionValueLength(uint8_t *option); 00225 00226 }; 00227 00228 /* 00229 #define COAP_CODE_EMPTY 0x00 00230 00231 // method codes 0.01-0.31 00232 #define COAP_CODE_GET 0x01 00233 #define COAP_CODE_POST 0x02 00234 #define COAP_CODE_PUT 0x03 00235 #define COAP_CODE_DELETE 0x04 00236 00237 // Response codes 2.00 - 5.31 00238 // 2.00 - 2.05 00239 #define COAP_CODE_CREATED 0x41 00240 #define COAP_CODE_DELETED 0x42 00241 #define COAP_CODE_VALID 0x43 00242 #define COAP_CODE_CHANGED 0x44 00243 #define COAP_CODE_CONTENT 0x45 00244 00245 // 4.00 - 4.15 00246 #define COAP_CODE_BAD_REQUEST 0x80 00247 #define COAP_CODE_UNAUTHORIZED 0x81 00248 #define COAP_CODE_BAD_OPTION 0x82 00249 #define COAP_CODE_FORBIDDEN 0x83 00250 #define COAP_CODE_NOT_FOUND 0x84 00251 #define COAP_CODE_METHOD_NOT_ALLOWED 0x85 00252 #define COAP_CODE_NOT_ACCEPTABLE 0x86 00253 #define COAP_CODE_PRECONDITION_FAILED 0x8C 00254 #define COAP_CODE_REQUEST_ENTITY_TOO_LARGE 0x8D 00255 #define COAP_CODE_UNSUPPORTED_CONTENT_FORMAT 0x8F 00256 00257 // 5.00 - 5.05 00258 #define COAP_CODE_INTERNAL_SERVER_ERROR 0xA0 00259 #define COAP_CODE_NOT_IMPLEMENTED 0xA1 00260 #define COAP_CODE_BAD_GATEWAY 0xA2 00261 #define COAP_CODE_SERVICE_UNAVAILABLE 0xA3 00262 #define COAP_CODE_GATEWAY_TIMEOUT 0xA4 00263 #define COAP_CODE_PROXYING_NOT_SUPPORTED 0xA5 00264 */
Generated on Tue Jul 12 2022 21:53:28 by
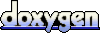